Closed spreagtha closed 5 years ago
After reading SubContainers.md, it appears this is expected behaviour as the passed parameters are injected into the sub-containers installer, and not directly into the facade.
Per SubContainers.md
One important difference with creating a Sub-Container using a factory, is that the parameters you supply to the factory are not necessarily forwarded to the facade class. In this example, the parameter is a float value for speed, which we want to forward to the ShipInputHandler class instead. That is why these parameters are always forwarded to an installer for the sub-container, so that you can decide for yourself at install time what to do with the parameter. Another reason for this is that in some cases the parameter might be used to choose different bindings.
Hello,
When attempting to use PlaceholderFactory Create method with an extra parameter, the parameter is not being bound and fails validation with the following error:
The following code is a simplification of the situation in my game project's code which fails the validation check for a parameter that should be injected through the PlaceholderFactory Create method. The instantiated prefab is of the 'FactoryTest' type as in my example code, and will also spawn instances of it's own prefab recursively in certain scenarios (my use case was a 'state tree node' type which recursively spawns on certain nodes.
FactoryTestObjectInstaller.cs
FactoryTest.cs
TestInstaller.cs
FactoryUseTest.cs
The prefab 'FactoryTest' script is on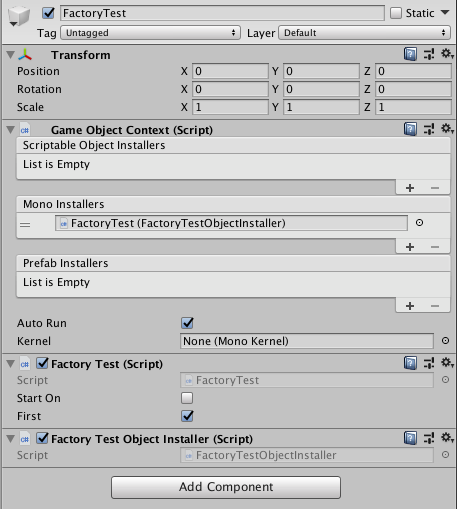
The 'root' FactoryTest spawning script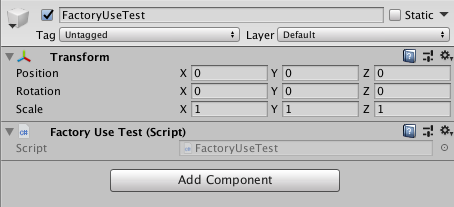
The SceneContext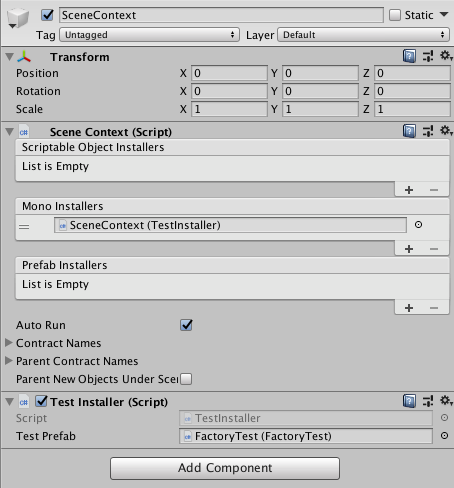
The Scene hierarchy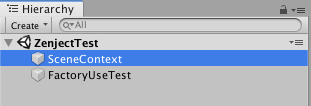