Closed saran13raj closed 2 years ago
@saran13raj
import React, { useContext } from "react";
import MDEditor, {
commands,
ICommand,
EditorContext
} from "@uiw/react-md-editor";
const Button = () => {
const { preview, dispatch } = useContext(EditorContext);
const click = () => {
dispatch!({
preview: preview === "edit" ? "preview" : "edit"
});
};
if (preview === "edit") {
return (
<svg width="12" height="12" viewBox="0 0 520 520" onClick={click}>
<polygon
fill="currentColor"
points="0 71.293 0 122 319 122 319 397 0 397 0 449.707 372 449.413 372 71.293"
/>
<polygon
fill="currentColor"
points="429 71.293 520 71.293 520 122 481 123 481 396 520 396 520 449.707 429 449.413"
/>
</svg>
);
}
return (
<svg width="12" height="12" viewBox="0 0 520 520" onClick={click}>
<polygon
fill="currentColor"
points="0 71.293 0 122 38.023 123 38.023 398 0 397 0 449.707 91.023 450.413 91.023 72.293"
/>
<polygon
fill="currentColor"
points="148.023 72.293 520 71.293 520 122 200.023 124 200.023 397 520 396 520 449.707 148.023 450.413"
/>
</svg>
);
};
const codePreview: ICommand = {
name: "preview",
keyCommand: "preview",
value: "preview",
icon: <Button />
};
export default function App() {
const [value, setValue] = React.useState("**Hello world!!!**");
return (
<div className="container">
<div>The system automatically sets the theme</div>
<MDEditor
value={value}
preview="edit"
extraCommands={[codePreview, commands.fullscreen]}
onChange={(val) => {
setValue(val!);
}}
/>
</div>
);
}
Thanks a lot. @jaywcjlove
This is great! but how can you do this in next.js?
When you import the context like this
import { EditorContext } from "@uiw/react-md-editor/lib/Context";
It loses the actual value
https://codesandbox.io/s/react-md-editor-296-forked-33p4i2?file=/fullscreencmd.js
How can I show edit and preview by using a single icon as toggle without using 2 different icons in toolbar? I don't need these 3 icons: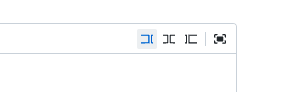
Instead, show the edit and preview as a toggle: