Closed yizhang-yiz closed 5 years ago
Thanks. @syclik---can you look this over and see if it's something we want to add? I'm afraid I don't know anything about stochastic diff eqs.
@syclik for some background there are two examples from https://discourse.mc-stan.org/t/ito-process-as-numerical-solution-of-stochastic-differential-equation/9192 demo the potential application. I'm working on the 3rd example.
The implementation used to run the examples in the above thread can be found at https://github.com/yizhang-yiz/math/blob/feature_sde_tamed_euler/stan/math/rev/mat/functor/ito_process_integrator.hpp
Three examples are included in the unit test with fixed Brownian motion path: geometric Brownian motion, stochastic SIR model, stochastic volatility model(Heston model): https://github.com/yizhang-yiz/math/blob/feature_sde_tamed_euler/test/unit/math/rev/mat/functor/ito_process_test.cpp
Thanks. Looks like the Discourse thread is picking up steam. I'll comment there.
Actually, some things that could be clarified on this issue itself. In the function signature:
F1
and F2
?y0
, weiner_normal
, theta1
, and theta2
?theta1
and theta2
declared as real[]
instead of vector
?stan::math::var
and double
)Is the primary use of this construct for convenience or for performance?
My take: if we can get the design straightened out, I think it's fine adding it as a function. I think that's the highest priority and we should focus on that.
On the implementation side, we need to make sure it's maintainable and there are enough tests to catch errors. and to help with future debugging. If there are dependencies to other libraries, we'll need to determine if it's appropriate if it requires additional dependencies. But I don't see why we shouldn't include something like this if it's designed well enough; it doesn't have to be perfect and hold forever. But it shouldn't just be slapped together either.
@syclik I've updated signature to c++, as I was posing in stan signature.
Is the primary use of this construct for convenience or for performance?
Convenience.
Allow me to redact the previous answer. The current proposed scheme(Euler) is rather easy to do in Stan, which is why the simple answer is "convenience". On the other hand, Euler is of order 1/2 for strong approximation, and naturally we may want something with higher order and/or more stable(like what happened in ODE solvers) in the future, say Milstein schemes, therefore laying out first steps is more than convenience.
@yizhang-yiz: thanks for updating the top level comment. I don't think there's anything conceptually stopping adding a function like this. From a design perspective, at the issue level, I think we should walk through the function signature checking for:
And then at the implementation level, it'll be whether there's a sensible software architecture in place (if it needs to do anything tricky) and whether it can be maintained (just good coding practice + tests so we can move it later if need be).
@syclik:
whether the function signature looks natural: order of arguments
I'll leave it to you guys as it involves personal taste.
names of arguments
I was following ODE signature, except w
(a matrix of iid standard normals).
doc
Maybe citing the literature for the scheme?
expectation on the functors: do they throw? do they check for good arguments at all? is it on the integration function to figure it out?
Since F1
and F2
are user-defined, I think it depends on the user. But we do want to check their returns to make sure consistent size.
From implementation level, the design is to break the functionality into stepper
and ito_process
. stepper
maps to a specific integration scheme(Euler in this case, but likely more in the future) that outputs result at step i+1
given that in step i
. ito_process
uses stepper
and given initial condition and wiener process to calculate the entire trajectory. One may be tempted to adopt boost odeint framework but IMO for the simple explicit scheme here it's an overkill.
I've also added some general background for SDE on discourse, shooting for common denominator of the audience.
I'm closing this as indicated in discourse discussion(https://discourse.mc-stan.org/t/ito-process-as-numerical-solution-of-stochastic-differential-equation/9192/36?u=yizhang) the way Stan samples doesn't support the scheme to be implemented using the proposed function. Further investigation required to support SDE inference through a different approach(State space model, for example).
Description
ito process as numerical solution of stochastic differential equation, solved by potentially various of schemes. The easiest ones are Euler-Maruyama and its variants.
Example
The function generating ito process could be like this
Expected Output
An
n x q
matrix that contains the simulated ito process, withn
being the system size(see above) andq
being the time steps for the process. This matrix can be param or data, depending on the input types of initial conditiony0
, Wiener process step normalsw
, and functor parameterstheta1
&theta2
.An example is the stochastic SIR model https://www.sciencedirect.com/science/article/pii/S0378437105001093, where the solution gives an Ito process of each compartment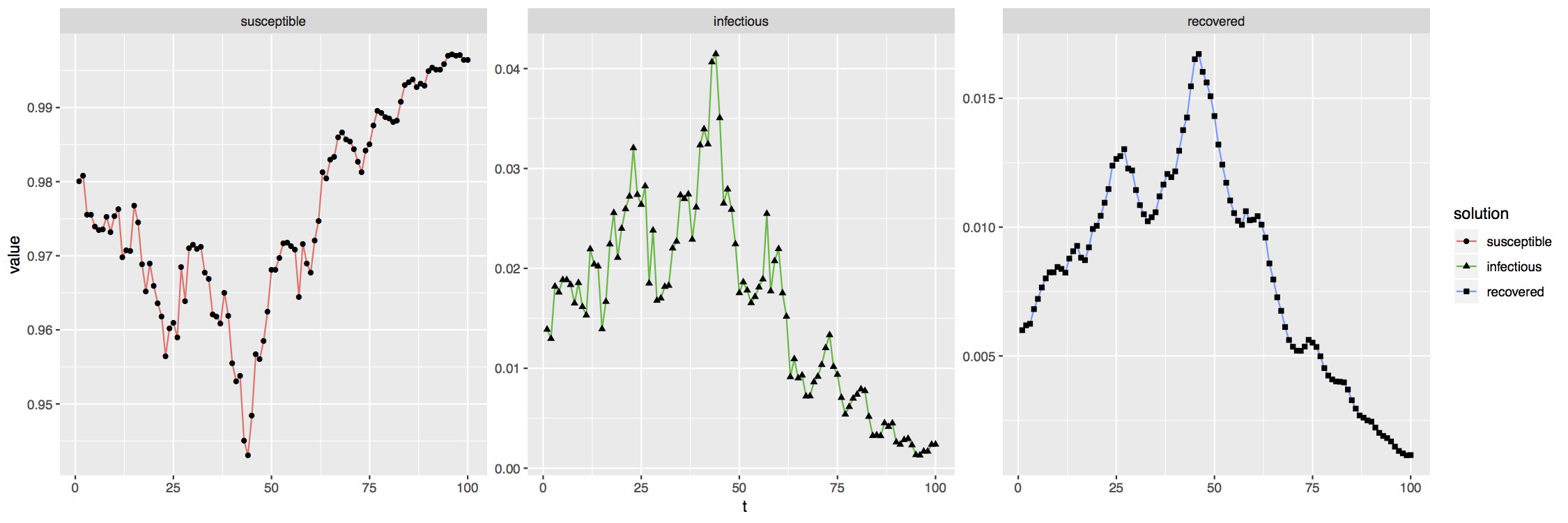
Current Version:
v2.19.1