Closed johann-petrak closed 1 year ago
I am pretty sure this happens if one of the angles values is float("nan")
It may be useful for the library to either ignore any NaN value or throw an exception giving the reason.
@johann-petrak I can't reproduce that behaviour using float("nan"), can you post the script you used for reproducibility?
#!/usr/bin/env python
"""
Module for debugging the robot disappears bug (qibullet #84)
"""
import qibullet
from qibullet import SimulationManager
import time
import numpy as np
PEPPER_ANGLES=dict(
HeadYaw=(-2.0857, 2.0857),
HeadPitch=(-0.7068, 0.4451),
RShoulderPitch=(-2.0857, 2.0857),
RShoulderRoll=(-1.5620, -0.0087),
RElbowYaw=(-2.0857, 2.0857),
RElbowRoll=(0.0087, 1.5620),
RHand=(0.0, 1.0),
LShoulderPitch=(-2.0857, 2.0857),
LShoulderRoll=(0.0087, 1.5620),
LElbowYaw=(-2.0857, 2.0857),
LElbowRoll=(-1.5620, -0.0087),
LHand=(0.0, 1.0),
KneePitch=(-0.5149, 0.5149),
HipPitch=(-1.0385, 1.0385),
HipRoll=(-0.5149, 0.5149),
)
if __name__ == "__main__":
print("qibullet version:", qibullet.__version__)
simulation_manager = SimulationManager()
client_id = simulation_manager.launchSimulation(gui=True, auto_step=False)
pepper = simulation_manager.spawnPepper(
client_id,
translation=[0, 0, 0],
quaternion=[0, 0, 0, 1],
spawn_ground_plane=True)
print("Pepper spawned:", pepper, type(pepper))
names = [n for n in PEPPER_ANGLES.keys()]
angles = [0.0 for n in names]
speeds = [1.0 for n in names]
pepper.setAngles(names, angles, speeds)
simulation_manager.stepSimulation(client_id)
time.sleep(5.0)
iteration = 0
while True:
iteration += 1
angles = [np.random.uniform(PEPPER_ANGLES[a][0], PEPPER_ANGLES[a][1]) for a in PEPPER_ANGLES.keys()]
time.sleep(0.10)
if iteration % 5:
angleidx = np.random.randint(0, len(PEPPER_ANGLES))
angles[angleidx] = float("nan")
print(f"Setting angle {names[angleidx]} to NaN")
try:
pepper.setAngles(names, angles, speeds)
simulation_manager.stepSimulation(client_id)
except Exception as ex:
print("Exception from setAngles: ", ex)
print("Angles:", angles)
With qibullet version: 1.4.5 reproduces the problem every time.
This should now be prevented on develop, the 1.4.6 version of qibullet will raise a pybullet error when a NaN is specified
Tested and works for me, throws an exception of type pybullet.error
now
I have written a simple program which receives joint and speed data and sends it to the VirtualPepper robot using the
pepper.setAngles(names, angles, speeds)
method.This works quite well for a while but suddenly the robot just disappears from the simulation in the GUI. However now exception is thrown and nothing is shown on the console.
When would this happen? How is it possible to debug what could cause this?
This is what the GUI looks before the error: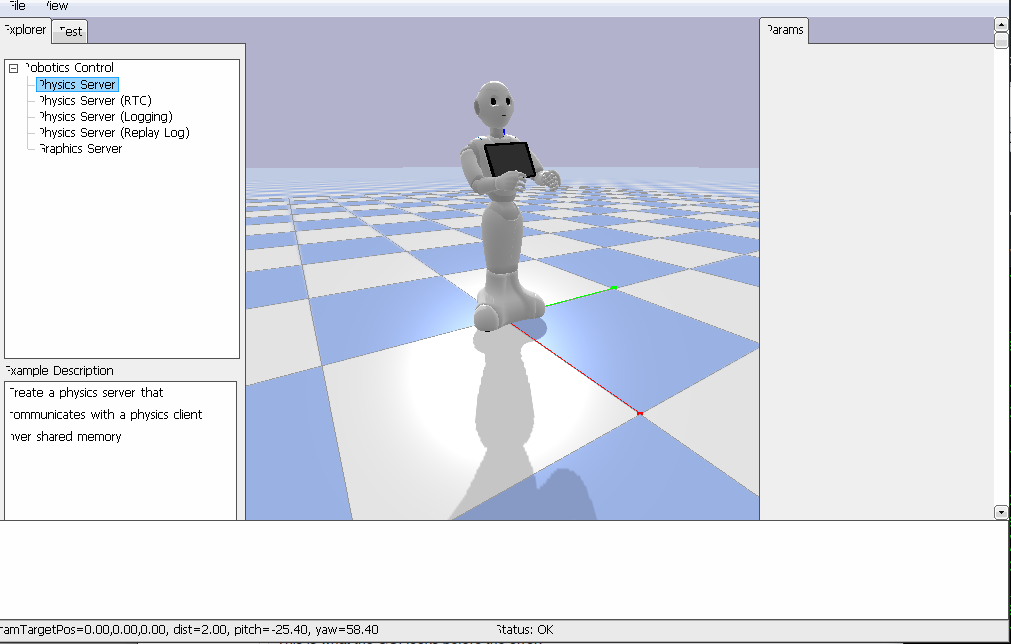
This is what the GUI looks after the error: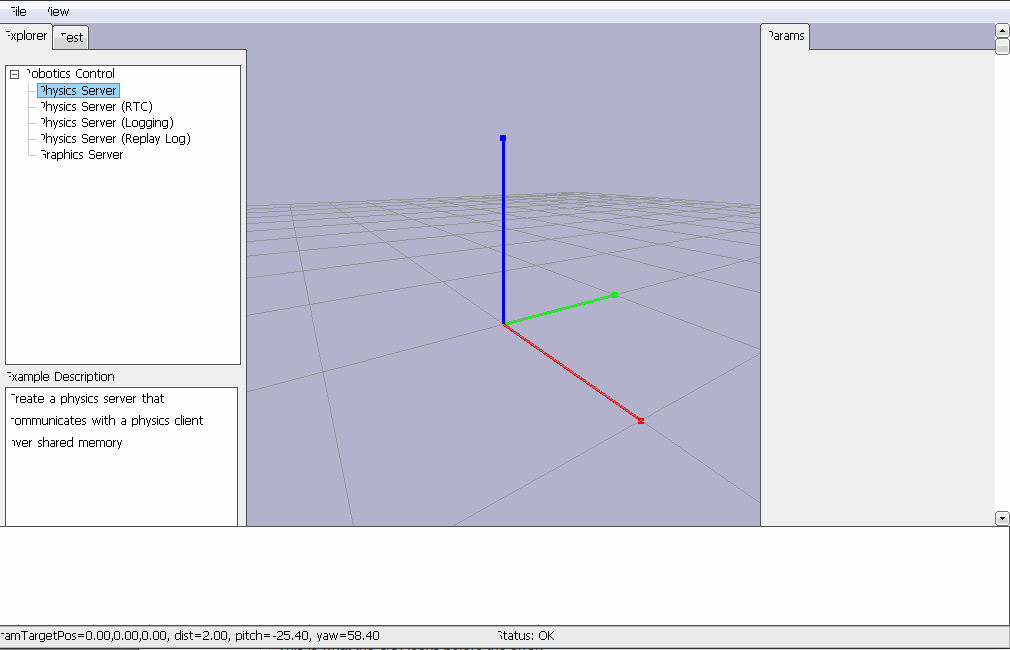