Closed gaetanmrnd closed 4 years ago
You can set alpha to a smaller value. This will reduce the regularization force that keeps the point together during non-rigid transformation.
With that being said, non-rigid deformation is not a suitable transformation for modelling a 3D-to-2D projection. You should look into a projection matrix. Unfortunately, I have not implemented such a transformation, and I do not intend to as this was not covered in the original CPD paper.
Hi, Thanks for your CPD implementation.
I'm having trouble matching a 3D model to points extracted from a 2D picture. It works fine with the affine deformation, but the result is not very precise (I'm guessing because the pinhole camera model needs nonrigid deformation).
When I try the nonrigid deformation, the source data is not modified and the error is unbelievably small. I don't understand, am I doing anything wrong or is there a problem with the deformable_registration class? I'm attaching my code (very close to the example code) and the results in both cases.
Thanks,
Gaetan
Affine: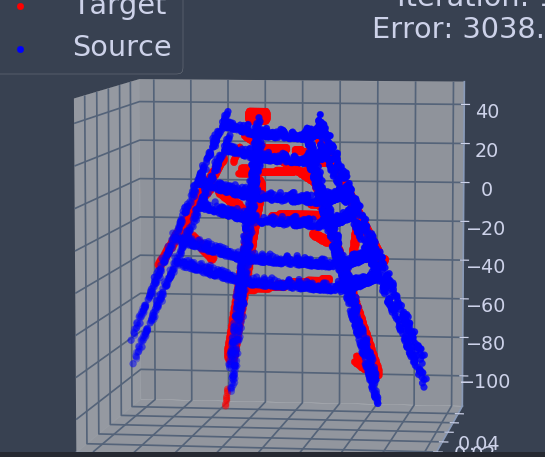
Nonrigid: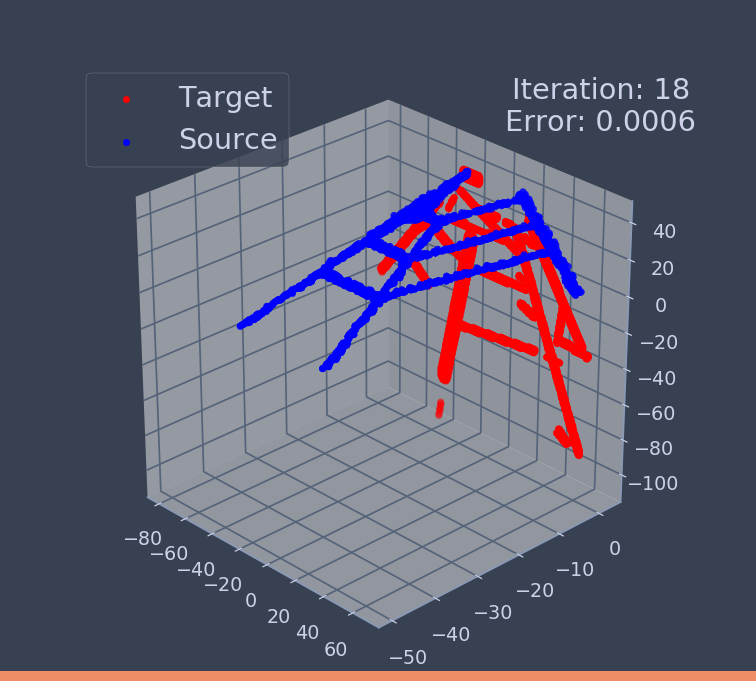