Open caramot opened 2 years ago
Hey thank you very much for this :) Is it maybe possible to add on/off? I also found a Socket thermostat for my infrared heater: https://smile.amazon.de/-/en/gp/product/B09169PPD9/ref=ask_ql_qh_dp_hza Do you think this one is going to work?
hello wolfsWelpe please select at the first for parameter ID , the parameter ON/Off on your thermical module. and for you, it's not necessary to put value on parameter "set heat/cold modes" please inform me in case problem , i add comment on the code for the first ID
Okay thanks I will try as soon as I receive the thermostat :)
Thanks for this, someone might learn from my slight mistake copy/pasting in the code into the translation/traduction (French?) files entry where I didn't put a comma on the end of the existing values so when I copy/pasted in the new code it was missing a comma half way through.
Anyway having fixed that I've got it so that it now detects my air con unit but I'm struggling with the very last part setting up the entity by re-running the LocalTuya integration install. Is there a way to work out what attributes are associated with which HVAC setting? I've worked out the current temperature and I thought I'd found the target temperature but it's an order of magnitude out (180c rather than 18c). I have the same device installed via the Tuya cloud app and obviously have access to it via Tuya IOT so I was wondering if there was a way to work them all out from either of those sources?
hello, to manage the configuration of your device , the best way are to create a screen shoot of ID value , change parameter on your device , and compare delta . i don't have best way , sorry ps i add comment for problem of comma in the code , thank you
I'm using an Eurom heater - mapping of fields to values is all over the place. One of the boolean switches seems to reset or crash the device... I can't seem to get this to work in any useable form. Some device details:
{
"result": {
"category": "qn",
"functions": [
{
"code": "temp_set",
"lang_config": {
"unit": "℃"
},
"name": "Set Temp",
"type": "Integer",
"values": "{"unit":"℃","min":0,"max":37,"scale":0,"step":1}"
},
{
"code": "switch",
"lang_config": {
"false": "OFF",
"true": "ON"
},
"name": "Power",
"type": "Boolean",
"values": "{}"
}
],
"status": [
{
"code": "temp_current",
"lang_config": {
"unit": "℃"
},
"name": "Current Temp",
"type": "Integer",
"values": "{"unit":"℃","min":-9,"max":99,"scale":0,"step":1}"
},
{
"code": "temp_set",
"lang_config": {
"unit": "℃"
},
"name": "Set Temp",
"type": "Integer",
"values": "{"unit":"℃","min":0,"max":37,"scale":0,"step":1}"
},
{
"code": "switch",
"lang_config": {
"false": "OFF",
"true": "ON"
},
"name": "Power",
"type": "Boolean",
"values": "{}"
}
]
},
"success": true,
"t": 1641473662033
}
Hello, could you try , to remove /10
on this line self._current_temperature = self.dps_conf(CONF_CLIMATE_CURRENT)/10
i think to removed your problem
Hello, help with Hysen HY08-1 Tuya Thermostat. I got most of DPs sorted out, but nothing works, when I set it up via Entity Configuration. Can I set this up using configuration.yaml? Create a climate entity and point to ids?
ID | REPRESENTS | VALUES -- | -- | -- ID1 | ON / OFF | True, Flase ID2 | TARGET TEMP (x10) | 210 ID3 | CURRENT TEMP (x10) | 185 ID4 | MODE | Manual, Program, Holiday ID6 | CHILD LOCK | True, Flase ID12 | ? | 0 ID101 | ? | True, Flase ID102 | HVAC CURRENT ACTION | True, Flase ID103 | ? | 0 ID104 | HOLIDAY MODE (DAY) | int 1 to 30 ID105 | HOLIDAY MODE (TEMP) | int 10 to 30 ID106 | ? | True, Flase ID107 | ? | True, Flase ID108 | ? | True, Flase ID109 | TEMP CALIBRATION (x10) | -20 ID110 | INT SENSOR DEADZONE (x10) | 5 ID111 | EXT SENSOR DEADZONE | 2 ID112 | HIGH TEMP PROTECTION | 45 ID113 | LOW TEMP PROTECTION | 6 ID114 | MAX TEMP LIMIT | 30 ID115 | MIN TEMP LIMIT | 10 ID116 | TEMPERATURE SENSOR | in, ext, all ID117 | DEVICE STATE ON POWER | Off, keep, On ID118 | PROGRAM TYPE | 2days, 1days, 0dayshello, i don't have simple solution for solve unit for target temp, x1 or x10 the official climate module have this option sorry
Hello,
please see below the code to add new addins climate on tuyalocal
on init.py file: line 31
on new file climate.py
on const.py file add
update to:
on traduction files § data (2 area) info , please put the symbol , at the end on your last line exemple : previews "textbody": "Textbody" post "textbody": "Textbody" , and text below
on pytuya.init.py line 502 , update text to
please advice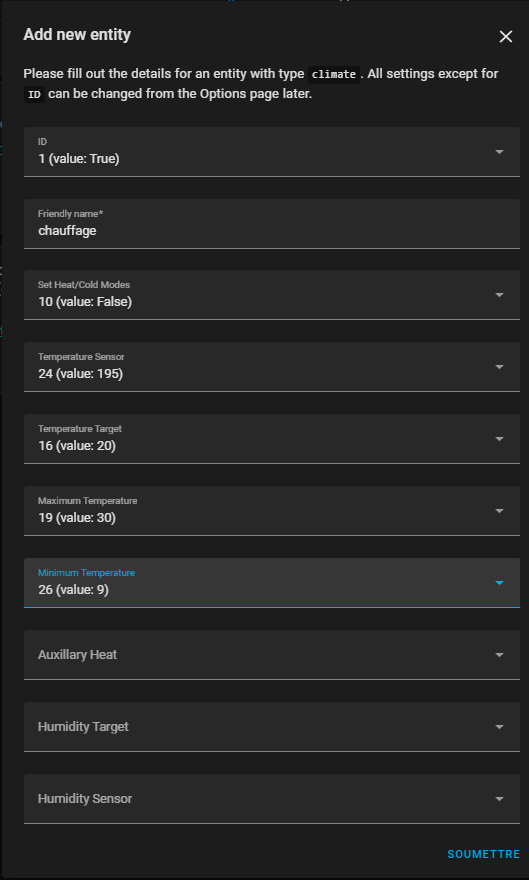