Closed ferdiamg closed 3 years ago
@ferdiamg Did you find solution?
I have almost same query, I need city state country separately
Had a similar requirement and made a workaround for it.Try this solution on this pull request
@nadesh-git Thanks for the solution and PR.
Here is example to get city, state and country.
const apiKey = "API_KEY_GOES_HERE";
const language = "de";
const region = "de";
const locationType = "ROOFTOP";
let city, state, country;
Geocode.fromLatLng(
"48.8583701",
"2.2922926",
apiKey,
language,
region,
locationType
).then(
(response) => {
for (let i = 0; i < response.results[0].address_components.length; i++) {
for (let j = 0; j < response.results[0].address_components[i].types.length; j++) {
switch (response.results[0].address_components[i].types[j]) {
case "locality":
city = response.results[0].address_components[i].long_name;
break;
case "administrative_area_level_1":
state = response.results[0].address_components[i].long_name;
break;
case "country":
country = response.results[0].address_components[i].long_name;
break;
}
}
}
console.log(city, state, country);
},
(error) => {
console.error(error);
}
);
If I access the results like the following:
I get this as an outcome for San Francisco: SFLO, Van Ness Ave, San Francisco, CA 94102, USA and for Berlin I get this: B2 7, 10178 Berlin, Deutschland
How can I access ONLY the city in that way for users from many different locations? Thats almost impossible, since even a regex can't work on a non-deterministic outcome.
If I access the whole response object, theres still no option to ONLY get the city: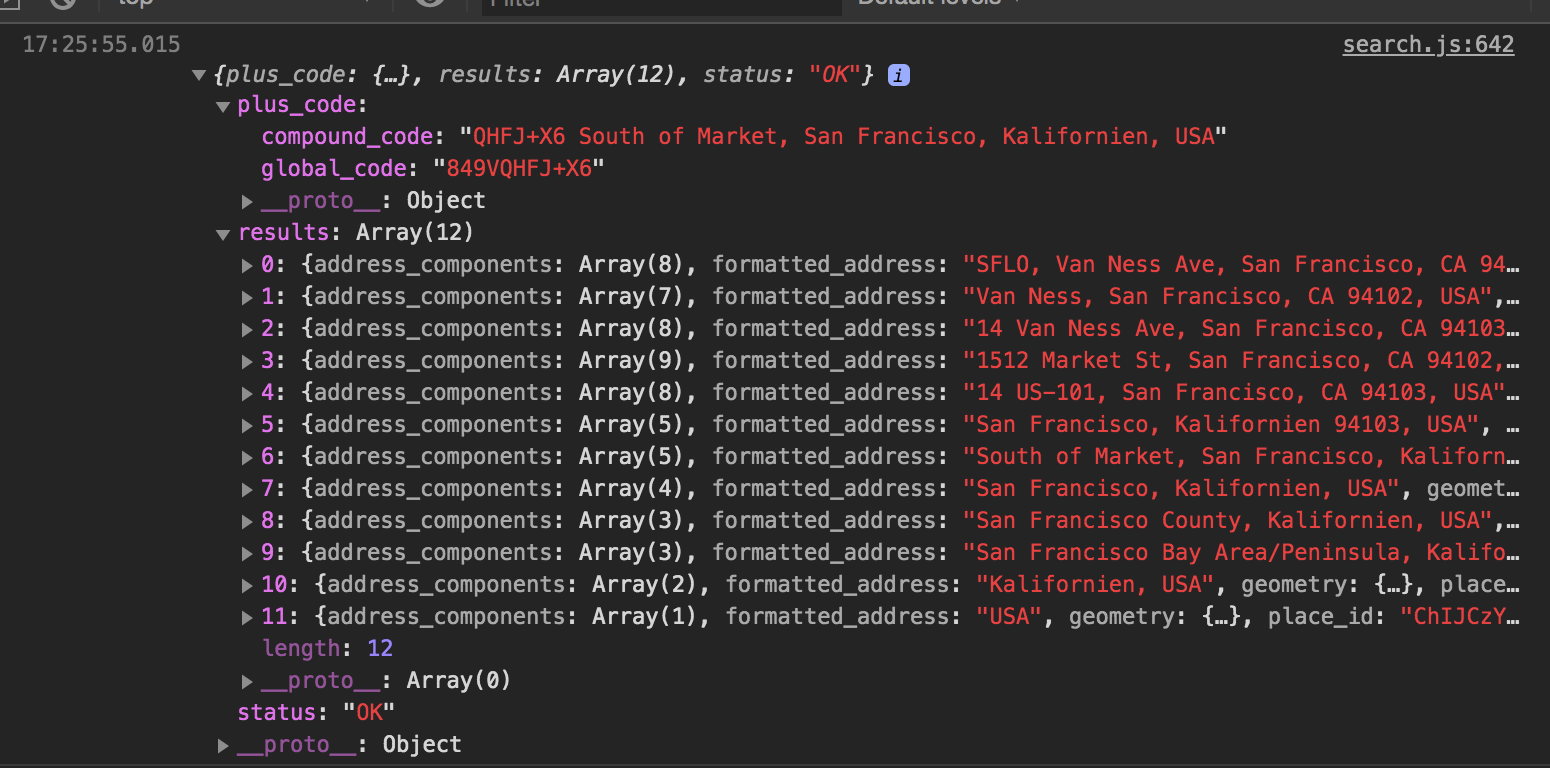