Closed vineetkala11 closed 8 months ago
Hello @spencergibb
Please share your view on this issue.
@spencergibb this issue has been reported multiple times and has been open for a really long time, is there anything that you suggest improving in the PR?
Closing in favor of https://github.com/spring-cloud/spring-cloud-gateway/pull/2774
Describe the bug
ModifyResponseBodyGatewayFilterFactory
is blocker for SSE based resources becausewriteWith
method ofModifiedServerHttpResponse
is responsible to modify response body for both stream and non stream mime-type,writeWith
convert response body to Mono, this behavior ofwriteWith
is a blocker for SSE.Due to this issue consumer of SSE endpoint getting all events in one go instead of in form of stream. Without
ModifyResponseBodyGatewayFilterFactory
things works perfectly.Current behavior of ModifyResponseBodyGatewayFilterFactory: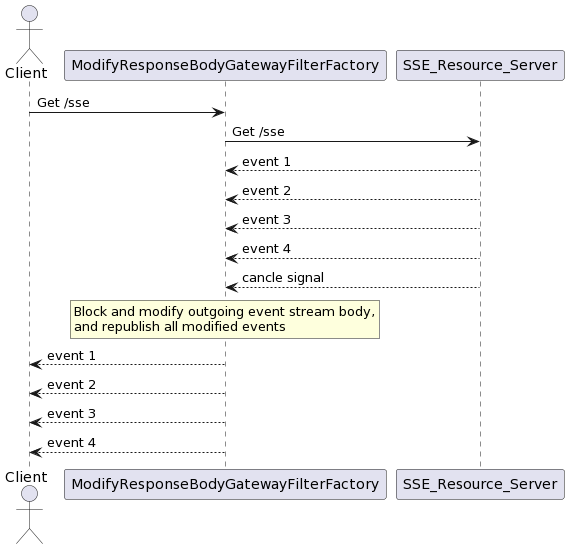
Expected behavior from ModifyResponseBodyGatewayFilterFactory: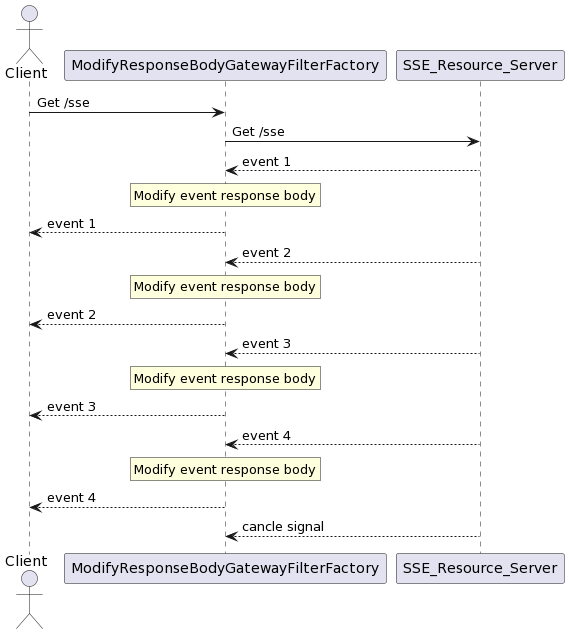
Fix
This PR contains fix for this issue and idea is to handle
writeAndFlushWith
method differently for stream mime type, aswriteAndFlushWith
being called by writer only when response content type is of Stream media type.Please review this PR, if looks ok please approve it.
Sample
Below test should fail with
DataBufferLimitException
, which means thatModifyResponseBodyGatewayFilterFactory
block all outgoing SSE events, until cancel signal received from publisher, and republish all events after modifying response body, as all events are in memory, which exceed the current buffer limitspring.codec.max-in-memory-size=40
-Adding test class -
SSE test controller -