Closed TheRadly closed 4 years ago
Video guide for reproduce: https://drive.google.com/open?id=1kHiXDcp-LVLJUpk8QMwQV_hefVGaFNDZ
You are lazy. We are lazy and won't watch any video. Sorry.
Please fill out the issue template and describe the problem. Please reduce this to the minimum that is needed to get the problem
Video guide for reproduce: https://drive.google.com/open?id=1kHiXDcp-LVLJUpk8QMwQV_hefVGaFNDZ
You are lazy. We are lazy and won't watch any video. Sorry.
Please fill out the issue template and describe the problem. Please reduce this to the minimum that is needed to get the problem
I updated the comment
Show your entities configuration.
Show your entities configuration.
Hi, er1z.
<?php declare(strict_types = 1);
namespace App\Entity\KnowledgeBase;
use Doctrine\ORM\Mapping as ORM;
/**
* @ORM\Table(name="knowledge_base_category")
* @ORM\Entity(repositoryClass="Doctrine\ORM\EntityRepository")
*/
class Category.php
{
/**
* @ORM\Id()
* @ORM\Column(type="integer")
* @ORM\GeneratedValue(strategy="AUTO")
* @var int
*/
protected $id;
/**
* @ORM\Column(type="string", nullable=false)
* @var string
*/
protected $name;
public function getName(): string
{
return $this->name ?? '';
}
public function setName(string $name): void
{
$this->name = $name;
}
public function getId(): int
{
return $this->id;
}
public function setId(int $id): void
{
$this->id = $id;
}
public function __toString(): string
{
return $this->getName();
}
}
<?php declare(strict_types = 1);
namespace App\Entity\KnowledgeBase;
use Doctrine\ORM\Mapping as ORM;
use Symfony\Bridge\Doctrine\Validator\Constraints\UniqueEntity;
use Symfony\Component\Validator\Constraints as Assert;
/**
* @ORM\Table(name="knowledge_base")
* @ORM\Entity(repositoryClass="Doctrine\ORM\EntityRepository")
* @UniqueEntity(
* fields={"url"},
* message="This url is already use."
* )
*/
class KnowledgeBase
{
/**
* @ORM\Id()
* @ORM\Column(type="integer")
* @ORM\GeneratedValue(strategy="AUTO")
* @var int
*/
protected $id;
/**
* @ORM\ManyToOne(targetEntity="App\Entity\KnowledgeBase\Category")
* @ORM\JoinColumn(name="category_id", referencedColumnName="id")
* @Assert\NotBlank
* @var Category
*/
protected $category;
/**
* @ORM\ManyToOne(targetEntity="App\Entity\KnowledgeBase\Subcategory")
* @ORM\JoinColumn(name="subcategory_id", referencedColumnName="id", nullable=true)
* @var Subcategory|null
*/
protected $subcategory;
/**
* @ORM\Column(type="string")
* @var string
*/
protected $title;
/**
* @ORM\Column(type="string", unique=true, nullable=false)
* @var string
*/
protected $url;
/**
* @ORM\Column(type="text")
* @var string
*/
protected $description;
public function getId(): int
{
return $this->id;
}
public function getTitle(): string
{
return $this->title ?? '';
}
public function setTitle(string $title): void
{
$this->title = $title;
}
public function getUrl(): string
{
return $this->url ?? '';
}
public function setUrl(string $url): void
{
$this->url = $url;
}
public function getDescription(): string
{
return $this->description ?? '';
}
public function setDescription(string $description): void
{
$this->description = $description;
}
/**
* @return Category|string|null
*/
public function getCategory()
{
return $this->category;
}
public function setCategory(Category $category): void
{
$this->category = $category;
}
/**
* @return Subcategory|string|null
*/
public function getSubcategory()
{
return $this->subcategory;
}
public function setSubcategory(?Subcategory $subcategory): void
{
$this->subcategory = $subcategory;
}
}
There's your problem:
public function setCategory(Category $category): void
Closing this, as we try to keep github issues for feature requests and bugs only.
Thanks! It is works! Sorry for my carelessness :) I could really think that this is a bug
Environment
Sonata packages
Symfony packages
PHP version
Subject
Expected argument of type "App\Entity\KnowledgeBase\Category", "NULL" given at property path "category"
- This error occurs when you delete an object from a sheet and leave it empty. When created, it is validated, does not allow to save, because the 'category' field is null. When the field is not empty, everything is OK. But when you go to edit this form, and with an empty field, when you click on the "Edit" button, validation does not work, and you get an errorSteps to reproduce
Expected results
Validation should work correctly when changed
Actual results
The data in the object is the same. What when creating, what when changing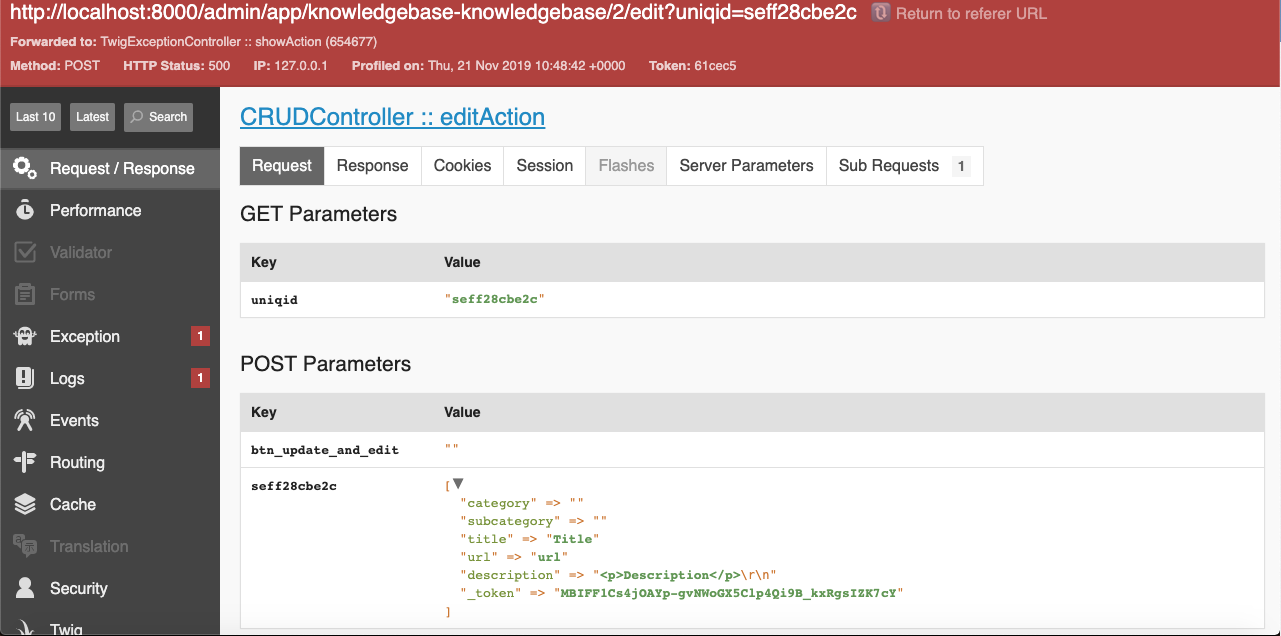