Open vikramlc opened 4 years ago
Different ways of creating arrays:
Array.from acts like a toArray() in JAVA:
const listItems = document.querySelectorAll('li');
const arrayListItems = Array.from(listItems); // li, li, li
push(), pop(), unshift(), shift():
let fruits = ['Apple', 'Banana']
let newLength = fruits.push('Orange')
// ["Apple", "Banana", "Orange"]
let last = fruits.pop() // remove Orange (from the end)
// ["Apple", "Banana"]
let first = fruits.shift() // remove Apple from the front
// ["Banana"]
let newLength = fruits.unshift('Strawberry') // add to the front
// ["Strawberry", "Banana"]
Splice(), Slice(): splice() -> returns the list of
fruits.push('Mango')
// ["Strawberry", "Banana", "Mango"]
let pos = fruits.indexOf('Banana')
// 1
let removedItem = fruits.splice(pos, 1) // this is how to remove an item
// ["Strawberry", "Mango"]
let removedItem = fruits.splice(1, 0, 'Grape') // this is how to remove an item
// ["Strawberry", "Grape", "Mango"]
let shallowCopy = fruits.slice() // this is how to make a copy
// ["Strawberry", "Grape", "Mango"]
const fruits = ['apple', 'mango', 'grape', 'banana'];
undefined
fruits
(4) ["apple", "mango", "grape", "banana"]
const splicedFruit = fruits.splice(1, 0, 'berry');
undefined
splicedFruit
[]
fruits
(5) ["apple", "berry", "mango", "grape", "banana"]
const removedFruit = fruits.splice(1, 1);
undefined
removedFruit
["berry"]
fruits
(4) ["apple", "mango", "grape", "banana"]
concat():
const array1 = ['a', 'b', 'c'];
const array2 = ['d', 'e', 'f'];
const array3 = array1.concat(array2);
console.log(array3);
// expected output: Array ["a", "b", "c", "d", "e", "f"]
const num1 = [1, 2, 3];
const num2 = [4, 5, 6];
const num3 = [7, 8, 9];
const numbers = num1.concat(num2, num3);
console.log(numbers);
// results in [1, 2, 3, 4, 5, 6, 7, 8, 9]
const letters = ['a', 'b', 'c'];
const alphaNumeric = letters.concat(1, [2, 3]);
console.log(alphaNumeric);
// results in ['a', 'b', 'c', 1, 2, 3]
indexOf(), lastIndexOf():
fruits.push('Mango')
// ["Strawberry", "Banana", "Mango", "Banana"]
let pos = fruits.indexOf('Banana')
// 1
let pos1 = fruits.indexOf('Banana')
// 3
find(), findIndex():
const inventory = [
{name: 'apples', quantity: 2},
{name: 'bananas', quantity: 0},
{name: 'cherries', quantity: 5}
];
const cherries = inventory.find(fruit => {
return fruit.name === 'cherries';
});
const cherriesIndex = inventory.findIndex(fruit => {
return fruit.name === 'cherries';
});
cherries
> {name: "cherries", quantity: 5}
cherriesIndex
> 2
includes():
const array1 = [1, 2, 3];
console.log(array1.includes(2));
// expected output: true
const pets = ['cat', 'dog', 'bat'];
console.log(pets.includes('cat'));
// expected output: true
console.log(pets.includes('at'));
// expected output: false
ForEach:
const prices = [10.99, 2.99, 5.99, 8.99];
const tax = 0.19;
const taxAdjustedPrices = [];
// ForEach have access to the index and also we can take action on each element
prices.forEach((price, idx, prices) => {
const actualObj = { index: idx, taxAdjPrice: price * (1 + tax)};
taxAdjustedPrices.push(actualObj);
});
console.log(taxAdjustedPrices);
Map:
const prices = [10.99, 2.99, 5.99, 8.99];
const tax = 0.19;
// Map -> returns a completely new array, the original array is untouched
const taxAdjustedPrices = prices.map((price, idx, prices) => {
const actualObj = { index: idx, taxAdjPrice: price * (1 + tax)};
return actualObj;
});
console.log(prices, taxAdjustedPrices);
Sort:
// This converts elements in array in string and thenn sorts.
const naturalSortedPrices = prices.sort();
console.log('Natural Sorted Prices', naturalSortedPrices); // o/p: [10.99, 2.99, 5.99, 8.99]
// This sorts elements based on the condition in the sort method.
const sortedPrices = prices.sort((a, b) => {
if(a > b) {
return 1;
} else if(a < b) {
return -1;
} else {
return 0;
}
});
console.log('Sorted Prices', sortedPrices);
console.log('Reversed Prices', sortedPrices.reverse());
Filter:
const filteredPrices = prices.filter((price, idx, prices) => {
return price > 6;
});
console.log('Filtered Prices', filteredPrices);
Reduce:
// Reduce(): Accepts 2 parameters: 1st: function(), 2nd: initial value
const sum = prices.reduce((prevValue, curValue, curIndex, prices) => {
return prevValue + curValue;
}, 0);
console.log('Sum: ', sum);
split(), join():
const data = 'new york;10.99;2000';
undefined
const transformedData = data.split(';');
undefined
transformedData
(3) ["new york", "10.99", "2000"]
transformedData[1] = +transformedData[1];
10.99
transformedData
(3) ["new york", 10.99, "2000"]
const nameFragments = ['Max', 'Miller'];
undefined
nameFragments.join();
"Max,Miller"
nameFragments.join(' ');
"Max Miller"
Spread operator:
// Copy elements into new array
const nameFragments = ['Max', 'Miller'];
const copiedNameFragments = [...nameFragments];
nameFragments.push('Mr');
console.log(nameFragments, copiedNameFragments);
// Find minimum using spread operator
const numbers = [3,4,1,2,0];
const minValue = Math.min(...numbers);
console.log(minValue);
// Copying objects into new array copies the addresses of the objects
const personData = [{name: 'Jane', age: 30}, {name: 'James', age: 27}, {name: 'Carry', age: 28}];
const copiedPersonData = [...personData];
// Changing the value in the object here will change in both the original array and copied array
personData[0].age = 99;
console.log(personData, copiedPersonData);
// Copying a completely new array
const newCopiedPersonData = personData.map(person => ({
name: person.name,
age: person.age
}));
personData[0].age = 100;
console.log(personData, newCopiedPersonData);
Array Destructuring:
// Array destructuring
const nameData = ['James', 'Lannister', 'Mr'];
const [firstName, lastName] = nameData;
console.log(firstName, lastName); // James Lannister
// Using spread operator with Array Destructuring
const nameData = ['James', 'Lannister', 'Mr', 30];
const [firstName, lastName, ...otherInfo] = nameData;
console.log(firstName, lastName, otherInfo); // James Lannister ['Mr', 30]
Iterables and Array-like objects:
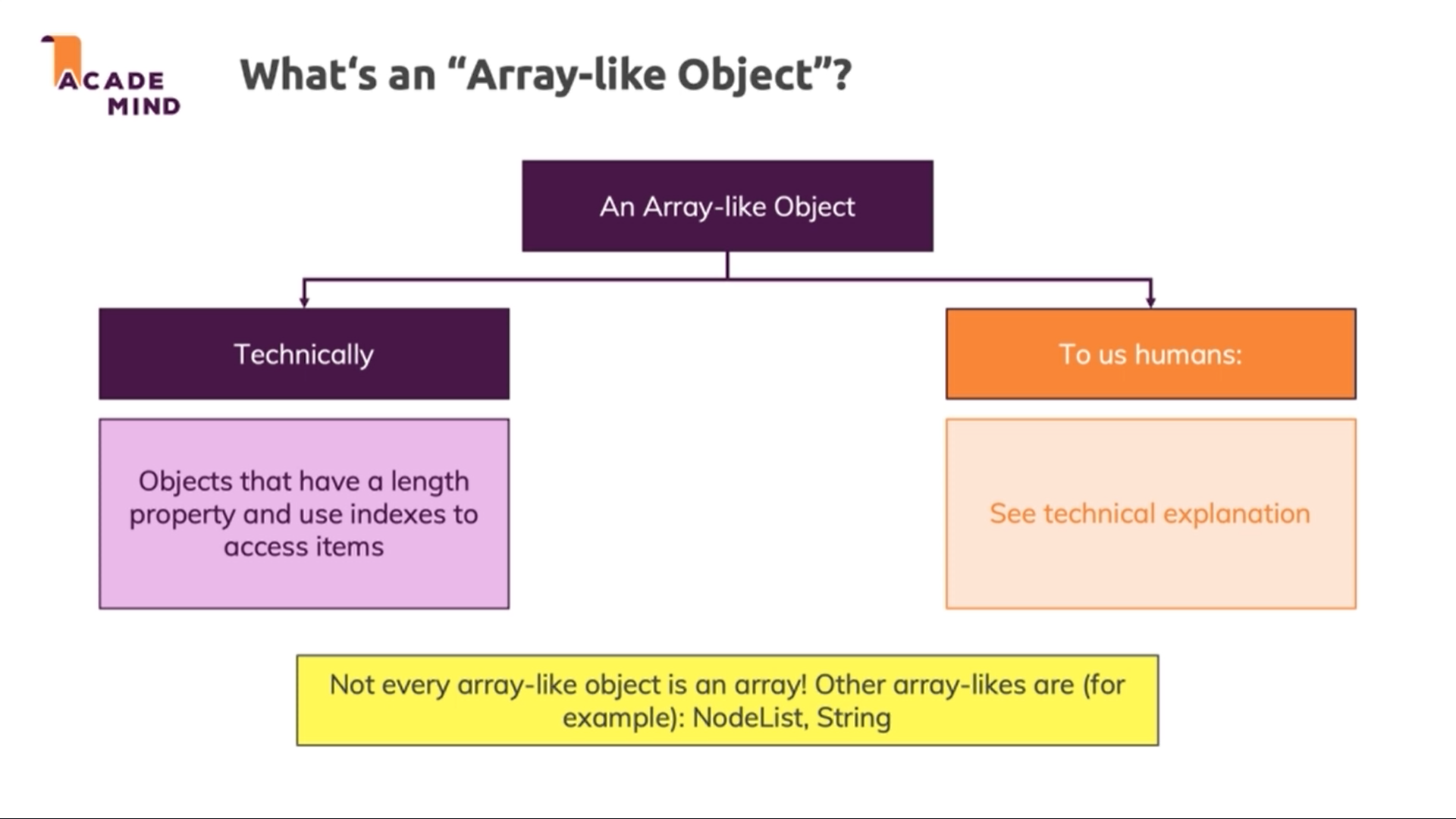