Closed zengqinglei closed 3 years ago
@maliming Hi: Is there a repair plan for this problem? Thank you!
I have tried, there is indeed a problem as you said.
Castle-windsor-ms-adapter
will support net core 3.0. We need to wait for hikalkan to handle this problem, the problem is beyond my ability. :)
@maliming ok!
Fixed by volosoft/castle-windsor-ms-adapter#38
Instructions When I use HttpClient and join HttpClientMessageHandler, an exception occurs:Cannot access a disposed object.
This exception occurs when I request more than twice
Run Environment .net core: 2.2 castle.windsor: 5.0.1
Minimal repro steps
Create asp.net core 2.2 api project
Inject HttpClient in Startup
Use the Castle.Windsor container
The code in ApiController is as follows:
Demo code: https://github.com/zengqinglei/TestHttpClientHandlerDispose.git
Then after starting the project, the second time the page is refreshed, the following exception occurs: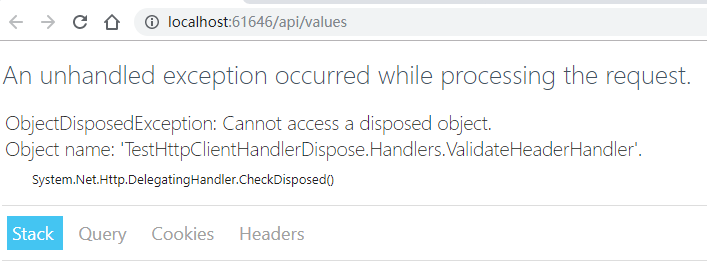
I use the Autofac container and it works fine! I hope to get your reply as soon as possible, thank you!