Open tobimichigan opened 5 years ago
Hi @tobimichigan ! What Node.js version do you use? Try to use Node.js 6
I have the same problem to):
seems to bee the problem with this line //const gcs = require('@google-cloud/storage')(); comment this line and deploy the functions >but only the like and the comment fun will work
@tobimichigan
@Batishev-Rozdoum what you say about that ?
@Batishev-Rozdoum , I dont think this is a node version issue. You should be aware that google is often updating their cloud functionalities. I am still debugging your repo and will give feedback shortly. @sourimoto I don't think your solution is practicable cos of runtime bugs.
@tobimichigan i don't have any runtime error, but I think the problem set in the billing
i am also facing this issue . did any one solve this ?
i am also facing problem @Batishev-Rozdoum @Batishev-Rozdoum can u tell us the how to use this without deploying it in your way
i mean to say is their any other method
@Shyamguptaa i have successfully uploaded cloud functions except generateThumnail by modifying index.js file.
Google sign in method didn't work on Android 8.0 (API level 26 or later), for Android 5.1 (API level 22) it's working fine. Any help?
@Shyamguptaa i have successfully uploaded cloud functions except generateThumnail by modifying index.js file.
please upload updated Index.js file
Hi guys! I think I've found the problem. Existing index.js file contains functions in JavaScript, not in TypeScript. Maybe during Functions Setup you choose TypeScript? In this case you can received this type of error.
Just in case, here is my parameters for working functions: Node version v8.9.1 Python 2.7.12
? What language would you like to use to write Cloud Functions? JavaScript ? Do you want to use ESLint to catch probable bugs and enforce style? Yes ? File functions/package.json already exists. Overwrite? No i Skipping write of functions/package.json ✔ Wrote functions/.eslintrc.json ? File functions/index.js already exists. Overwrite? No i Skipping write of functions/index.js ✔ Wrote functions/.gitignore ? Do you want to install dependencies with npm now? Yes
Hope it helps
@Shyamguptaa i have successfully uploaded cloud functions except generateThumnail by modifying index.js file.
please upload updated Index.js file
just remove the thumbnails function and rest of functions will work.
I Solved by const gcs = require('@google-cloud/storage'); (without () at the end of each statement)
Hi guys! I think I've found the problem. Existing index.js file contains functions in JavaScript, not in TypeScript. Maybe during Functions Setup you choose TypeScript? In this case you can received this type of error.
Just in case, here is my parameters for working functions: Node version v8.9.1 Python 2.7.12
? What language would you like to use to write Cloud Functions? JavaScript ? Do you want to use ESLint to catch probable bugs and enforce style? Yes ? File functions/package.json already exists. Overwrite? No i Skipping write of functions/package.json ✔ Wrote functions/.eslintrc.json ? File functions/index.js already exists. Overwrite? No i Skipping write of functions/index.js ✔ Wrote functions/.gitignore ? Do you want to install dependencies with npm now? Yes
Hope it helps
no that's not the problem
Hi there developers,
I recently started working on this repo and set it up successfully but during the cloud function deployment, issues sprung up:
Original from repo index.js `const functions = require('firebase-functions');
const admin = require('firebase-admin'); admin.initializeApp(functions.config().firebase);
const actionTypeNewLike = "new_like"; const actionTypeNewComment = "new_comment"; const actionTypeNewPost = "new_post"; const notificationTitle = "Social App";
const followingPosDbValue = "following_post"; const followingPosDbKey = "followingPostsIds"; const followingsDbKey = "followings"; const followersDbKey = "followers"; const followingDbKey = "follow";
const postsTopic = "postsTopic";
const THUMB_MEDIUM_SIZE = 1024; //px const THUMB_SMALL_SIZE = 100; //px
const THUMB_MEDIUM_DIR = "medium"; const THUMB_SMALL_DIR = "small";
const gcs = require('@google-cloud/storage')(); const path = require('path'); const sharp = require('sharp'); const os = require('os'); const fs = require('fs');
exports.pushNotificationLikes = functions.database.ref('/post-likes/{postId}/{authorId}/{likeId}').onCreate((snap, context) => {
});
exports.pushNotificationComments = functions.database.ref('/post-comments/{postId}/{commentId}').onCreate((snap, context) => {
});
exports.pushNotificationNewPost = functions.database.ref('/posts/{postId}').onCreate((snap, context) => { const postId = context.params.postId;
});
exports.addNewPostToFollowers = functions.database.ref('/posts/{postId}').onCreate((snap, context) => { const postId = context.params.postId;
});
exports.removePostFromFollowingList = functions.database.ref('/posts/{postId}').onDelete((snap, context) => { const postId = context.params.postId; const authorId = snap.val().authorId;
});
exports.generateThumbnail = functions.storage.object().onFinalize((object) => { const fileBucket = object.bucket; // The Storage bucket that contains the origin file. const filePath = object.name; // File path in the bucket. const contentType = object.contentType; // File content type.
});
function generateThumbnailsGeneral(fileBucket, filePath, contentType) { console.log("fileBucket", fileBucket); console.log("filePath", filePath); console.log("contentType", contentType);
}
function createThumb(fileName, originFilePath, tempFilePath, thumbDir, size, bucket) { const newFileTemp = path.join(os.tmpdir(),
${fileName}_${size}_tmp.jpg
); const newFilePath = path.join(path.dirname(originFilePath), thumbDir, fileName);}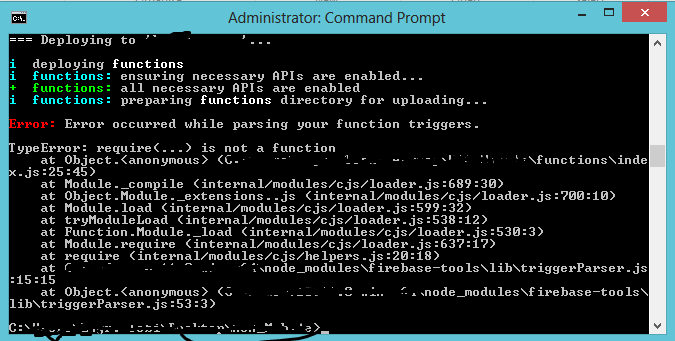
Please is anyone there with good pointers as to why this error occurs? `