Open aligoglos opened 3 years ago
I also encounter the same issue (output grayscale when single frame as input). Have you addressed this issue?
I encounter this issue, too. Is there anyone make it ?
I also encounter the same issue (output grayscale when single frame as input). Have you addressed this issue?
Dr zhao, have you overcome this problem?
You have to emulate multiple frames by duplicating the image to make the temporal convolutions work:
input = torch.tile(input, (1, 1, 5, 1, 1))
The network still isn't able to use colors from the reference images if they are significantly different from the gray image.
I wrote simple code to run model on a single image but result is gray still !! minimal demo :
input image :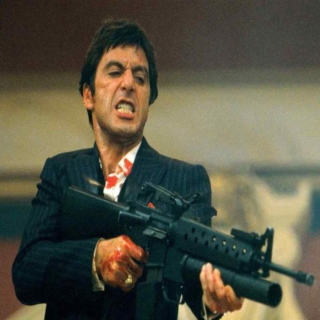
out put :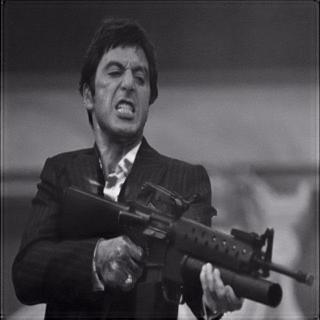
** Note : reference image is equal to input