Closed ccmoralesj closed 2 years ago
Canvas render does not support shader effect.
Please change type: Phaser.CANVAS
to type: Phaser.WEBGL
Hey @rexrainbow, thanks for the quick response. I changed the type to Phaser.WEBGL
and now my config looks like this.
const config: Phaser.Types.Core.GameConfig = {
type: Phaser.WEBGL,
pixelArt: true,
fps: {
target: 1,
},
backgroundColor: GAME_BG_COLOR,
scale: {
width: 1920,
height: 1080,
mode: Phaser.Scale.FIT,
autoCenter: Phaser.Scale.CENTER_BOTH,
},
plugins: {
scene: [
{
key: 'rexUI',
plugin: RexUIPlugin,
mapping: 'rexUI',
},
{
key: 'rexGlowFilterPipeline',
plugin: GlowFilterPipelinePlugin,
mapping: 'rexGlow',
start: true,
},
],
},
};
But I'm still getting the error:
Uncaught TypeError: Cannot read properties of undefined (reading 'resetFromJSON')
🤔
GlowFilterPipelinePlugin is a global plugin, not a scene plugin. Here is a test code of GlowFilterPipelinePlugin .
It worked! I just changed it to glabl and with the WEBGL type and now I can use it pretty easily from every scene using the extends from my base class like this.rexGlow.add(gameObject, config)
I think that WEBGL type issue is something worth to have in the documentation.
Thanks a lot @rexrainbow
Great response. Just what I needed ❤️
Will add more description to notify user about that. Thanks for suggestion.
Hey there 👋🏻
I've been trying to use
GlowFilterPipelinePlugin
in my Phaser app. I've tried a lot of setups, according to the official documentation and I managed to get to a good point but I'm getting a weird error. I implementedrexUI
and is working like a charm, but I don't have the same luck withGlowFilterPipelinePlugin
.I've searched everywhere for a solution but I've only found the same issue here with no solution.
I'll get into detail here trying to give all the info and all the implementations I have tried. Any help will be appreciated A LOT.
thanks!
My Setup (as SCENE plugin)
Following the examples in the official documentation, I have gotten to this point implementing everything as a scene plugin.
Base class
I have a class that I use to extend all my Scenes
main.ts
Class where I implement the base class
The Error
The error I get is this one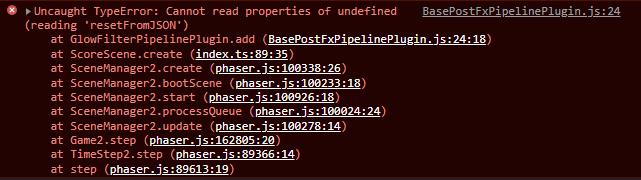
Once I get into the error, it seems the class doesn't have the
PostFxPipelineClass
(see image below) but that's not quite true because once Iconsole.log({ rexGlow: this.rexGlow })
thePostFxPipelineClass
is there.My Setup (as GLOBAL plugin)
Following the examples in the official documentation, I have gotten to this point by implementing everything as a global plugin.
main.ts
Class where I implement the base class
The Error
the error I get is this one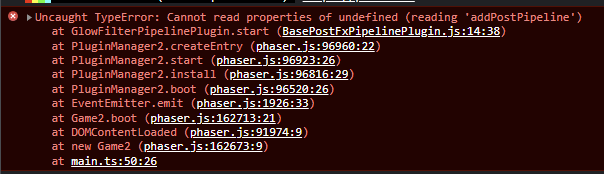
Once I get into the error I get this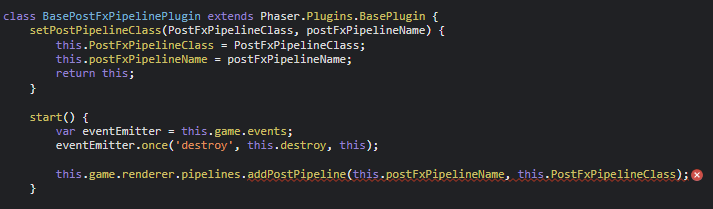