Closed cschuhknecht closed 3 years ago
Hi epiCS97, try the following code, it may be helpful. Let me know!
Stefano
P.S.: remember to add the bot to a chat group ;-)
#include <CTBot.h>
#include <Utilities.h> // for int64ToAscii() helper function
#define WIFI_SSID "YOUR _SSID"
#define WIFI_PSW "YOUR_PASSWORD"
#define TOKEN "YOUR_TELEGRAM_TOKEN"
CTBot myBot;
void setup() {
Serial.begin(115200); // initialize the serial
delay(1000);
myBot.wifiConnect(WIFI_SSID, WIFI_PSW); // connect to the wifi network
myBot.setTelegramToken(TOKEN); // set the telegram bot token
Serial.println("\nBot online!");
}
void loop() {
TBMessage msg;
// check if there is a new incoming message
if (myBot.getNewMessage(msg)) {
// check if the message is a text message
if (msg.messageType == CTBotMessageText) {
// print some message related details to the sender account
myBot.sendMessage(msg.sender.id, "ID: " + (String)msg.sender.id +
"\nfirstName: " + msg.sender.firstName +
"\nlastName: " + msg.sender.lastName +
"\nusername: " + msg.sender.username +
"\nMessage: " + msg.text +
"\nChat ID: " + int64ToAscii(msg.group.id) +
"\nChat title: " + msg.group.title);
// check if the message comes from a chat group (the group.id is negative)
if (msg.group.id < 0) {
// echo the message to the chat group
Serial.printf("Chat ID: %ld\n", msg.group.id);
myBot.sendMessage(msg.group.id, msg.text);
}
}
}
delay(500); // wait 500 milliseconds
}
That Code helped. I messed with the type of the Group Chat ID. Thank you.
Hi, i tried to send a message to a group chat. When I paste my personal ID into the following line of code it works. But not with the group chat. The Group ID has a '-' sign in front of the integers, maybe thats why.
myBot.sendMessage(MyID, "TEST");
I also noticed, I can't do TBGroup Type: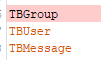
Thanks in advance.