Closed msayubi76 closed 1 year ago
Please share the structure of all three tables.
I jsut updated the question. Please have a look. @staudenmeir
Is groups.assigned_user_id
connected to users.assigned_user_id
or users.id
?
Is
groups.assigned_user_id
connected tousers.assigned_user_id
orusers.id
?
@staudenmeir it's connected with users.id
Use the eloquent-has-many-deep package:
class User extends Authenticatable implements MustVerifyEmail
{
use \Staudenmeir\EloquentHasManyDeep\HasRelationships;
public function companies()
{
return $this->hasManyDeep(
User::class,
['group_user', Group::class],
[null, null, 'id'],
[null, null, 'assigned_user_id']
);
}
}
Use this relationship to get unique users:
class User extends Authenticatable implements MustVerifyEmail
{
use \Staudenmeir\EloquentHasManyDeep\HasRelationships;
public function companies()
{
return $this->hasManyDeep(
User::class,
['group_user', Group::class],
[null, null, 'id'],
[null, null, 'assigned_user_id']
)->distinct();
}
}
Thanks @staudenmeir a little close. I tried and results are empty. In fact records are present in DB. This was the query after distinct
relation.
SELECT DISTINCT `users`.*,
`group_user`.`user_id` AS `laravel_through_key`
FROM `users`
INNER JOIN `groups`
ON `groups`.`assigned_user_id` = `users`.`id`
INNER JOIN `group_user`
ON `group_user`.`group_id` = `groups`.`id`
WHERE `group_user`.`user_id` = 43
But I need
SELECT DISTINCT `users`.*,
`group_user`.`user_id` AS `laravel_through_key`
FROM `users`
INNER JOIN `groups`
ON `groups`.`assigned_user_id` = `users`.`id`
INNER JOIN `group_user`
ON `group_user`.`group_id` = `groups`.`id`
WHERE `groups`.`assigned_user_id` = 43
Could you please where should I make changes above mentioned distinct
relation.
.
SELECT DISTINCT
users
.*,group_user
.user_id
ASlaravel_through_key
FROMusers
INNER JOINgroups
ONgroups
.assigned_user_id
=users
.id
INNER JOINgroup_user
ONgroup_user
.group_id
=groups
.id
WHEREgroups
.assigned_user_id
= 43
Did you run this query in your database?
Yes and get the accurate results that are required but I don't know how can I make changes in relation
I asked because the changed query no longer has the structure of a "normal" relationship query:
Deep relationship queries are a chain of join clauses where every join table is used in two join/where constraints ("on both sides"). In your query however, group_user
is only used once which doesn't fit into the whole structure of relationship queries.
Can you provide a small dataset and the expected result for me to reproduce to test both queries?
@staudenmeir I already shared all required tables and structure of data that is required. What else you need?. I'll provide you.
@staudenmeir I try to capture everything in single image. Hopefully this image will help you to understand my problem.
I just created a public repository for fresh new project. please have a look on it. Repository Link
@staudenmeir bro I'm still stuck in it. Would you please help ?
How can I reproduce the issue with your repository? I saw that the relationships are generated randomly. How do I know what the expected result is?
How can I reproduce the issue with your repository? I saw that the relationships are generated randomly. How do I know what the expected result is?
You can clone this project on your PC. I want to fetch all unique users added to all groups of login users. I've mentioned required results in this issue as well.
How can I reproduce the issue with your repository? I saw that the relationships are generated randomly. How do I know what the expected result is?
Actually I added all my of code to public repository so that you can understand it clearly. Let me know if you didn't get anything.
Login user can create multiple groups and add multiple user to each group. I want to display all unique users added to all groups. So far I tried Belongs to Trhough and Eloquent has many deep but unable to resolve my issue. Anyone help
User Model
Group Model
GroupUser Model for intermediate table
Fetch myCompanies
Exception Illuminate\Database\QueryException SQLSTATE[42S22]: Column not found: 1054 Unknown column 'group_user.id' in 'where clause' (SQL: select
users
.* fromusers
inner joingroups
ongroups
.user_id
=users
.id
inner joingroup_user
ongroup_user
.assigned_user_id
=groups
.id
wheregroup_user
.id
is null limit 1)Users Table Structure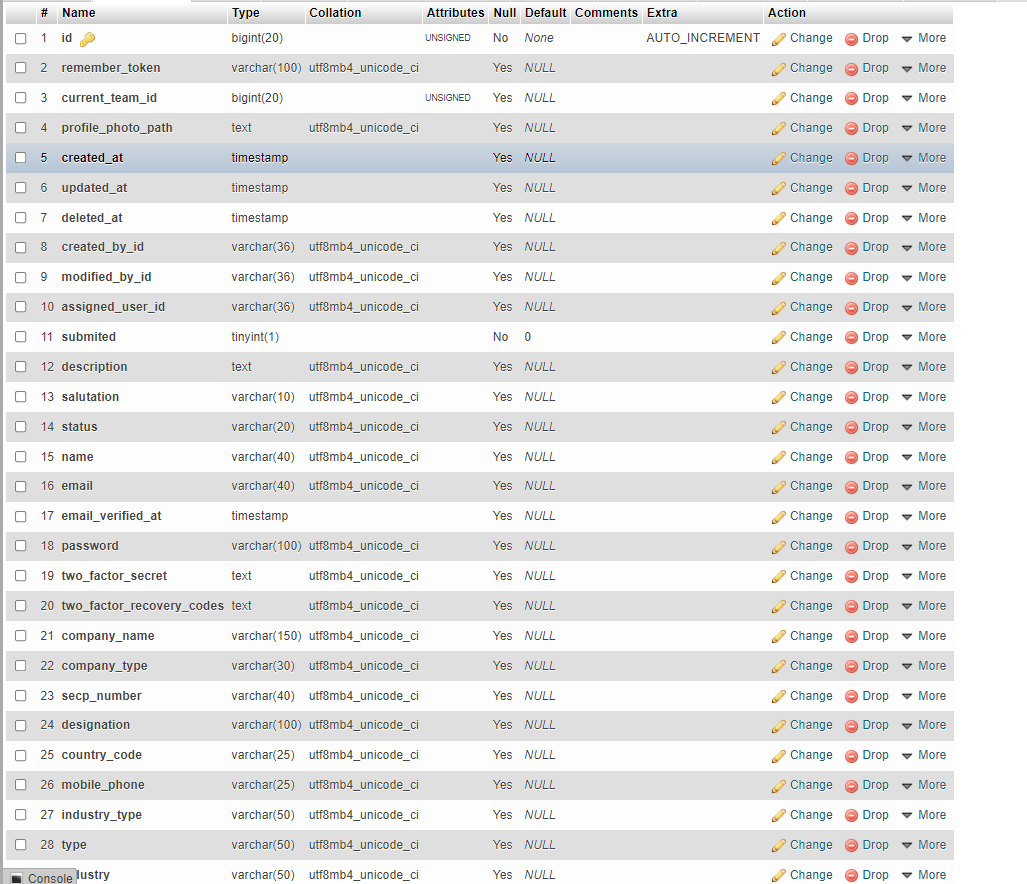
Groups Table Structure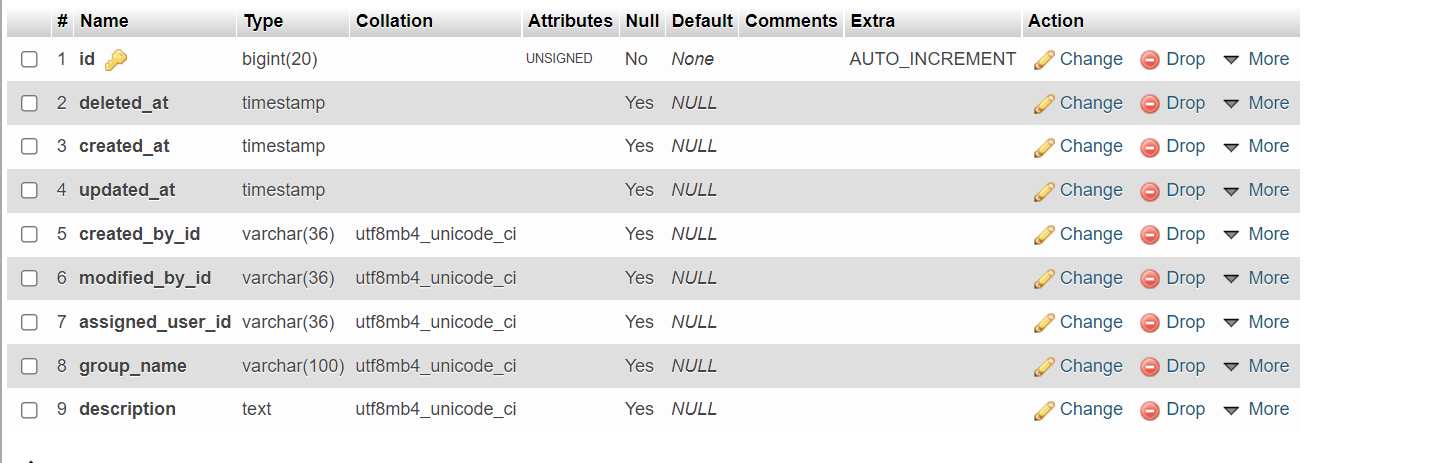
GroupUser Table Structure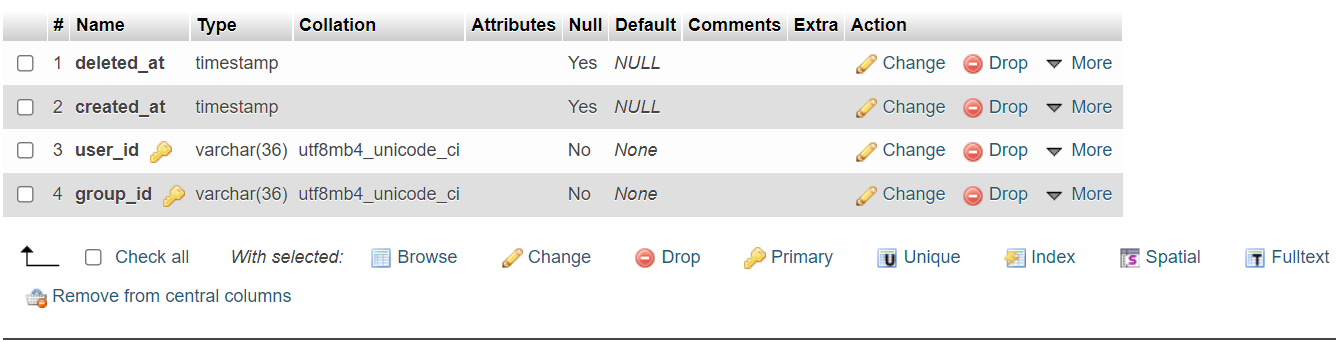