Closed shoutingwei closed 6 years ago
offsetTop: 元素外边框距离offsetParent的上内边框的距离
offsetLeft: 元素外边框距离offsetParent的左内边框的距离
offsetParent 是一个只读属性,返回一个指向最近的(closest,指包含层级上的最近)包含该元素的定位元素。如果没有定位的元素,则 offsetParent 为最近的 table 元素对象或根元素(标准模式下为 html;怪异模式下为 body)。当元素的 style.display 设置为 none 或定位为fixed时,offsetParent 返回 null
scrollLeft: 被隐藏在内容区域左侧的宽度(有横向滚动条时)
scrollTop: 被隐藏在内容上边区域的高度(有竖向滚动条时)
浏览器可视区宽高
// 不包含滚动条
// width
document.documentElement.clientWidth
// height
document.documentElement.clientHeight
// 包含滚动条(ie9+, 不是css规范)
// width
window.innerWidth
// height
window.innerHeight
元素滚动隐藏区域宽高
// top
el.scrollTop;
// left
el.scrollLeft;
//文档滚动大小
// top
document.body.scrollTop
// left
document.body.scrollLeft
元素距离文档顶部大小
// 当offsetParent为body时
// top
el.offsetHeight;
// 当offsetParent不为body时,需要一层层循环至offsetParent为null(通用方法)
// top
function getTop(el){//不加border??
var top = el.offsetTop,
currentParent = el.offsetParent;
while(currentParent != null){
top += currentParent.offsetTop;
currentParent = currentParent.offsetParent;
}
return top;
}
元素距离文档左侧大小(与上面类似)
// left
el.offsetLeft;
// left
function getLeft(el){
var top = el.offsetLeft,
currentParent = el.offsetParent;
while(currentParent != null){
top += currentParent.offsetLeft;
currentParent = currentParent.offsetParent;
}
return top;
}
元素距离视口顶部大小
// 元素距离视口顶部大小 = 元素距离文档顶部大小 - 文档正文垂直滚动高度;
function topToViewport(el){
return getTop(el) - document.body.scrollTop;
}
元素距离视口左侧大小
// 元素距离视口顶部大小 = 元素距离文档左侧大小 - 文档正文水平滚动高度;
function leftToViewport(el){
return getLeft(el) - document.body.scrollLeft;
}
判断元素是否在可视区
// 文档正文垂直滚动高度 < 可视区域范围 < (浏览器可视区高度+文档正文垂直滚动高度)
function isOnViewport(el){
var offsetTop = getTop(el), // 元素距离顶部高度
st = document.body.scrollTop, // 文档正文垂直滚动高度
vh = document.documentElement.clientHeight; // 视口高度
return (offsetTop > st) && (offsetTop < st + vh);
}
判断是否滚动到底部
function isBottom(){
var currentTop = document.body.scrollTop + document.documentElement.clientHeight;
var totalTop = document.documentElement.offsetHeight;
return currentTop == totalTop;
}
**鼠标点击位置坐标
相对于屏幕**
function getMousePos(event) {
var e = event || window.event;
return {'x':e.screenX,'y':screenY}
}
相对浏览器窗口
function getMousePos(event) {
var e = event || window.event;
return {'x':e.clientX,'y':clientY}
}
相对文档
function getMousePos(event) {
var e = event || window.event;
var scrollX = document.documentElement.scrollLeft || document.body.scrollLeft;
var scrollY = document.documentElement.scrollTop || document.body.scrollTop;
var x = e.pageX || e.clientX + scrollX;
var y = e.pageY || e.clientY + scrollY;
//alert('x: ' + x + '\ny: ' + y);
return { 'x': x, 'y': y };
}
body是DOM对象里的body子节点,即
标签;documentElement 是整个节点树的根节点root,即 标签;
https://segmentfault.com/a/1190000005155278 https://www.cnblogs.com/dolphinX/archive/2012/11/19/2777756.html
The Element.clientWidth property is zero for elements with no CSS or inline layout boxes, otherwise it's the inner width of an element in pixels. It includes padding but not the vertical scrollbar (if present, if rendered), border or margin.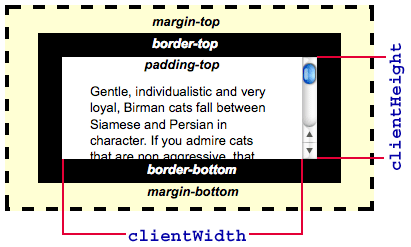
The Element.scrollWidth read-only property is a measurement of the width of an element's content, including content not visible on the screen due to overflow.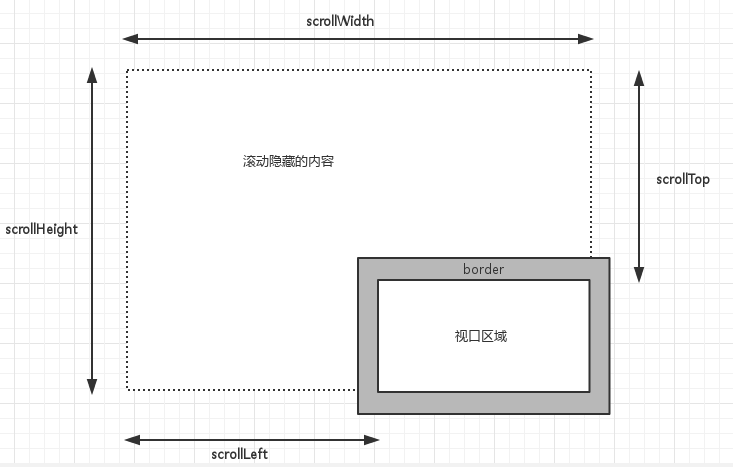
The scrollWidth value is equal to the minimum width the element would require in order to fit all the content in the viewport without using a horizontal scrollbar. The width is measured in the same way as clientWidth: it includes the element's padding, but not its border, margin or vertical scrollbar (if present). It can also include the width of pseudo-elements such as ::before or ::after. If the element's content can fit without a need for horizontal scrollbar, its scrollWidth is equal to clientWidth
The HTMLElement.offsetWidth read-only property returns the layout width of an element. Typically, an element's offsetWidth is a measurement which includes the element borders, the element horizontal padding, the element vertical scrollbar (if present, if rendered) and the element CSS width. If the element is hidden (for example, by style.display on the element or one of its ancestors to "none"), then 0 is returned.