Open kxiong22 opened 3 years ago
Hi,
This seems to be a pretty non-trivial example. If possible, could you please start from empty, then gradually introduce the ray marching functionality piece by piece to see which part results in the difference? Thanks
Describe the bug I am trying to write a differentiable raymarching function in Taichi, but the gradient of the loss wrt to the volume that Taichi computes seems to be off by some rotation + translation compared to the same gradient computed with equivalent raymarching code in PyTorch.
To Reproduce Below is my code. The forward() method in RaymarchFunction works as I want it to, but the backward() method does not and grad_input_template is wrong. If it helps, this code is basically just a combination of these two examples: https://github.com/yuanming-hu/difftaichi/blob/master/examples/volume_renderer.py, https://github.com/taichi-dev/taichi/blob/master/tests/python/test_torch_ad.py
Here is the equivalent code in PyTorch:
Log/Screenshots Here is a picture of one slice of the volume that is the difference between the gradient of the loss wrt the volume in PyTorch and Taichi (it would be entirely gray if the gradients were equal).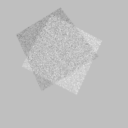
Additional comments I can definitely clarify more if this was not clear. Thanks so much!