Open xdek42 opened 4 years ago
Both experiences seem to be related to the same issue, which is memory exhaustion. How much memory did you allocate to FlowDroid? Note that you may need to explicitly increase the Java heap space using the -Xmx
parameter, e.g., -Xmx4g
for 4 GB of heap space.
You can also try to use a different FlowDroid configuration if you are short on memory on your machine: https://github.com/secure-software-engineering/FlowDroid#configuring-flowdroid-for-performance
Thank you for your reply. my -Xms default configuration is 4GB, When I increase the java heap memory to 16G, the program does not throw gc exception, but the thread blocks.
The error occurred in the TypeAssigner step of the jimplify process, I think the reason may be that the code of the target function (Lcom/paopao/lucky/LuckyAutoActivity;->Myclick) caused some kind of infinite loop during the TypeAssigner process, causing soot to allocate memory all the time.
Actual debugging verified my guess, I found an infinite loop.
package soot.jimple.toolkits.typing.fast;
public class TypeResolver {
private Collection<Typing> applyAssignmentConstraints(Typing tg, IEvalFunction ef, IHierarchy h) {
final int numAssignments = this.assignments.size();
LinkedList<Typing> sigma = new LinkedList<Typing>(), r = new LinkedList<Typing>();
if (numAssignments == 0) {
return sigma;
}
HashMap<Typing, BitSet> worklists = new HashMap<Typing, BitSet>();
sigma.add(tg);
BitSet wl = new BitSet(numAssignments - 1);
wl.set(0, numAssignments);
worklists.put(tg, wl);
//Here is an infinite loop
while (!sigma.isEmpty()) {
tg = sigma.element();
wl = worklists.get(tg);
...
...
}
Hello, I encountered two errors when converting Dalvik bytecode to Jimple using Soot, my code is as follows:
1. java.lang.OutOfMemoryError: GC overhead limit exceeded
Sample Apk: https://drive.google.com/open?id=1IX323NMM0M3YKLWSVT2v-u7UNNwCUEXT When I analyze this apk, FlowDroid will encounter OutOfMemoryError
2. Thread Blocking
Sample Apk: https://drive.google.com/open?id=1fJCSJchxoHhr5skvpAhANPI5l3UsFQ3Q When I analyze this apk, all threads of FlowDroid will be blocked, except one thread. The program does not throw any exceptions, but it will not terminate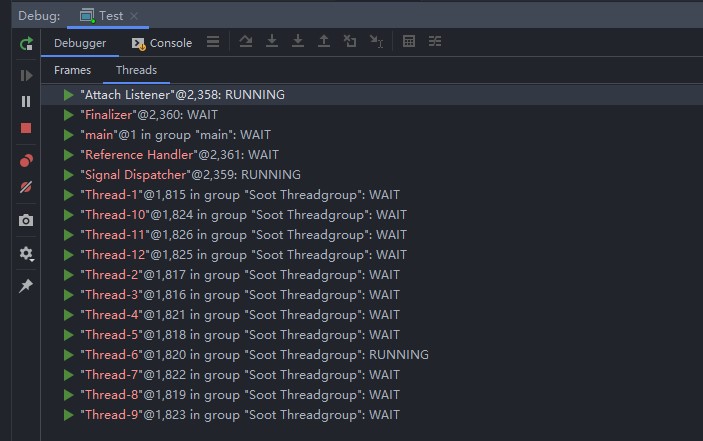
How can i fix these two errors ?Look forward to your help