Open sloway opened 2 years ago
영상에서는 3가지로 설명하고 있지만 하나를 분리해서 4가지로 기록함
three.js official document 의 kr/en 의 code snippet이 가 달라서 둘 다 기록함
// KR version
<script type="module">
// Find the latest version by visiting https://cdn.skypack.dev/three.
// 위 주속에 쓰여진대로 아래 <version> 은 버전을 찾아서 바꿔줘야 함
import * as THREE from 'https://cdn.skypack.dev/three@<version>';
const scene = new THREE.Scene();
// EN // Since Import maps are not yet supported by all browsers, it is necessary to add the polyfill es-module-shims.js.
### 3. npm 을 이용한 설치
- Node Pacakge Manager를 이용해서 three package 설치
[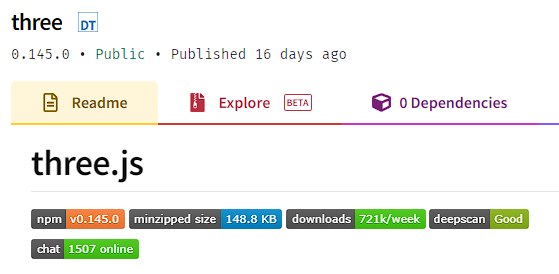](https://www.npmjs.com/package/three)
- 2번(runtime loading) 과 다르게 미리 설치 받아놓고 사용(buildtime loading) 하는 형태
- NPM 을 사용하기는 하지만 rumtime 이 node.js 는 아님
npm install --save three
// Option 1: Import the entire three.js core library. import * as THREE from 'three'; const scene = new THREE.Scene();
// Option 2: Import just the parts you need. import { Scene } from 'three'; const scene = new Scene();
### 4. Boilerplate 를 이용한 설치
- https://github.com/aakatev/three-js-webpack
- 영상에서는 위 경로의 boilerplate 를 이용했음
- 위 경로의 project는 npm 으로 three.js 를 설치하고 webpack 으로 실행하도록 가이드
- 만들어진 project 중에서 불필요한 코드는 제거가능
---
여러가지 방법이 소개되었지만 결국 가장 많이 사용하는 방법은 아래 2개일 것임
- Runtime loading with CDN
- Buildtime loading with NPM
Node.js 나 NPM 을 좋아하긴 하지만,
(Webpack 등 다른 npm package 들을 이용하지 않을 거라면)
Tutorial 수준에서는 Runtime loading 이면 충분하지 않을까?
Unity 에서의 개념과 동일하기 때문에 이 부분에 대한 이해는 크게 어렵지 않음 그래도 Three.js official guide에 설명이 잘 되어 있어서 한번 읽어보아야 겠음
여기서 작성한 Code는 간단한 WebGL 작업 할 때 skeleton code로 이용해도 되겠음. Custom code snippet으로 만들어볼까???
const geometry = new THREE.BoxGeometry(0.5, 0.5, 0.5);
const material = new THREE.MeshStandardMaterial({ color: 0x999999 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
// alpha 는 anti-aliasing 과는 무관
const renderer = new THREE.WebGLRenderer({ antialias: true, alpha: true });
function OnWindowResize() {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
}
window.addEventListener("resize", OnWindowResize);
이번 영상에서는 pointlight 추가 해봄
const light = new THREE.PointLight(0xffffff, 1);
light.position.set(0, 2, 12);
scene.add(light);
const materials = [
new THREE.MeshStandardMaterial({ map: textureBaseColor }),
new THREE.MeshStandardMaterial({
map: textureBaseColor,
normalMap: textureNormalMap,
}),
new THREE.MeshStandardMaterial({
map: textureBaseColor,
normalMap: textureNormalMap,
displacementMap: textureHeightMap,
displacementScale: 0.1,
}),
new THREE.MeshStandardMaterial({
map: textureBaseColor,
normalMap: textureNormalMap,
displacementMap: textureHeightMap,
displacementScale: 0.1,
roughnessMap: textureRoughnessMap,
roughness: 0.8,
}),
];
height map 은 차이가 확 느껴지는데 다른 map은 아직은 잘....
각 Light마다 Helper class 가 존재함.
Helper class를 만들어서 Scene 에 추가하면 빛에 대한 정보가 화면에 그려짐
주로 Constructor arguments 를 보면 특성이 보이기는 함. 하지만 6가지를 필요에 따라 잘 쓰려면 경험이 좀 필요해 보임. 나는 일단은 DirectionalLight 면 충분해 보임
renderer.shadowMap.enabled = true; // Renderer 에서 활성화
light.castShadow = true; // 그림자를 cast
light.shadow.mapSize.width = 2048; // mapSize 가 클 수록 그림자 계단 현상 없음
light.shadow.mapSize.height = 2048;
light.shadow.radius = 8; // radius 가 클수록 그림자 경계가 부드러워짐
plane.receiveShadow = true; // 그림자를 cast
mesh.castShadow = true; // 그림자를 Receive
//결론적으로 light 와 mesh 에 의해서 만들어진 그림자를 plane 에 표시
https://threejs.org/docs/#examples/en/controls/OrbitControls
import { OrbitControls } from "orbitcontrols";
const controls = new OrbitControls(camera, renderer.domElement); controls.enableDamping = true; controls.minDistance = 2; // Zoon in 한계 controls.maxDistance = 6; // Zoom out 한계 controls.maxPolarAngle = Math.PI / 2; // Camera가 바닥 아래로 내려가지 않음 controls.update();
function render(time) { time *= 0.001; meshes.forEach((mesh) => { mesh.rotation.y = time; }); controls.update(); // render 에도 설정 renderer.render(scene, camera); requestAnimationFrame(render); }
requestAnimationFrame(render);
업무는 주로 Unity 로 진행하고 있지만, Project 성격이나 Runtime env 에 따라서 Unity보다 WebGL 이 더 좋을 때가 있음 그래서 한번은 배워둘 필요성을 느끼던 중에 괜찮은 강의를 발견해서 연습하려고 함