Open krupis opened 3 years ago
You can try to read directly from SerialAT
or SerialMon
which was used to initialize the modem
example
while(SerialAT.available())
Serial.write(SerialAT.read());
}
Use sendAT
with waitResponse
, just remember that the sendAT
command already includes the "AT". So sendAT(GF("AT"))
actually sends "ATAT". If you want to check signal/CSQ, you'd only include the "+CSQ" bit, not the "AT+CSQ": modem.sendAT(GF("+CSQ"));
If you're expecting "OK" within 1s, all you need is the sendAT with an empty waitResponse. The result of the waitResponse is 1 for 'OK', 2 for 'ERROR'. If you want to wait longer or less than 1s, use waitResponse(5000L)
with whatever the expected wait is. If you're expecting some other response (or one from a list of possible responses) you can add those in to the waitResponse command: waitResponse(timeout_ms, GF("CONNECT OK" GSM_NL), GF("CONNECT FAIL" GSM_NL), GF("ALREADY CONNECT" GSM_NL), GF("ERROR" GSM_NL), GF("CLOSE OK" GSM_NL));
The GF
is just a abstraction of the flash-string macro to put strings into flash for AVR boards that support it.
Hi, and how can i get the response message for exemple i want to read a message: modem.sendAT("+CMGR=10"); thank you.
@HazemBenAmmar - use the waitResponse(...)
function.
If you're looking to keep the text returned, you can feed a string to wait response and it will populate it:
String res1;
modem.sendAT("+CMGR=10");
modem.waitResponse(1000L, res1);
Hello. I am using this library to program lilygo ESP32 GSM development board. It works fine but I want more flexibility and I want to control what AT commands I want to send and what commands I do not need to send. Can someone explain to me how am I suppose to use sendAT() method correctly? Few questions:
What is GF meaning when calling sendAT() function for example:
modem.sendAT(GF("AT"));
How can I see the response from the command sendAT() command.
For example in my loop I call these methods
void loop()
And the serial monitor returns :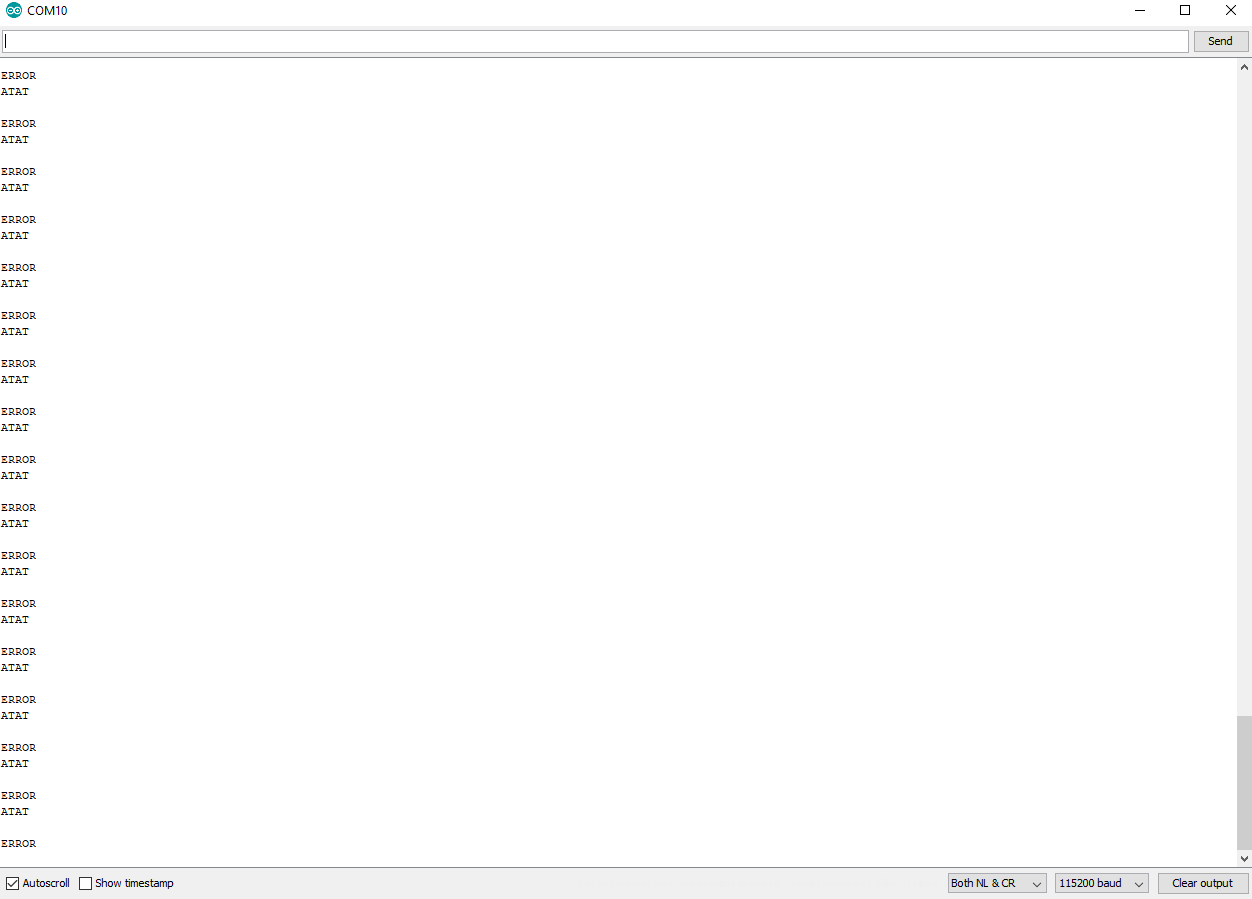
I simply want to know how can I write my own AT commands and retrieve the response