Open bearwmceo opened 4 years ago
The third parameter of the colorRegions
method will help keep letters together when they're made up of separate lines which aren't touching, and the exact_characters
setting can be used whenever a CAPTCHA system always has exactly the same number of characters.
These settings seem to work well.
var cbl = new CBL({
preprocess: function(img) {
img.debugImage("debugPreprocessed");
img.binarize(200);
img.debugImage("debugPreprocessed");
img.colorRegions(50, true, 3);
img.debugImage("debugPreprocessed");
},
character_set: "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789",
exact_characters: 5,
pattern_width: 25,
pattern_height: 25,
blob_min_pixels: 10,
blob_max_pixels: 1000,
allow_console_log: true,
blob_console_debug: true,
perceptive_colorspace: true,
blob_debug: "debugSegmented"
});
cbl.train("3jrH5.png");
cbl.train("bHaGR.png");
cbl.train("GCXKa.png");
cbl.train("gSBTt.png");
cbl.train("mXTtA.png");
cbl.train("PWRSX.png");
var saveModel = function() {
cbl.condenseModel();
cbl.sortModel();
cbl.visualizeModel("visualizeModel");
cbl.saveModel();
}
Captchas.zip I tried with this captchas but It's not working always
Did you train a model? What's your solver look like? You can check out the quick start for help in getting started.
I ran through the training for the images above and generated this model:
You may want to train on more samples or play around with the segmentation settings, but it performs decently.
<div class="main">
<img id="captcha" src="mXTtA.png" />
<br />
<input type="text" id="solution">
<br />
<a href="javascript: void(0)" id="solve" onclick="solve()" style="display: none">Solve!</a>
</div>
<script>
var cbl = new CBL({
preprocess: function(img) {
img.binarize(200);
img.colorRegions(50, true, 3);
},
/* Load the model we saved during training. */
model_file: "condensed-30.txt",
character_set: "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789",
exact_characters: 5,
pattern_width: 25,
pattern_height: 25,
blob_min_pixels: 10,
blob_max_pixels: 1000,
//allow_console_log: true,
//blob_console_debug: true,
perceptive_colorspace: true,
//blob_debug: "debugSegmented",
/* Define a method that fires immediately after successfully loading a saved model. */
model_loaded: function() {
// Don't enable the solve button until the model is loaded.
document.getElementById('solve').style.display = "block";
}
});
var solve = function() {
// Using the saved model, attempt to find a solution to a specific image.
cbl.solve("captcha").done(function (solution) {
// Upon finding a solution, fill the solution textbox with the answer.
document.getElementById('solution').value = solution;
});
}
</script>
Hi, I tried to resolve this captcha but without success, I hope someone can help me.
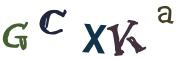