Closed ryanbeau closed 1 year ago
Oh my... I just discovered that this works in Go:
// Update the intermediate texture with the contents of the RGBA buffer.
pixels := argbbuffer.Pixels()
tpixels, _, err := texture.Lock(nil)
if err != nil {
panic("oops")
}
for i := 0; i < len(tpixels); i++ {
tpixels[i] = pixels[i]
}
texture.Unlock()
And it's the same as this:
// Update the intermediate texture with the contents of the RGBA buffer.
SDL_UpdateTexture(texture, NULL, argbbuffer->pixels, argbbuffer->pitch);
I suppose this thread could be closed, unless the above behavior is unexpected considering it works in C. Thanks
Hi @ryanbeau, it seems to be caused by the pixel data address being the address of the pixels slice &pixels
rather than its data &pixels[0]
when passed to texture.Update()
function.
That makes sense & works. Thanks
Go version:
1.19
Go-SDL2 version:v0.4.33
SDL2 version:2.0.20
OS:Windows 10
&Ubuntu Ubuntu 22.04.1 LTS
(I tried both)In an attempt to copy SDL2 setup & functionality from: Chocolate-Doom - i_video.c
What it does: It attempts to keep the original resolution of Doom (320x200) and upscales it to fit the window or screen. It has a 320*200 screenBuffer Surface with a 256 color palette. The surface does a LowerBlit to an ARGB destination Surface. It then updates the low scale Texture from the pixels of the ARGB Surface, and then copies the low scale texture to the upscaled texture, and draws that to the screen.
I'm unsure if I've done something different or incorrectly. Most specifically I'm unsure about the unsafe.Pointer to the ARGB Pixels.
In C, the results are as expected: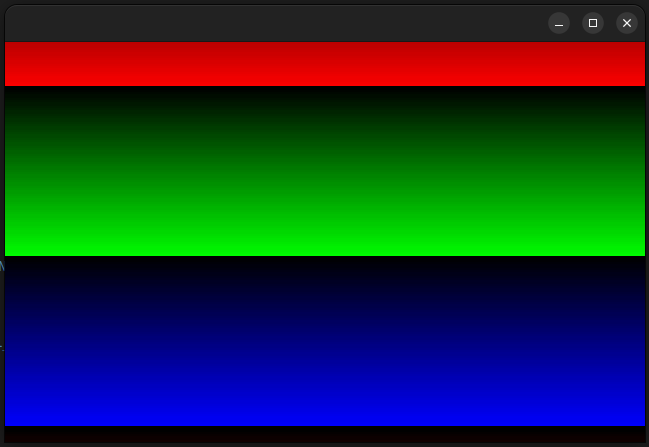
Expand to view C source
```c #includeIn Go, the results are quite random: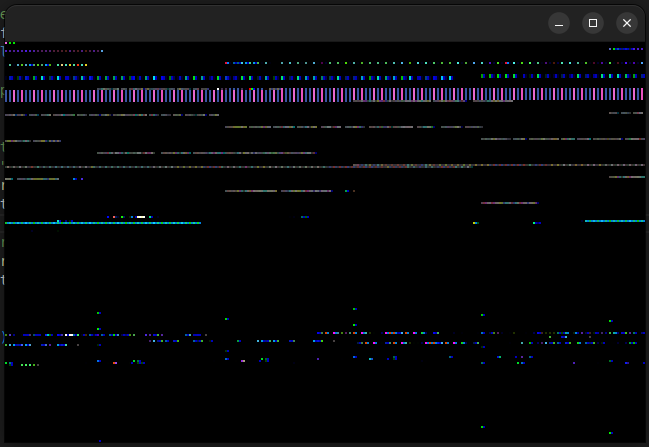
Thanks