Closed baehongjun0212 closed 3 years ago
My only guess is that you're using HLS for Safari, so it may be going through the browser's native HLS implementation instead of using MediaSource. I could imagine an implementation of HLS in the browser that could bypass WebAudio.
Try this configuration in Shaka:
player.configure('streaming.useNativeHlsOnSafari', false);
This will prefer MediaSource to native HLS. On iOS, we will still be forced to use native HLS, so I don't expect this to be effective on iOS. But it may work in desktop Safari.
Does this help?
@baehongjun0212 Does this answer all your questions? Can we close the issue?
Closing due to inactivity. If this is still an issue for you or if you have further questions, you can ask us to reopen or have the bot reopen it by including @shaka-bot reopen
in a comment.
Have you read the Tutorials? Yes
Have you read the FAQ and checked for duplicate open issues? Yes; #2595 #3484 #3563
What version of Shaka Player are you using? 3.2.0
Please ask your question The sound of the video was visualized as a canvas using sample code from #2595. But in safari, they don't draw anything on the canvas. How can we solve this?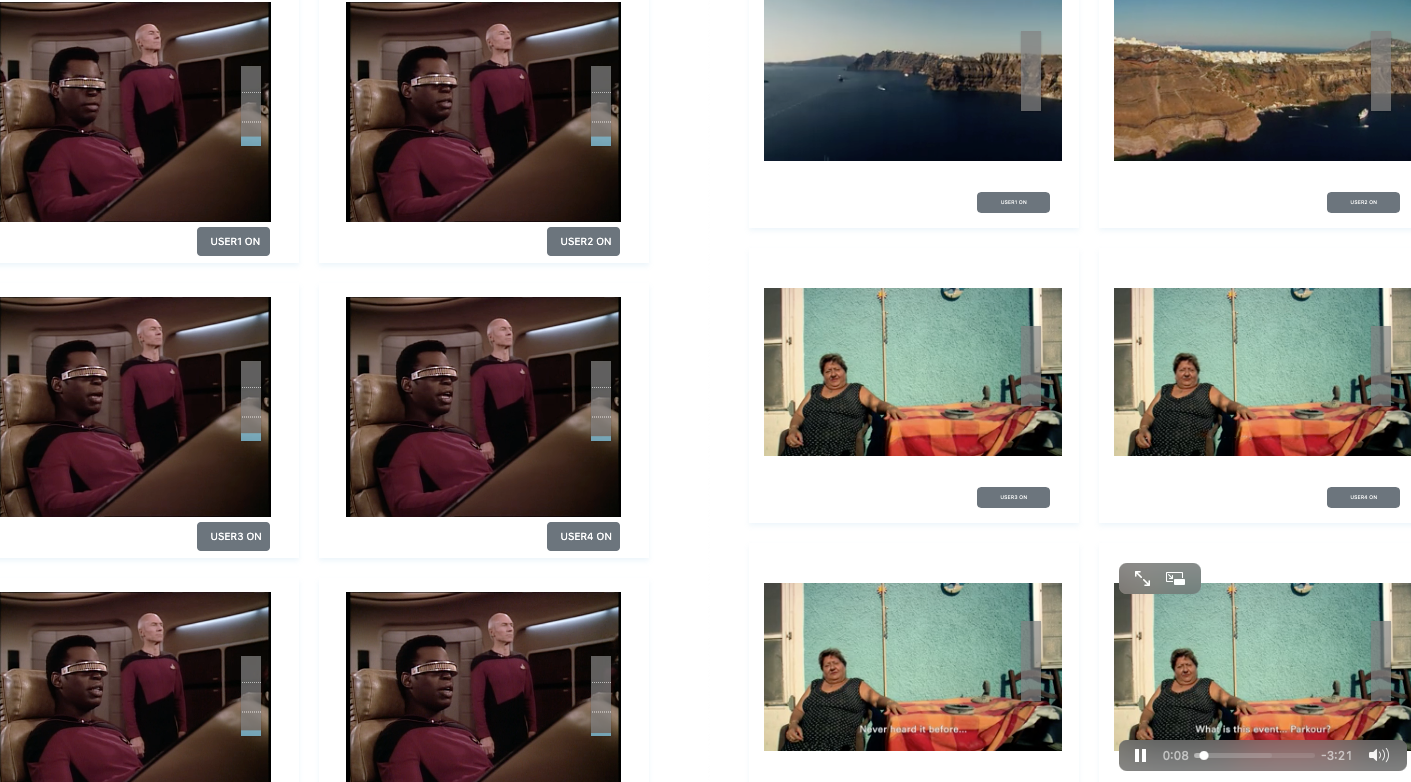
I'll attach my code.