Closed kpishere closed 4 months ago
It looks like what you're trying to do is expose two serial port interfaces, but you've implemented it as two usb devices. Unfortunately, your microcontroller is only a single device and it cannot act is two devices simultaneously. What you'd want is to expose two interfaces as an interface association as a single device. This is something the usbd_serial
crate would have to support for you to use. Otherwise you'll have to do it yourself.
Just to follow up, this should be supported with the current implementation. You would simply create multiple usbd_serial::SerialPort
items and change the device_class
to 0 (https://www.usb.org/defined-class-codes).
That being said, I was trying to do this with the usbd_serial
crate and am having trouble getting both ports to enumerate. https://github.com/rust-embedded-community/usbd-serial/issues/34 should be the proper issue for tracking this behavior.
I'm trying to create two serial devices over USB and I get an error at initialization of the second serial device.
The above code crashes at
let serial_log = SerialPort::new(usb_bus);
In that call, lower down, here is where it fails.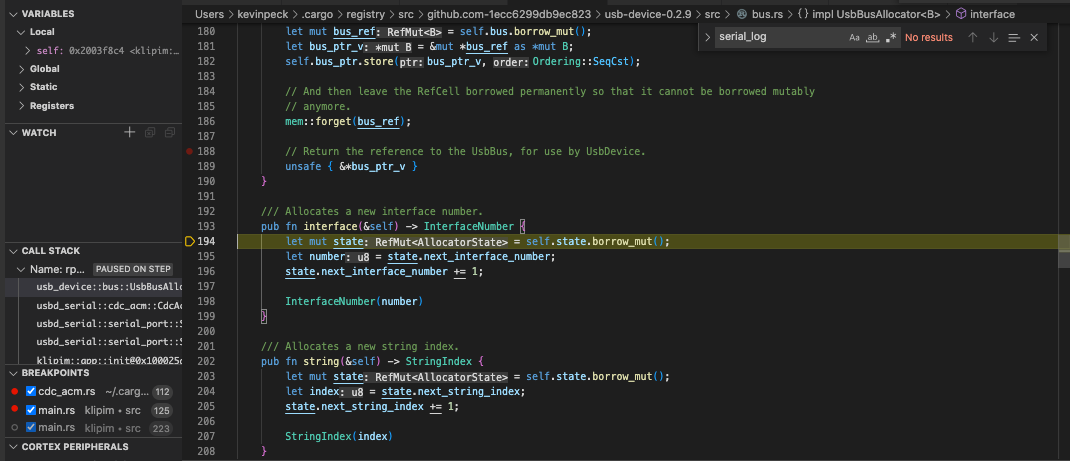