Closed ahmed-adly-khalil closed 3 years ago
I found a solution for anyone who will face this in the future:
import { useEffect } from 'react';
export default function GlobeComponent() {
useEffect(async () => {
const ThreeGlobe = (await import('three-globe')).default
const myGlobe = new ThreeGlobe()
console.log(myGlobe);
}, []);
return (
<div> </div>
)
};
Hello, I'm so sorry to open this, however I have spent the past 8 hours trying to get it done with next JS with no luck.
I have the following great example fully working in an HTML file
Now i need to move this to next JS, and I want to use
three-globe
notreact-globe.gl
as this one gives me the needed control to do customization.Here is the code for my next JS component.
and it gives this error whatever i try to do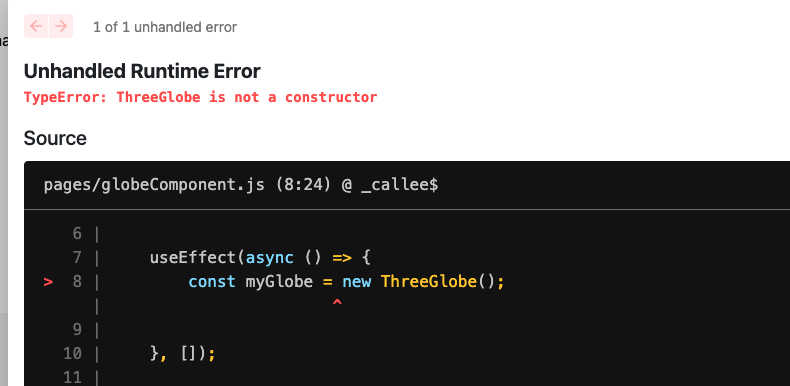
I think it might be something with how
kapsule-class.js
is implemented but i can't wrap my head around it.Thank you so much.