Closed JamesBrightman-pp closed 1 year ago
Because each hook call is it's own instance e.g. calling the hook outside the component will not affect the component's hook, that would create wild UI behaviour for users. Hooks are meant for sharing logic across codebases. Maybe take a look at this ā https://beta.reactjs.org/learn#using-hooks
I have written a simple component and test file which aims to test the hook and the UI. It uses the
useCounter
hook example from the docs.TextComp.tsx
TestComp.spec.tsx
The test file has 4 tests.
All of these tests pass except 4. Where I expect to see "COUNT: 1" I actually see "COUNT: 0". Why is this?
Error message dump: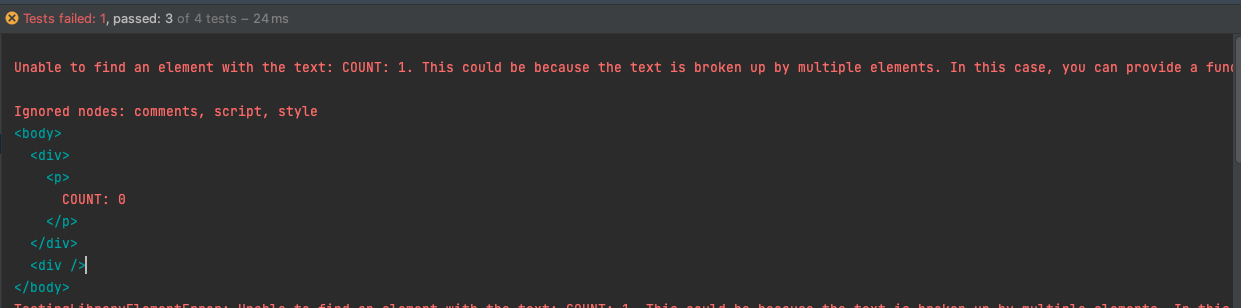
Is this due to a race condition / async operation? Am I using the library completely wrong? I want to be able to test that the hook functionality properly updates the UI, but I am seeing what looks like an out of date Ui version. Thanks for the help.