Open nunoguedelha opened 6 years ago
CC @traversaro @DanielePucci
@prashanthr05, in case the motor velocity measurement is not provided by the hardware, you should stub the respective method getMotorEncoderSpeeds in the remapper, with a code that estimates the velocity from other measurements (also considering the gearbox ratio).
I am not sure about this. If no low-level speed estimates are available, the proper replacement is a non-causal velocity estimation, that cannot be done in realtime without delay that could affect the estimation.
@traversaro , yes indeed. Sorry for the confusion, I was focusing on the interface for getting the velocity. The idea would be to have a stub that gets the last results from a velocity estimator. That estimator could eventually even be implemented in C++ and running under a different thread.
Perhaps we need to discuss this f2f, but what I meant is that for estimation we should use a non-causal velocity estimation, that could be implemented in matlab.
ok, I get it. Anyway we can discuss further after lunch.
I am not sure about this. If no low-level speed estimates are available, the proper replacement is a non-causal velocity estimation, that cannot be done in realtime without delay that could affect the estimation.
@traversaro To have clarity, in our case (if I'm not wrong), the joint velocities are obtained as a result of the velocity estimator, however we do not have a measure or an estimate for the motor velocities. But this could be obtained by mapping the joint velocities through the transmission. But, it would be better to not rely on these velocity estimates due to the inherent delay involved in the controller.
Hence we would rely on offline non-causal estimation of the velocities and accelerations by applying a savitzy-golay filtering over the joint positions. Please correct me, if I'm wrong ?
ok, I get it. Anyway we can discuss further after lunch.
I'm sorry, I'm not in IIT. I could join you through skype, if you're going to have a meeting/discussion on this.
Before using current control, I require to do current control calibration similarly to LowlevTauCtrlCalibrator
, and I am going to implement a LowlevCurrCtrlCalibrator
task (to compute PWM-current constant and BEMF friction constant).
In order to do that I need to record current measurement that is currently not available in sensor-calib-intertial
.
I have some questions regarding this implementation. @nunoguedelha
The current, as the other quantities, is written on the StateExt
port. However it is not parsed in readStateExt.m, and the getCurrents
method is not defined in the sensor-calib-intertial
ControlBoardRemapper.
I think I can work on adding current measurement, but I am probably missing some passages of the sensors measurement process. It would help if you could give me some extra info on sensors measurement framework in sensor-calib-intertial
?
When a profile is defined, clearly the option ['meas' 'joint']
enable the measurement of qs, dqs, d2qs
(joint position, velocity and acceleration) while ['meas' 'jtorq']
enables the measurement of taus
(torques). I noticed there is no specific option for dqMs
(motor velocity) and pwms
, that anyway seems to be enabled by the joints measurement option ['meas' 'joint']
.
Did I get it right?
Considering that I want to measure the current, does it need to be enabled by a different option ['meas' 'curr'], or should it be included in ['meas' 'joint']
option?
"It would help if you could give me some extra info on sensors measurement framework in sensor-calib-intertial?"
I forgot to mention in the initial notes... The compomemt SensorDataYarpI
(snapshot on the left) handles the ports required for acquiring data from specific sensors. This is defined jointly by the config file https://github.com/robotology-playground/sensors-calib-inertial/blob/master/src/conf/yarpPortNameRules.m and the method buildSensType2portNgetter(...)
:
https://github.com/robotology-playground/sensors-calib-inertial/blob/dacb506e6dc92bde46eacf6211a78a517d3cca95/src/%40SensorDataYarpI/SensorDataYarpI.m#L123-L147
This function maps the sensor types to the Yarp ports where the respective data can be retrieved. The entries to that map are the sensor types you find in the Motion Sequence Profile files like https://github.com/robotology-playground/sensors-calib-inertial/blob/master/src/conf/advanced/lowLevTauCtrlCalibratorSequenceProfile1.m. This way ['joint' , 'from']
will map to the /icub/<part>/stateExt:o
port.
The initial idea was to have an interface of the profile files independent w.r.t. how the ports group the sensor data on iCub, so although there are two labels 'joint' (for joint encoders) and 'jtorq', they both map to the same port on iCub. For some reason I forgot (probably lack of time), I didn't define a label for 'pwm' ad 'mot', but just reused 'joint'. So the option {'meas','joint'}
is the one used.
For that to work, in the stateExt:o
port, we parse all the available measurements joint q, dq, d2q, motor dq, and pwm.
Considering that I want to measure the current, does it need to be enabled by a different option ['meas' 'curr'], or should it be included in ['meas' 'joint'] option?
As you like, I suggest you use ['meas' 'curr'] and on the go, correct the case for 'pwm' and 'mot' (motor q,dq,d2q).
readStateExt.m
I let you take a look at the script and we can re-discuss on this tomorrow:
src/utils/yarp/readStateExt.m:function [q, dq, d2q, dqM, tau, pwm, time] = readStateExt(n, filename)
I am trying to run the sensor-calib-inertial
(master
branch)but at runtime I get the following error in MATLAB:
Error using yarpMEX
Invalid default value for property 'ctrlModeVocabDef' in class 'RemoteControlBoardRemapper':
Invalid default value for property 'aVocab' in class 'y':
function VOCAB requires at least 4 arguments
The problem may be related to the compilation of the bindings, and doesn't depend specifically on the ctrlModeVocabDef
property since I got the same error with pidTypeVocabDef
if it is moved before ctrlModeVocabDef
.
I am currently at:
yarp-matlab-bindings
: bindings/regenerateyarp
8c421d66b57d767f7385a56fe0b7611c8c39f975I don't get any problem during the compilation.
@nunoguedelha
@lrapetti , could you move this to a new issue please? in order to leave in this one only the tutorial discussion.
@lrapetti, @prashanthr05, I will depict here the link between the blocks in the framework design diagram below and the code entry points: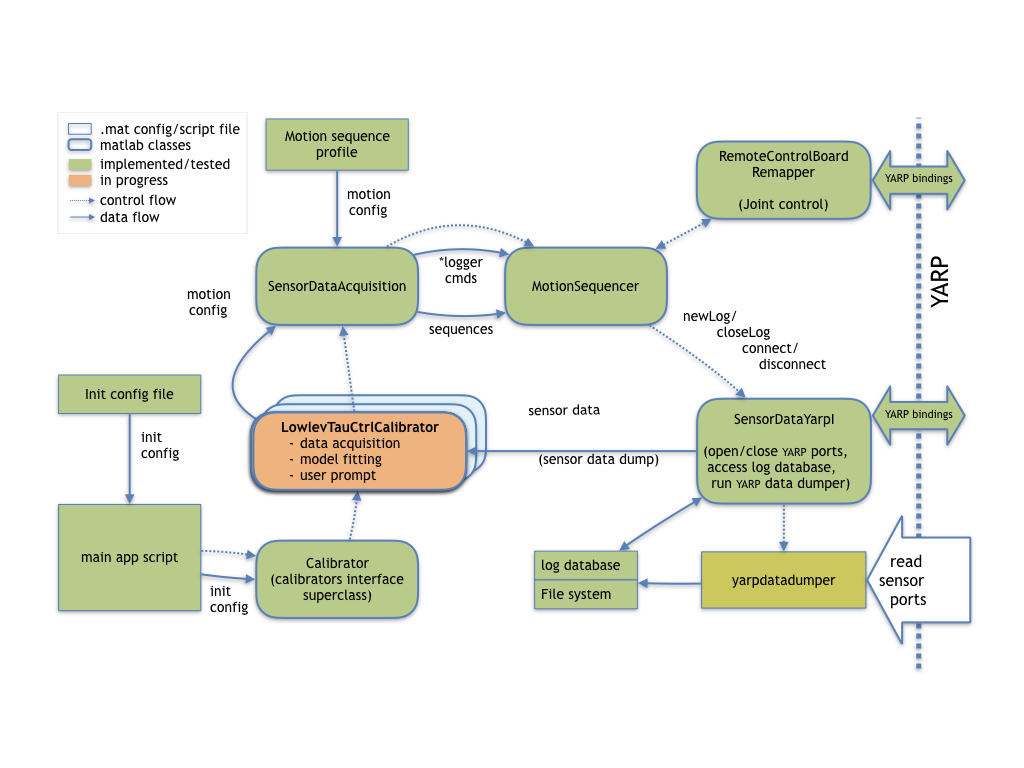
General architecture
All the framework is designed following an Object Oriented approach:
Init configuration files
Init config file
: src/conf/sensorSelfCalibratorInit.m is the main user interface. The script defines:modelName
andmodelPath
dataPath
where all the logs will be savedcalibrationMapFile
. All the paths here are relative, starting from the application folder<repo_ROOT_path>/src/app
calibrateLowLevTauCtrl = true;
and the other entries tofalse
calibedParts = {'right_leg'[,'left_leg','right_arm','left_arm']}
selects the parts to calibrate;calibedJointsIdxes
fine selects the joints for each active part (only the lines relative to selected parts are relevant);sensorDataAcq
value triggers for each estimation procedure, a new acquisition, the last acquired data, or a specific data log ID. The two later features haven't been tested for the friction/motor parameters estimation. Refer to the comments for more details.Changes for motor current control: you need an additional entry in the task checklist, and the respective block with the parameters
calibedParts
,calibedJointsIdxes
andsensorDataAcq
.Motion Sequence Profiles
:Each of these define the target joint positions sequences to execute while acquiring the desired data, along with the list of sensors to get the data from. The profile1 defines the sequences for the friction parameters estimation, while profile2 targets the motor parameters estimation. Each structure defining the joints position sequence has two fields:
labels
(the headers) andval
(the values). There are two types of structures:homeCalib
Each column defines a task (motion control, sensor data acquisition, control mode switch, ...), and each line in the
val
field section defines a time step in the sequence. Most often, each column header (for instance{'ctrl','pos','left_arm'}'
) defines respectively: an action, an action parameter, a target part.NA
stands for Not Applicable and results in no action. Let's describe each column in this example over the two time steps. First step: https://github.com/robotology-playground/sensors-calib-inertial/blob/dacb506e6dc92bde46eacf6211a78a517d3cca95/src/conf/advanced/lowLevTauCtrlCalibratorSequenceProfile1.m#L40mode
) to position control mode (keywordctrl
)ctrl
, positionpos
relative to theleft_arm
is set tohomeCalib.val{1}
, velocityvel
is set torepmat( 4,[1 7])
=> go to position that position (vector for the left arm 7 DoFs) with maximum velocity 4 degrees/smeas
from joint encodersjoint
and joint torquesjtorq
on theleft_arm
are set tofalse
=> this step is just for positioning the arm column 7: irrelevant.Second step: https://github.com/robotology-playground/sensors-calib-inertial/blob/dacb506e6dc92bde46eacf6211a78a517d3cca95/src/conf/advanced/lowLevTauCtrlCalibratorSequenceProfile1.m#L41
promptStr
to the usermode
) to PWM control mode (keywordpwmctrl
)meas
from joint encodersjoint
and joint torquesjtorq
on theleft_arm
('true')pwmctrl
), set the PWM (pwm
) parameter value to 0 for the motor namedmotor
(before this script is run, 'motor' is set to the joints/motors group being calibrated).Changes for motor current control: replace the
pwmctrl
mode by a new modecurrctrl
, and the parameterpwm
by a new parametercurr
. These parameters are processed in theMotionSequencer
class methods. Refer to the next section for more details.Yarp and robot interface:
robots-configuration/hardware/mechanicals
) that cannot be properly retrieved from the robot interface yet:fullscalePWM
,Gearbox_M2J
(torque gearbox ratio from motor to joint),matrixM2J
(motor to joint coupling matrix). TheGearbox_M2J
is used here: https://github.com/robotology-playground/sensors-calib-inertial/blob/dacb506e6dc92bde46eacf6211a78a517d3cca95/src/%40LowlevTauCtrlCalibrator/calibrateSensors.m#L90The Yarp port naming rules and the hardware transmission parameters are already defined for iCub and don't require any change except if you're using another robot. The current information should already be logged in the
stateExt
port.Main app, calibrator and motion sequencer classes
LowlevTauCtrlCalibrator
is the main class driving the friction and motor parameters estimation procedures.MotionSequencer
class triggers the ports open/close commands ('run' method) and processes the motor control parameters (run
andseqMap2runner
methods) extracted from the sequences defined in the sequence profiles mentioned in the previous section.Changes for motor current control: add the new control mode
currctrl
handling. The existing modepwmctrl
is handled in the below code sections: https://github.com/robotology-playground/sensors-calib-inertial/blob/dacb506e6dc92bde46eacf6211a78a517d3cca95/src/%40MotionSequencer/seqMap2runner.m#L76-L82(A similar section should be added for the current control mode
currctrl
)https://github.com/robotology-playground/sensors-calib-inertial/blob/dacb506e6dc92bde46eacf6211a78a517d3cca95/src/%40MotionSequencer/run.m#L82-L110 (A similar section should be added for the current control mode
currctrl
, replacing theMotorPWMcontroller
by a new current controller).Motor control interface
The class
RemoteControlBoardRemapper
wraps the bindings for creating the remote control board remapper device and get the motor interface for changing the control mode or setting the PWM (or the same way, the current).setMotorsPWM(...)
is a good example on how to get an interface likeIPWMControl
to set desired motor parameters: https://github.com/robotology-playground/sensors-calib-inertial/blob/dacb506e6dc92bde46eacf6211a78a517d3cca95/src/%40RemoteControlBoardRemapper/setMotorsPWM.m#L9Changes for motor current control: A similar interface should be created for the current control.
Note on the motor velocity measurement
@prashanthr05, in case the motor velocity measurement is not provided by the hardware, you should stub the respective method
getMotorEncoderSpeeds
in the remapper, with a code that estimates the velocity from other measurements (also considering the gearbox ratio).