Open felixpalmer opened 1 year ago
https://github.com/visgl/deck.gl/pull/7569 provides an easier way to pass parameters to texture
I personally like the TextureArray approach, though I don't know how it can handle various pattern dimensions. I'm less worried about the WebGL2 requirement since it's supported in most mainstream browsers now.
Using color channels just seems so limited, I'm not sure it's worth the effort.
For the time being, I think we can really benefit from a write-up in the FillStyleExtension
documentation, similar to this section. There are several mitigations that we can offer before this issue is addressed in code:
textureParameters
to the layer to use mipmap and wrapping
Target Use Case
The FillStyleExtension hard codes the texture it uses to use
LINEAR
filtering, which means mipmaps are not used when rendering. When the view is zoomed out, artifacts appear in the form of Moire patterns as the sampling rate is too low to capture the texture. This can be easily seen in the example on the FillStyleExtension doc page.Changing the texture to use
LINEAR_MIPMAP_LINEAR
filtering helps and when the maps is zoomed out the pattern fades into a single color, as expected.However, doing so introduces another artifact which is harder to fix. Because the extension uses a texture atlas to support multiple patterns it cannot use
REPEAT
texture wrapping, and instead emulates this in the shader usingmod()
functions. These work fine with theLINEAR
filtering, but not withLINEAR_MIPMAP_LINEAR
because the incorrect mipmap is selected at the periodic boundary of themod()
function. This happens because the the mipmap level is selected based on the derivative of thetexCoords
variable and this shoots up every timemod
clips it from a value of e.g. 1.0 -> 0.0.To reproduce
Below is a test app which shows how the code can be patched to allow correct filtering, but only with a single texture. This cropped image should be used: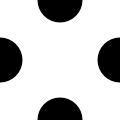
Proposal
The rendering of patterns is not usable in its current form, except for a narrow range of zoom levels. To fix it a number of options are available:
My preference is for option 2, as it would cover a lot of use cases, be WebGL1 compatible and isn't too onerous to implement.