Adds middleware to your express app to serve the Swagger UI bound to your Swagger document. This acts as living documentation for your API hosted from within your app.
MIT License
1.42k
stars
225
forks
source link
Textfield immediately clear when move out the focus #231
I try to test my APIs using swagger-ui-express version 4.1.5, but when I lose focus on id or password text field, the input was immediately cleared. But there are no problems with another text field. Like this.
By the way, I solve this problem by changing swagger-ui-express version from 4.1.5 to 3.0.10 but version 4.1.5 still has this miracle bug.
I hope someone can fix this bug. Thank you for reading.
After 3.0.10 is when we moved to using swagger-ui-dist. Based on this and looking at the description the issue is most likely related to the swagger-ui project and you should raise it there.
This is my Swagger Configuration
config/swagger.ts
index.ts
Here is my sample API description
docs/auth.yaml
package.json
I try to test my APIs using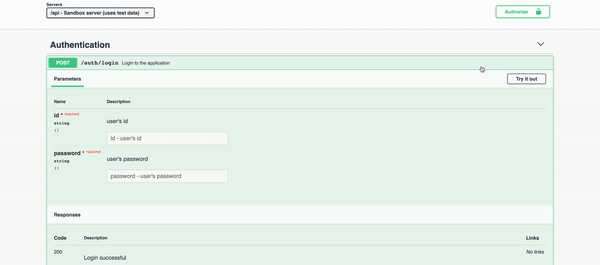
swagger-ui-express
version4.1.5
, but when I lose focus onid
orpassword
text field, the input was immediately cleared. But there are no problems with another text field. Like this.By the way, I solve this problem by changing
swagger-ui-express
version from4.1.5
to3.0.10
but version4.1.5
still has this miracle bug.I hope someone can fix this bug. Thank you for reading.