Open diepthaiphu opened 4 years ago
First of all you need to increase the blob_max_pixels
since those characters are pretty large. You may also want to switch to comparing colors in a perceptive colorspace with perceptive_colorspace
and remove any image preprocessing that changes the colors to black before the colorRegions
(this is because each character is a separate color, which makes it easier to segment later). I've also used a new command called removeLight
, so you'll want to get the latest version of the library to use it.
var cbl = new CBL({
preprocess: function(img) {
img.debugImage("debugPreprocessed");
img.removeLight(128);
img.debugImage("debugPreprocessed");
img.colorRegions(14, true);
img.debugImage("debugPreprocessed");
},
character_set: "0123456789",
exact_characters_width: 50,
exact_characters_play: 2,
exact_characters: 4,
blob_min_pixels: 50,
blob_max_pixels: 100000,
blob_max_width: 50,
pattern_width: 20,
pattern_height: 20,
allow_console_log: true,
blob_console_debug: true,
perceptive_colorspace: true,
blob_debug: "debugSegmented"
});
cbl.train("3458.jpg");
cbl.train("4273.jpg");
Play around with that and see if it helps.
Can you help resolve this
train
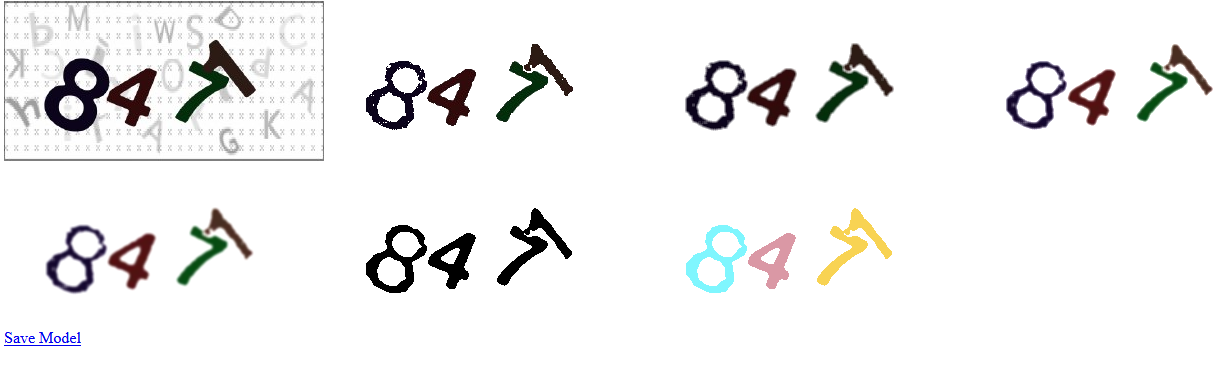