I have written a Python code to send a POST request to a URL using the requests library. The code works fine when used outside Scrapy. However, when I tried to use the same code in Scrapy shell, it did not work and returned an error.
I have attached the code below for reference. The first snippet uses requests library and returns a 200 response when executed. The second snippet uses Scrapy's Request object to send the POST request. Although it uses the same headers, cookies, and body as the first snippet, it does not work in Scrapy shell and throws an error.
Please note that I have also attached a screenshot of the error message for reference.
This is the Scrapy issue tracker, please ask questions about your code on suitable platforms. If you think you've found a bug in Scrapy you need to provide a minimal reproducible example of it.
I have written a Python code to send a POST request to a URL using the
requests
library. The code works fine when used outside Scrapy. However, when I tried to use the same code in Scrapy shell, it did not work and returned an error.I have attached the code below for reference. The first snippet uses
requests
library and returns a 200 response when executed. The second snippet uses Scrapy'sRequest
object to send the POST request. Although it uses the same headers, cookies, and body as the first snippet, it does not work in Scrapy shell and throws an error.Please note that I have also attached a screenshot of the error message for reference.
This code returns a 200 response, but it doesn't work when used in scrapy shell.
Using requests code in scrapy shell won't work but outside scrapy it will.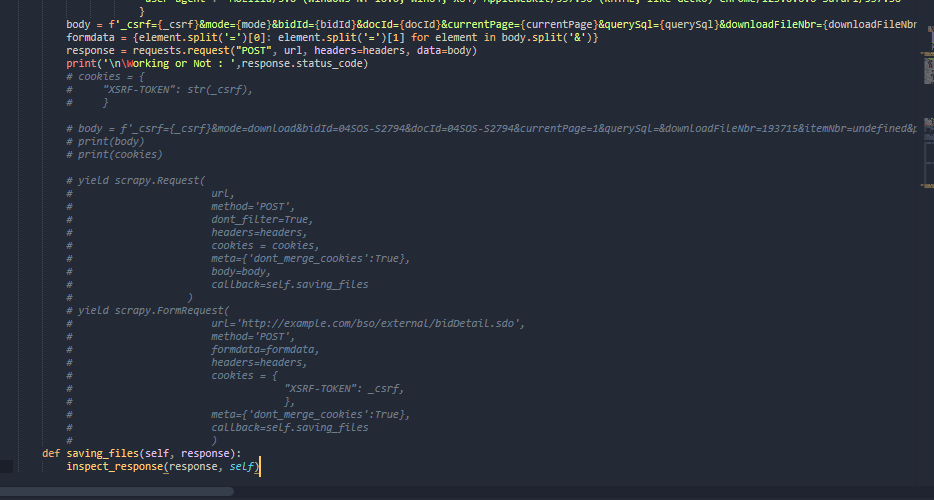