Closed ediaz2 closed 2 years ago
using index would be brittle :thinking: maybe a custom filter function added here https://github.com/svitejs/vite-plugin-qrcode/blob/ed2f4e0d1a7a3fc6060ab3f4df8d0ffa90d51b70/packages/vite-plugin-qrcode/src/index.ts#L64
maybe we should also filter on vite server address if it wasn't started with 0.0.0.0
The local network appears when the server.host
has the value 0.0.0.0
or true
;
If it is filtered, these 2 cases should be taken into account.
I'm going to review the vite
documentation more, I use this plugin daily to review the mobile view.
Thanks for your time.
I think it makes sense to introduce a filter function too, something like:
// vite.config.js
import { qrcode } from 'vite-plugin-qrcode';
export default defineConfig({
plugins: [
qrcode({
filter: (url, i) => i === 0 // or match by url incase index changes
})
]
});
There's now a filter
option that you can use to filter for a specific IP.
Thanks 😊
Describe the problem
It shows the qr of all the networks, to access local from the mobile I have to scroll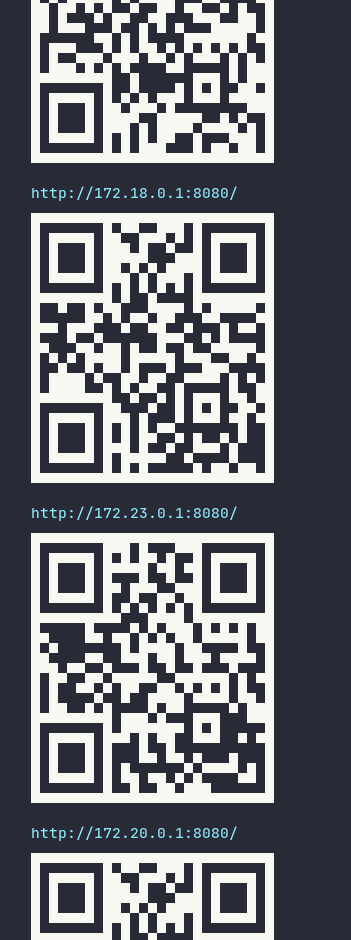
Describe the proposed solution
Pass an option as a parameter indicating the key to show optionally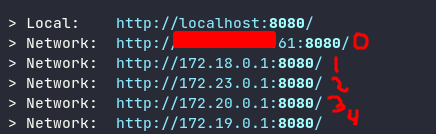
Alternatives considered
Use a
firstNetwork : boolean
parameter, so that only the first is displayed, although I don't know if they all get the same orderImportance
would make my life easier