Closed MistrKernnunos closed 6 years ago
The first problem seems to be the connections of the encoder: common goes to ground, and you don't need external pull-down resistors (you'd use pull-up, if any).
The problem with your MIDI messages is that you're not using the correct baud rate. The default baud rate of the MIDI Controller library is 31250 baud (i.e. the official MIDI hardware baud rate). It is meant to be used with HIDUINO, as explained in the ReadMe. If you want to use it with hairless, you have to explicitly instantiate a HairlessMIDI_Interface.
Documentation
For example:
#define USE_ROTARY_ENCODER
#include <MIDI_Controller.h>
HairlessMIDI_Interface myHairlessMidi;
// ...
The default baud rate for Hairless is 115200 baud, you can edit this in Settings.h
, or you can instantiate a HardwareSerialMIDI_Interface:
HardwareSerialMIDI_Interface myMidi(Serial, baud);
Thanks, it worked. but now, when i twist to one side, the value changes only by one to 63. And when i twist to other side it changes to 65.
That depends on the representation you use. https://github.com/tttapa/MIDI_controller/wiki/Library-documentation#rotaryencoder
But it stayes in that value until i twist on the other side, and then it changes by 1 and stayes at that value
I don't know what you mean. Please post some of the output you're seeing.
Yes, that's perfectly fine. What were you expecting to see?
Rotary encoders produce incremental output, Light Room should be able to handle that.
i was expetcting numbers between 1 and 127
1 just means '+1' and 127 means '-1'. A rotary encoder doesn't have an absolute position.
so i can't have it set on 20?
What do you mean? You have it set to controller # 20 right now. This controller has a value, for example, the brightness in LR (I have no idea if this is realistic, I've never used LR). If the brightness value in LR is at 200, for example, and you turn the encoder two clicks counterclockwise, the MIDI controller will send 2 messages containing the number 127 (= -1 in 7bit-two's-complement), so LR decreases the brightness by 2·(-1) → 198.
You have no control over the absolute position of the encoder (at least not directly).
Have you tried mapping it in LR? What were the results?
yes, now it works. I only neede to chamge some setting in lr.
And how can i setup 16 chanel multiplexer with buttons? It is 74HC154N. Thanks for your advices
Yes, you can setup a 16 channel multiplexer to work with the library. But the 74HC154N is not a multiplexer, it's a demultiplexer.
i figured it out. Now I am using 2 m74hc4051b1 in this configuration.
and whe i press some button conected to the first one it activates all 8 buttons conected to it, but when i press some on the second it activates only the one.
Here is my code
HairlessMIDI_Interface myHairlessMidi;
const uint8_t Channel = 1; // MIDI channel 1 const uint8_t Controller = 0x14; // MIDI Control Change controller number const int speedMultiply = 1; // No change in speed of the encoder (number of steps is multiplied by 1)
// Create a new instance of the class 'RotaryEncoder', called 'enc', on pin 2 and 3, controller number 0x14, on channel1, // no change in speed (speed is multiplied by 1), it's used as a jog wheel, and the sign mode is set to two's complement. RotaryEncoder encoder(2, 3, Controller, Channel, speedMultiply, 4, TWOS_COMPLEMENT); DigitalCC button(6, 25, 1);
void setup() {}
void loop() { // Refresh the encoder (check whether the position has changed since last time, if so, send the difference over MIDI) MIDI_Controller.refresh(); }
The code you posted doesn't declare any multiplexers. Please look at the wiki and the examples.
I apologize, i posted bad code. AnalogMultiplex multiplexers[] = {
{A0, {11,10,9}}, { A1, {11,10,9}}, }; HairlessMIDI_Interface myHairlessMidi;
// Create 8 new instances of the class 'Analog', on the 8 pins of the multiplexer, // that send MIDI messages with controller 7 (channel volume) on channels 1 - 8 DigitalCC butons[] = { {multiplexers[0].pin(0), 0x1, 1}, {multiplexers[0].pin(1), 0x2, 1}, {multiplexers[0].pin(2), 0x3, 1}, {multiplexers[0].pin(3), 0x4, 1}, {multiplexers[0].pin(4), 0x5, 1}, {multiplexers[0].pin(5), 0x6, 1}, {multiplexers[0].pin(6), 0x7, 1}, {multiplexers[0].pin(7), 0x8, 1}, {multiplexers[1].pin(1), 0x9, 1},
That code doesn't compile. Please post your complete code, and surround it with tripple backticks (```) so it is readable.
Please read this: https://help.github.com/articles/creating-and-highlighting-code-blocks/
It wasnt problem with code. I have just conected wrong line on bread board. Now is every thing working just fine. Thanks for your Advices.
Но он остается в этом значении до тех пор, пока я не повернусь с другой стороны, а затем он изменится на 1 и останется на это значение
MistrKernnunos, Hi, I also make the light room controller and also faced the problem of a rotary encoder. Can you describe in detail how you managed to solve the problem?
What do you mean? You have it set to controller # 20 right now. This controller has a value, for example, the brightness in LR (I have no idea if this is realistic, I've never used LR). If the brightness value in LR is at 200, for example, and you turn the encoder two clicks counterclockwise, the MIDI controller will send 2 messages containing the number 127 (= -1 in 7bit-two's-complement), so LR decreases the brightness by 2·(-1) → 198.
You have no control over the absolute position of the encoder (at least not directly).
Have you tried mapping it in LR? What were the results?
please tell in detail how to implement it?
Description of the problem or question
When i am using rotary encoders. They dont send Control Change, but some random stuff like timing clocks etc.
Steps to reproduce the problem
I used the example with RotaryEncoder
Hardware
Arduino Uno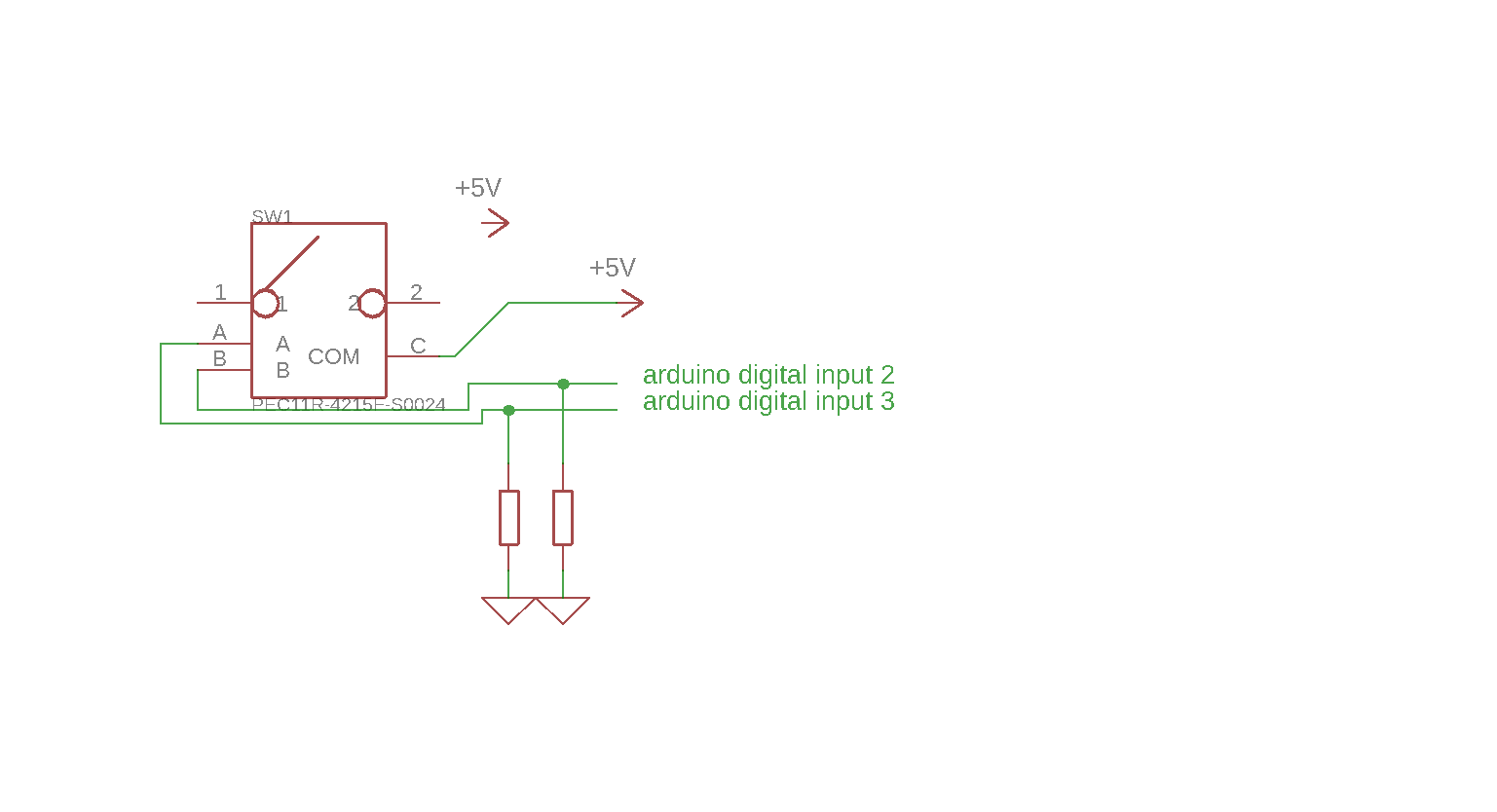
Software versions:
MIDI Controller library> latest Arduino IDE: ? 1.8.4 ?
Operating System: ? Windows Operating System version 10
Settings in the IDE
Full code
Steps taken to try to diagnose or solve the problem
? Tried the MIDI debug mode, used a MIDI monitor, ... ?
The goal of your project and aditional information
reporting midi control change values into pc and the using them in light room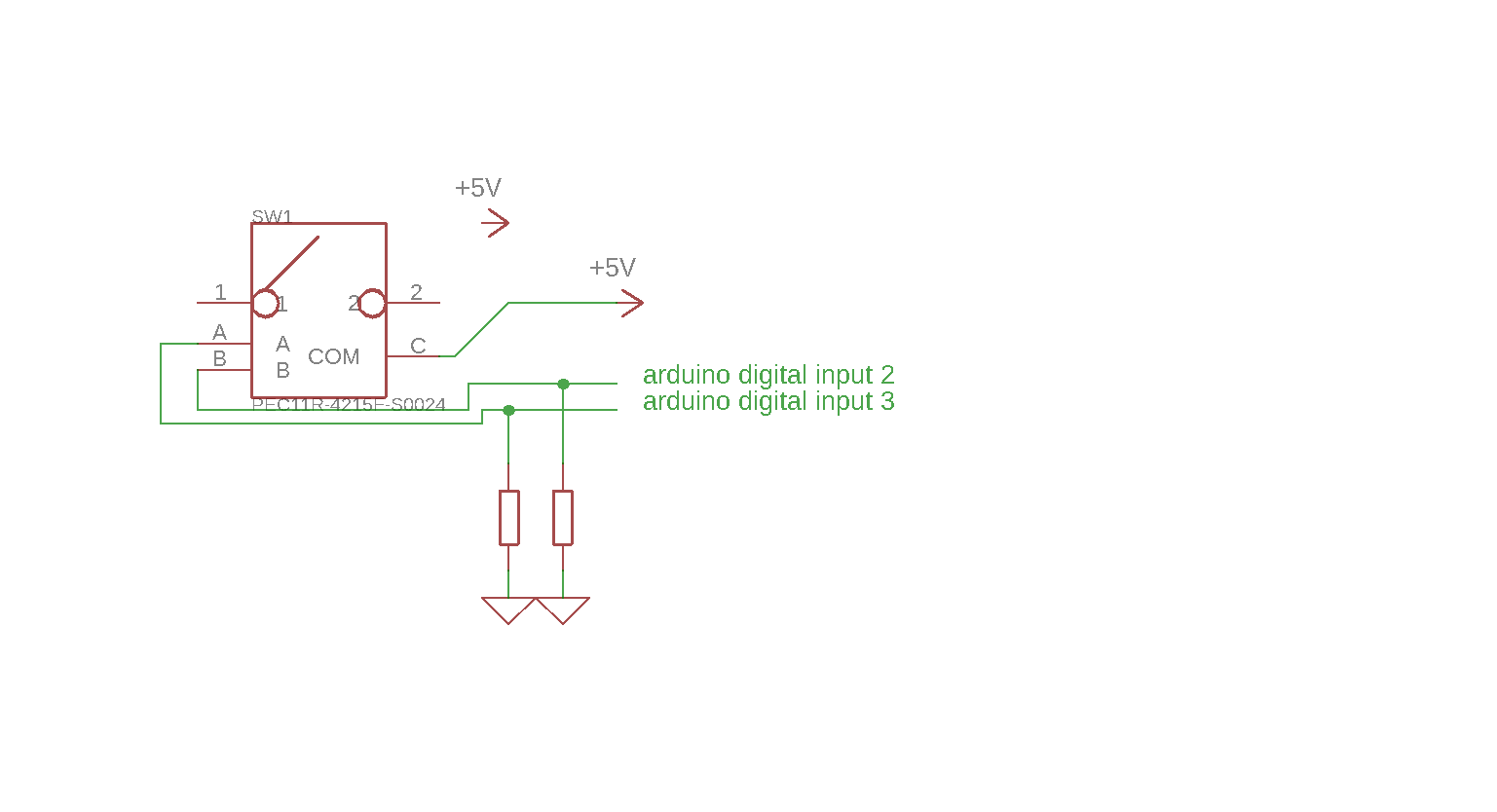