Closed xahon closed 5 years ago
Problem didn't happened when i used ID
(Uppercase) field in my struct
Hm, I'll tag this as a bug and maybe be able to tackle it when I port over the sqlite3 support to v3.
Stale. Not sure if this problem reproduces itself on v3 with latest sqlite3
What version of SQLBoiler are you using (
sqlboiler --version
)?SQLBoiler v2.6.0
Minimal code to reproduce
Ouput of GO:
Output of sqliteBrowser: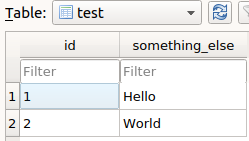
P.S. If you change table fieldname
id
toidd
and struct nameId
toIdd
it works like a charm P.P.S Problem occurs if you will useuid
andUid
fields P.P.P.S Problem occurs if you will useguid
andGuid
fields