Open graham-jessup opened 3 years ago
You should decouple the step generation from the communication. What happens is the motor very briefly stops when the MCU executes everything else in the loop and that throws off the readings. You can use an interrupt like in the original example.
The communication error occurs even when not driving the motor, the serial monitor screenshot above is while no steps are being generated. Normal behavior for sg_result would be a constant value (799 in this case), I think, but it's still being replaced with zeros intermittently.
I figured out the issue: The Teensy 4.1 runs SPI communication too fast for the TMC2130, and it results in frequent failed communications where the TMC2130 returns "0" for the stallguard value. Specifically, the chip select (CS) pin is switched too fast between SPI datagrams.
Solution (using software SPI):
In "TMC2130Stepper.cpp" I added a delayMicroseconds(1); line to the switchCSPin function (line 63)
void TMC2130Stepper::switchCSpin(bool state) {
digitalWrite(_pinCS, state);
delayMicroseconds(1); //GrahamJ Edit - slows down CS signal
<--- Added delay here
}
I also experimented with adding delays to the SW_SPI.cpp file to slow down the SPI clock frequency, but that ended up not being necessary.
I have not tried hardware SPI with this fix, I don't know if it uses the same switchCSPin function.
EDIT: This fix also works for hardware SPI on the Teensy 4.1.
I'm using a variant of the "Stallguard" example to tune the stallguard settings of my TMC 2130 SPI driver (from BigTreeTech), but I am having an SPI issue where the value for drv_status.sg_result returns 0 about half the time. SPI communication appears to be working for the most part, I can intialize the driver and run the stepper, but the real-time monitoring of the stallguard value is not very useful with all the zeros interspersed.
Am I missing a larger compatibility issue between the TMCStepper library and the Teensy 4.1?
What could be causing the SPI communication to intermittently fail?
Hardware: Teensy 4.1 BigtreeTech TMC2130 V3.0 SPI 24V power supply Nema 17 Stepper
Here's an example of what I'm seeing in the serial monitor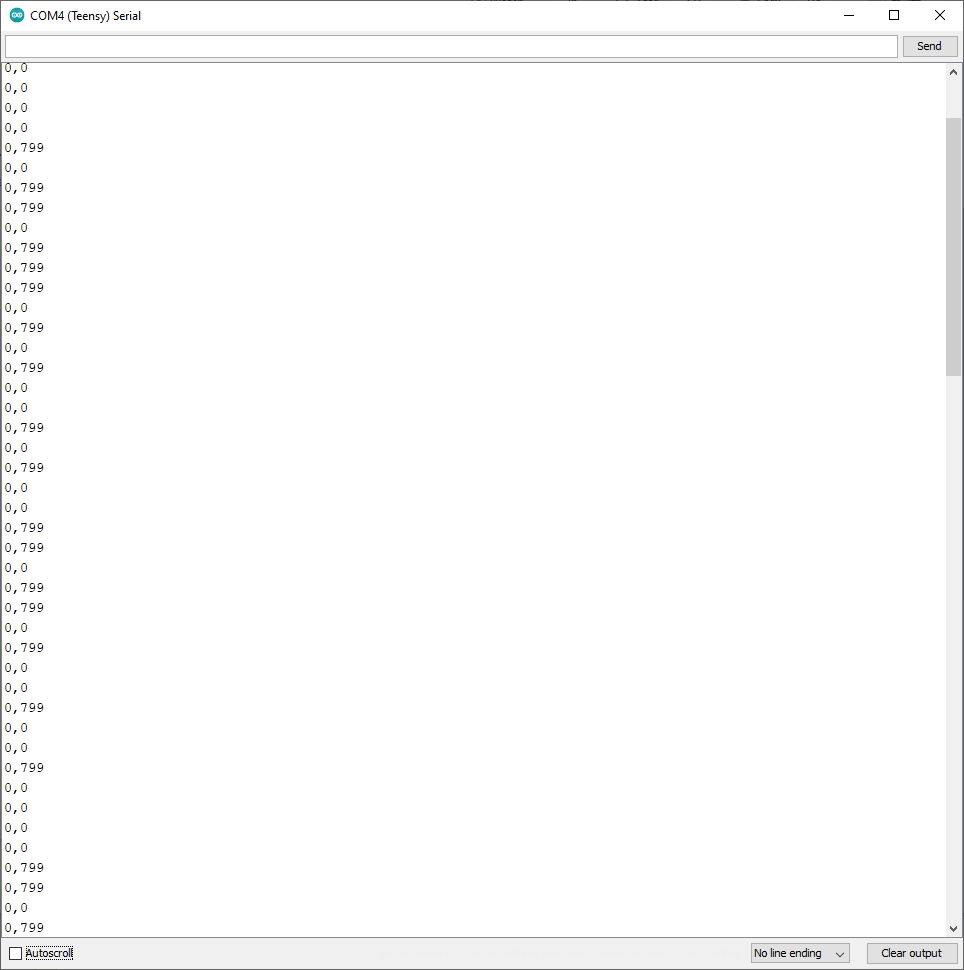
This is what I'm seeing with a logic analyzer reading the SPI communication when the drv_status.sg_result returns zero.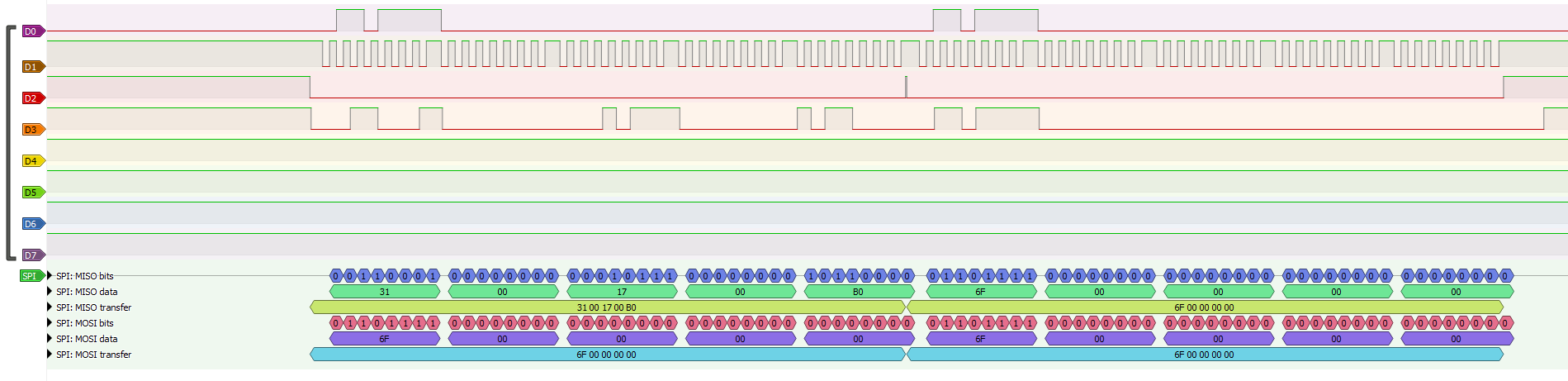
Here's a photo of my setup: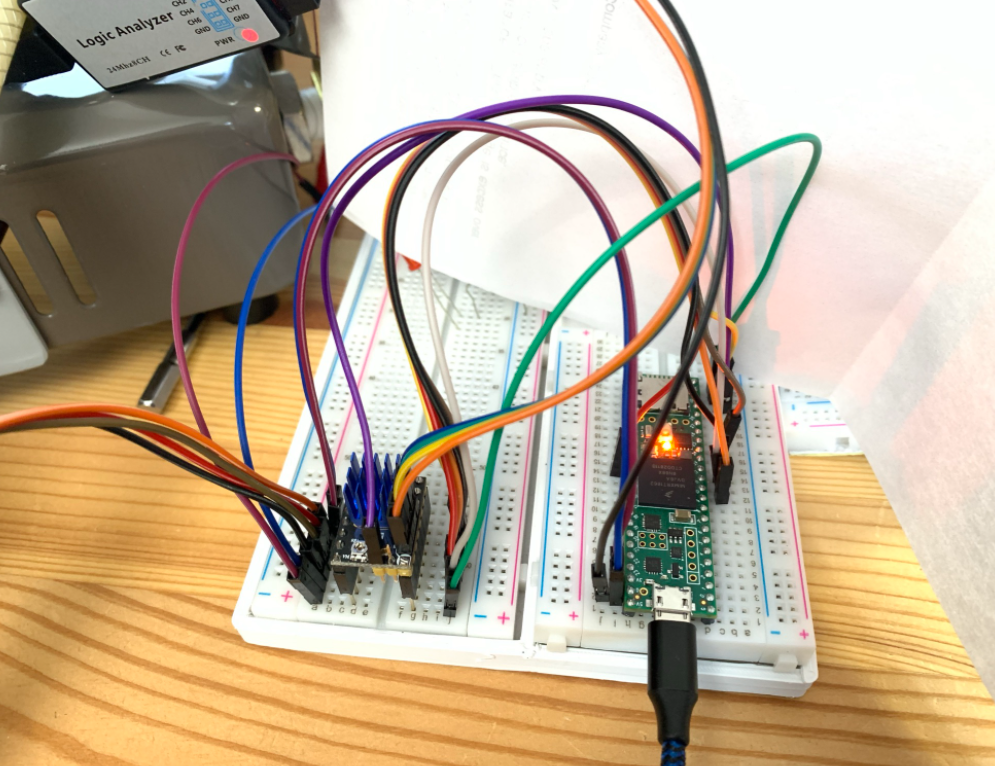
And finally the code: