Open thomassajot opened 2 years ago
I can manually find an appropriate scale but this is not automated:
long_domain = source['longitude_degs'].agg([min, max]).tolist()
lat_domain = source['latitude_degs'].agg([min, max]).tolist()
center = ((long_domain[0] + long_domain[1]) / 2, (lat_domain[0] + lat_domain[1]) / 2, )
points = alt.Chart(source).mark_line().encode(
longitude='longitude_degs:Q',
latitude='latitude_degs:Q',
order='index')
chart = (london + points).project(center=center, scale=80000)
display(chart)
This may be a better question for the vega-lite repository – Altair doesn't have access to the necessary information to auto-generate domains
See Vega Lite issue
@jakevdp is seems that a fit
option can be given to Vega-Lite. Is this feature available in Altair?
https://github.com/vega/vega-lite/issues/7448
This works for me on the development version of Altair:
import altair as alt
from vega_datasets import data
source = alt.topo_feature(data.world_110m.url, 'countries')
features = [
{
"geometry": {
"coordinates": [[[-3, 52], [4, 52], [4, 45], [-3, 45], [-3, 52]]],
"type": "Polygon"
},
"type": "Feature"
},
{
"geometry": {
"coordinates": [[[-3, 59], [4, 59], [4, 52], [-3, 52], [-3, 59]]],
"type": "Polygon"
},
"type": "Feature"
}
]
alt.Chart(source).mark_geoshape(clip=True).project(fit=features)
@thomassajot There is a new page in the documentation on the geoshape mark including how to use project(fit=...)
. Does this resolve your original inquiry or do you think there is something still missing?
When using Altair for plotting geographic objects, the visualization automatically chooses an appropriate projection. However if we overlay another object, I would like to rescale / crop the chart to have a better zoomed view
Example:
The above display returns the following image: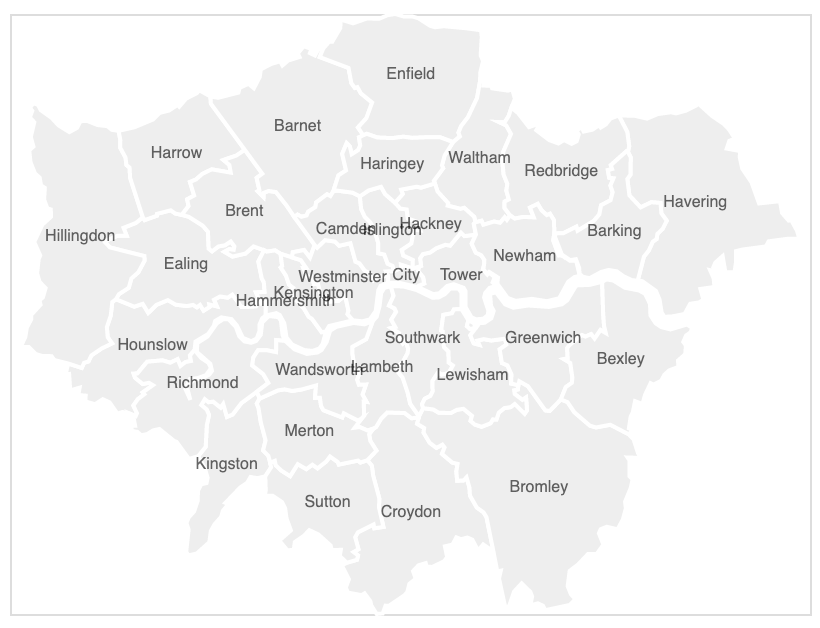
Where an appropriate projection has been chosen. If I add an additional object on the chart.
The projection chosen is based on the largest fitting projection. However I would like to "zoom" in on the smaller object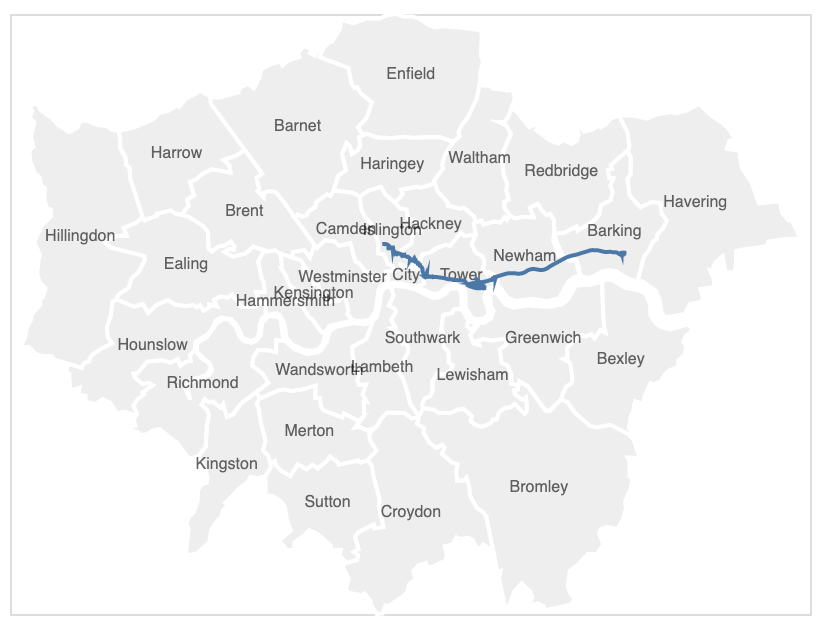
Something along the lines of:
But of course,
points.projection
is also undefined.Please follow these steps to make it more efficient to respond to your feature request.