Closed jmwielandt closed 3 years ago
Hi,
These error messages are coming from serde
. It's failing to deserialize the response from the database to the type you are expecting, String
. If you look at RethinkDB API docs for the official drivers you will see that both db_create
and table_create
return an object
not a string.
Whenever you are not sure of the database response type or you don't care, you should use serde_json::Value
instead. Also if you enable logging you will see what the database is returning before the driver tries to deserialize it. See https://github.com/rethinkdb/rethinkdb-rs/blob/5eac333bdb54d451f9964486d4361fdc71056f68/examples/reql/changes.rs for an example that showcases both.
Hi, I'm learning how to use RethinkDB and I ran into a weird error.
Code:
The output:
And the weird part: the database and table are being created: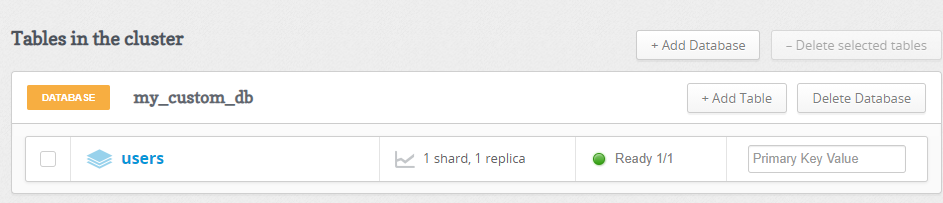
Am I doing something wrong? Thanks!