Closed osugiw closed 2 years ago
The documentation:
@osugiw How did you solve the problem. I have a similar problem.
@osugiw How did you solve the problem. I have a similar problem.
Sorry, I little forget how I solved this problem, If I'm not wrong my problem that time because of the baudrate of UART
@osugiw How did you solve the problem. I have a similar problem.
I have just remembered, my problem that time was the sim card provider isn't compatible with SIM7000. You have to look up the datasheet for the bandwidth frequencies that supported in SIM7000.
Thank you for your quick answer. Unfortunately this wasn't my problem.
In case someone has a similar issue than I have, for me it was, that I used the wrong HardwareSerial Pins.
#define ESP32_TX 16 // ESP32 hardware serial RX2 (GPIO16)
#define ESP32_RX 17 // ESP32 hardware serial TX2 (GPIO17)
// For ESP32 hardware serial
#include <HardwareSerial.h>
HardwareSerial SerialAT(1);
and in setup()
:
SerialAT.begin(115200, SERIAL_8N1, ESP32_RX,ESP32_TX); // baud rate, protocol, ESP32 RX pin, ESP32 TX pin
Edit: Changed Code Format
Thank you for your quick answer. Unfortunately this wasn't my problem.
In case someone has a similar issue than I have, for me it was, that I used the wrong HardwareSerial Pins.
#define ESP32_TX 16 // ESP32 hardware serial RX2 (GPIO16) #define ESP32_RX 17 // ESP32 hardware serial TX2 (GPIO17) // For ESP32 hardware serial #include <HardwareSerial.h> HardwareSerial SerialAT(1);
and in
setup()
:SerialAT.begin(115200, SERIAL_8N1, ESP32_RX,ESP32_TX); // baud rate, protocol, ESP32 RX pin, ESP32 TX pin
Edit: Changed Code Format
Hi , there can you provide me the code of your project?
Hi, I was starting with this example https://github.com/vshymanskyy/TinyGSM/blob/master/examples/MqttClient/MqttClient.ino.
Here is my complete code, although I'm not too sure if it was working. 😅
/**************************************************************
*
* For this example, you need to install PubSubClient library:
* https://github.com/knolleary/pubsubclient
* or from http://librarymanager/all#PubSubClient
*
* TinyGSM Getting Started guide:
* https://tiny.cc/tinygsm-readme
*
* For more MQTT examples, see PubSubClient library
*
**************************************************************
* This example connects to HiveMQ's showcase broker.
*
* You can quickly test sending and receiving messages from the HiveMQ webclient
* available at http://www.hivemq.com/demos/websocket-client/.
*
* Subscribe to the topic GsmClientTest/ledStatus
* Publish "toggle" to the topic GsmClientTest/led and the LED on your board
* should toggle and you should see a new message published to
* GsmClientTest/ledStatus with the newest LED status.
*
**************************************************************/
// Select your modem:
// #define TINY_GSM_MODEM_SIM800
// #define TINY_GSM_MODEM_SIM808
// #define TINY_GSM_MODEM_SIM868
// #define TINY_GSM_MODEM_SIM900
#define TINY_GSM_MODEM_SIM7000
// #define TINY_GSM_MODEM_SIM7000SSL
// #define TINY_GSM_MODEM_SIM7080
// #define TINY_GSM_MODEM_SIM5360
// #define TINY_GSM_MODEM_SIM7600
// #define TINY_GSM_MODEM_UBLOX
// #define TINY_GSM_MODEM_SARAR4
// #define TINY_GSM_MODEM_M95
// #define TINY_GSM_MODEM_BG96
// #define TINY_GSM_MODEM_A6
// #define TINY_GSM_MODEM_A7
// #define TINY_GSM_MODEM_M590
// #define TINY_GSM_MODEM_MC60
// #define TINY_GSM_MODEM_MC60E
// #define TINY_GSM_MODEM_ESP8266
// #define TINY_GSM_MODEM_XBEE
// #define TINY_GSM_MODEM_SEQUANS_MONARCH
#include "mySim7000.h"
#include "mySim7x00.h"
// Set serial for debug console (to the Serial Monitor, default speed 115200)
#define SerialMon Serial
#define ESP32_TX 16 // ESP32 hardware serial RX2 (GPIO16)
#define ESP32_RX 17 // ESP32 hardware serial TX2 (GPIO17)
// For ESP32 hardware serial
#include <HardwareSerial.h>
// HardwareSerial SerialAT(1);
/*
// Set serial for AT commands (to the module)
// Use Hardware Serial on Mega, Leonardo, Micro
#ifndef __AVR_ATmega328P__
#define SerialAT Serial1
// or Software Serial on Uno, Nano
#else
#include <SoftwareSerial.h>
SoftwareSerial SerialAT(2, 3); // RX, TX
#endif
*/
// See all AT commands, if wanted
// #define DUMP_AT_COMMANDS
// Define the serial console for debug prints, if needed
#define TINY_GSM_DEBUG SerialMon
// Range to attempt to autobaud
// NOTE: DO NOT AUTOBAUD in production code. Once you've established
// communication, set a fixed baud rate using modem.setBaud(#).
#define GSM_AUTOBAUD_MIN 9600
#define GSM_AUTOBAUD_MAX 115200
// #define GSM_AUTOBAUD_MAX 9600
// Add a reception delay, if needed.
// This may be needed for a fast processor at a slow baud rate.
// #define TINY_GSM_YIELD() { delay(2); }
// Define how you're planning to connect to the internet.
// This is only needed for this example, not in other code.
#define TINY_GSM_USE_GPRS true
#define TINY_GSM_USE_WIFI false
// set GSM PIN, if any
#define GSM_PIN ""
// Your GPRS credentials, if any
const char apn[] = "scs.telekom.tma.iot";
const char gprsUser[] = "";
const char gprsPass[] = "";
// Your WiFi connection credentials, if applicable
const char wifiSSID[] = "YourSSID";
const char wifiPass[] = "YourWiFiPass";
// MQTT details
const char* broker = "IP";
// const uint16_t mqttPort = 1883;
const unsigned short mqttPort = 35648;
const char* topicLed = "GsmClientTest/led";
const char* topicInit = "GsmClientTest/init";
const char* topicLedStatus = "GsmClientTest/ledStatus";
#include <PubSubClient.h>
#include <TinyGsmClient.h>
// Just in case someone defined the wrong thing..
#if TINY_GSM_USE_GPRS && not defined TINY_GSM_MODEM_HAS_GPRS
#undef TINY_GSM_USE_GPRS
#undef TINY_GSM_USE_WIFI
#define TINY_GSM_USE_GPRS false
#define TINY_GSM_USE_WIFI true
#endif
#if TINY_GSM_USE_WIFI && not defined TINY_GSM_MODEM_HAS_WIFI
#undef TINY_GSM_USE_GPRS
#undef TINY_GSM_USE_WIFI
#define TINY_GSM_USE_GPRS true
#define TINY_GSM_USE_WIFI false
#endif
#ifdef DUMP_AT_COMMANDS
#include <StreamDebugger.h>
StreamDebugger debugger(SerialAT, SerialMon);
TinyGsm modem(debugger);
#else
// TinyGsm modem(SerialAT);
#endif
// TinyGsmClient client(modem);
// PubSubClient mqtt(client);
#define LED_PIN 13
int ledStatus = LOW;
uint32_t lastReconnectAttempt = 0;
uint32_t loop_counter = 0;
uint32_t rate = GSM_AUTOBAUD_MAX;
sim7000 SIM7000X(1, rate, ESP32_RX, ESP32_TX);
void setup() {
char* apn = (char*)"scs.telekom.tma.iot";
// char* apn = (char*)"lpwa.vodafone.com";
// Set console baud rate
SerialMon.begin(115200);
// Start at default SIM7000 shield baud rate
// SerialAT.begin(rate, SERIAL_8N1, ESP32_RX,
// ESP32_TX); // baud rate, protocol, ESP32 RX pin, ESP32 TX pin
delay(10);
pinMode(LED_PIN, OUTPUT);
// !!!!!!!!!!!
// Set your reset, enable, power pins here
// !!!!!!!!!!!
SerialMon.println("Wait...");
// SIM7000X.Send_AT_Command("AT+CGATT?", 1000, "+CGATT: 1", "+CGATT: 0");
// SIM7000X.Get_Signal_Value();
// if (SIM7000X.Set_Network_APN(apn)) {
// printf("Setting network apn to %s successfully.\n", apn);
// } else if (SIM7000X.Get_Current_APN(apn)) {
// printf("Current network apn is %s.\n", apn);
// } else {
// printf("Setting network apn to %s failed.\n", apn);
// }
// SIM7000X.Bring_Up_Connection();
uint8_t bands[] = {8,20};
uint8_t band_count = 2;
bool ret = SIM7000X.InitConnection(apn, PreferredLTEMode::NB_IoT, bands, band_count);
if (!ret) {
printf("Init connection failed.\n");
return;
}
SIM7000X.Get_Local_IP();
SIM7000X.Open_Socket_Client("UDP", "172.27.131.100", 15683);
Serial.println("Sending data...");
uint32_t randomInt = random(10000, 99999);
char buffer[260];
char* message = (char*)"ESP32 Message NB-IoT 32 Byte Test Nachricht 01NB-IoT 32 Byte Test Nachricht "
"01NB-IoT 32 Byte Test Nachricht 01NB-IoT 32 Byte Test Nachricht 01NB-IoT 32 Byte Test Nachricht "
"01NB-IoT 32 Byte Test Nachricht 01NB-IoT 32 Byte Test Nachricht 01NB-IoT 32 B";
sprintf(buffer, "%d: %s", randomInt, message);
SIM7000X.Send_Data(buffer,
256);
Serial.println("Data sent.");
// Serial.println("Receiving data...\n");
SIM7000X.Close_Connect();
Serial.println("Sending complete");
/*
// Set GSM module baud rate
TinyGsmAutoBaud(SerialAT, GSM_AUTOBAUD_MIN, GSM_AUTOBAUD_MAX);
// SerialAT.begin(9600);
delay(6000);
// Restart takes quite some time
// To skip it, call init() instead of restart()
SerialMon.println("Initializing modem...");
modem.setPreferredMode(2);
// modem.restart();
modem.init();
*/
}
void loop() {
// if ((loop_counter + 10) % 100 == 0) {
// SerialMon.print("Signal quality:");
// SerialMon.println(modem.getSignalQuality());
// modem.sendAT(GF("+CSQ"));
// SerialMon.print("Signal quality CMD send: ");
// SerialMon.print(modem.waitResponse(GF("+CSQ:")));
// SerialMon.println(modem.stream.readStringUntil('\n'));
// SerialMon.print("Is network connected: ");
// SerialMon.println(modem.getLocalIP());
// SerialMon.println(modem.isNetworkConnected());
// }
// if (loop_counter == 100) {
// SerialMon.println("sending UDP Message");
// }
delay(300);
loop_counter++;
}
Hi everyone, currently I'm working on ESP32 and SIM7000C Waveshare NB-IOT . What I'm trying to do is sending AT Command from Serial monitor to SIM7000C Waveshare extension board through serial communication (RX and TX). The wiring diagram that I use look like this:
I'm using code example from TinyGSM which is ALLFunctions example and changing the modem type into SIM7000 and add hardwareserial code. here's the code:
`/** *
**/
// Select your modem: //#define TINY_GSM_MODEM_SIM800 // #define TINY_GSM_MODEM_SIM808 // #define TINY_GSM_MODEM_SIM868 // #define TINY_GSM_MODEM_SIM900
define TINY_GSM_MODEM_SIM7000
// #define TINY_GSM_MODEM_SIM7000SSL // #define TINY_GSM_MODEM_SIM7080 // #define TINY_GSM_MODEM_SIM5360 // #define TINY_GSM_MODEM_SIM7600 // #define TINY_GSM_MODEM_UBLOX // #define TINY_GSM_MODEM_SARAR4 // #define TINY_GSM_MODEM_M95 // #define TINY_GSM_MODEM_BG96 // #define TINY_GSM_MODEM_A6 // #define TINY_GSM_MODEM_A7 // #define TINY_GSM_MODEM_M590 // #define TINY_GSM_MODEM_MC60 // #define TINY_GSM_MODEM_MC60E // #define TINY_GSM_MODEM_ESP8266 // #define TINY_GSM_MODEM_XBEE // #define TINY_GSM_MODEM_SEQUANS_MONARCH
// Set serial for debug console (to the Serial Monitor, default speed 115200)
define SerialMon Serial
// Set serial for AT commands (to the module) // Use Hardware Serial on Mega, Leonardo, Micro
ifndef __AVR_ATmega328P__
define SerialAT Serial1
// or Software Serial on Uno, Nano
else
include
//SoftwareSerial SerialAT(2, 3); // RX, TX HardwareSerial Serial1(1);
define SerialAT Serial1
endif
// See all AT commands, if wanted // #define DUMP_AT_COMMANDS
// Define the serial console for debug prints, if needed
define TINY_GSM_DEBUG SerialMon
// Range to attempt to autobaud // NOTE: DO NOT AUTOBAUD in production code. Once you've established // communication, set a fixed baud rate using modem.setBaud(#).
define GSM_AUTOBAUD_MIN 9600
define GSM_AUTOBAUD_MAX 57600
// Add a reception delay, if needed. // This may be needed for a fast processor at a slow baud rate. // #define TINY_GSM_YIELD() { delay(2); }
/*
define TINY_GSM_TEST_GPRS true
define TINY_GSM_TEST_WIFI false
define TINY_GSM_TEST_TCP true
define TINY_GSM_TEST_SSL true
define TINY_GSM_TEST_CALL false
define TINY_GSM_TEST_SMS false
define TINY_GSM_TEST_USSD false
define TINY_GSM_TEST_BATTERY true
define TINY_GSM_TEST_TEMPERATURE true
define TINY_GSM_TEST_GSM_LOCATION false
define TINY_GSM_TEST_NTP false
define TINY_GSM_TEST_TIME false
define TINY_GSM_TEST_GPS false
// disconnect and power down modem after tests
define TINY_GSM_POWERDOWN false
// set GSM PIN, if any
define GSM_PIN ""
// Set phone numbers, if you want to test SMS and Calls // #define SMS_TARGET "+380xxxxxxxxx" // #define CALL_TARGET "+380xxxxxxxxx"
// Your GPRS credentials, if any const char apn[] = "internet"; // const char apn[] = "ibasis.iot"; const char gprsUser[] = ""; const char gprsPass[] = "";
// Your WiFi connection credentials, if applicable const char wifiSSID[] = "*"; const char wifiPass[] = "**";
// Server details to test TCP/SSL const char server[] = "vsh.pp.ua"; const char resource[] = "/TinyGSM/logo.txt";
include
if TINY_GSM_TEST_GPRS && not defined TINY_GSM_MODEM_HAS_GPRS
undef TINY_GSM_TEST_GPRS
undef TINY_GSM_TEST_WIFI
define TINY_GSM_TEST_GPRS false
define TINY_GSM_TEST_WIFI true
endif
if TINY_GSM_TEST_WIFI && not defined TINY_GSM_MODEM_HAS_WIFI
undef TINY_GSM_USE_GPRS
undef TINY_GSM_USE_WIFI
define TINY_GSM_USE_GPRS true
define TINY_GSM_USE_WIFI false
endif
ifdef DUMP_AT_COMMANDS
include
StreamDebugger debugger(SerialAT, SerialMon); TinyGsm modem(debugger);
else
TinyGsm modem(SerialAT);
endif
void setup() { // Set console baud rate SerialMon.begin(115200); delay(10); SerialAT.begin(115200,SERIAL_8N1,16,17,false); delay(3000); // !!!!!!!!!!! // Set your reset, enable, power pins here // !!!!!!!!!!!
DBG("Wait..."); delay(6000);
// Set GSM module baud rate TinyGsmAutoBaud(SerialAT, GSM_AUTOBAUD_MIN, GSM_AUTOBAUD_MAX); // SerialAT.begin(9600); }
void loop() { // Restart takes quite some time // To skip it, call init() instead of restart() DBG("Initializing modem..."); if (!modem.restart()) { // if (!modem.init()) { DBG("Failed to restart modem, delaying 10s and retrying"); // restart autobaud in case GSM just rebooted // TinyGsmAutoBaud(SerialAT, GSM_AUTOBAUD_MIN, GSM_AUTOBAUD_MAX); return; }
String name = modem.getModemName(); DBG("Modem Name:", name);
String modemInfo = modem.getModemInfo(); DBG("Modem Info:", modemInfo);
if TINY_GSM_TEST_GPRS
// Unlock your SIM card with a PIN if needed if (GSM_PIN && modem.getSimStatus() != 3) { modem.simUnlock(GSM_PIN); }
endif
if TINY_GSM_TEST_WIFI
DBG("Setting SSID/password..."); if (!modem.networkConnect(wifiSSID, wifiPass)) { DBG(" fail"); delay(10000); return; } SerialMon.println(" success");
endif
if TINY_GSM_TEST_GPRS && defined TINY_GSM_MODEM_XBEE
// The XBee must run the gprsConnect function BEFORE waiting for network! modem.gprsConnect(apn, gprsUser, gprsPass);
endif
DBG("Waiting for network..."); if (!modem.waitForNetwork(600000L, true)) { delay(10000); return; }
if (modem.isNetworkConnected()) { DBG("Network connected"); }
if TINY_GSM_TEST_GPRS
DBG("Connecting to", apn); if (!modem.gprsConnect(apn, gprsUser, gprsPass)) { delay(10000); return; }
bool res = modem.isGprsConnected(); DBG("GPRS status:", res ? "connected" : "not connected");
String ccid = modem.getSimCCID(); DBG("CCID:", ccid);
String imei = modem.getIMEI(); DBG("IMEI:", imei);
String imsi = modem.getIMSI(); DBG("IMSI:", imsi);
String cop = modem.getOperator(); DBG("Operator:", cop);
IPAddress local = modem.localIP(); DBG("Local IP:", local);
int csq = modem.getSignalQuality(); DBG("Signal quality:", csq);
endif
if TINY_GSM_TEST_USSD && defined TINY_GSM_MODEM_HAS_SMS
String ussd_balance = modem.sendUSSD("*111#"); DBG("Balance (USSD):", ussd_balance);
String ussd_phone_num = modem.sendUSSD("*161#"); DBG("Phone number (USSD):", ussd_phone_num);
endif
if TINY_GSM_TEST_TCP && defined TINY_GSM_MODEM_HAS_TCP
TinyGsmClient client(modem, 0); const int port = 80; DBG("Connecting to", server); if (!client.connect(server, port)) { DBG("... failed"); } else { // Make a HTTP GET request: client.print(String("GET ") + resource + " HTTP/1.0\r\n"); client.print(String("Host: ") + server + "\r\n"); client.print("Connection: close\r\n\r\n");
}
endif
if TINY_GSM_TEST_SSL && defined TINY_GSM_MODEM_HAS_SSL
TinyGsmClientSecure secureClient(modem, 1); const int securePort = 443; DBG("Connecting securely to", server); if (!secureClient.connect(server, securePort)) { DBG("... failed"); } else { // Make a HTTP GET request: secureClient.print(String("GET ") + resource + " HTTP/1.0\r\n"); secureClient.print(String("Host: ") + server + "\r\n"); secureClient.print("Connection: close\r\n\r\n");
}
endif
if TINY_GSM_TEST_CALL && defined TINY_GSM_MODEM_HAS_CALLING && \
DBG("Calling:", CALL_TARGET);
// This is NOT supported on M590 res = modem.callNumber(CALL_TARGET); DBG("Call:", res ? "OK" : "fail");
if (res) { delay(1000L);
}
endif
if TINY_GSM_TEST_SMS && defined TINY_GSM_MODEM_HAS_SMS && defined SMS_TARGET
res = modem.sendSMS(SMS_TARGET, String("Hello from ") + imei); DBG("SMS:", res ? "OK" : "fail");
// This is only supported on SIMxxx series res = modem.sendSMS_UTF8_begin(SMS_TARGET); if (res) { auto stream = modem.sendSMS_UTF8_stream(); stream.print(F("Привіііт! Print number: ")); stream.print(595); res = modem.sendSMS_UTF8_end(); } DBG("UTF8 SMS:", res ? "OK" : "fail");
endif
if TINY_GSM_TEST_GSM_LOCATION && defined TINY_GSM_MODEM_HAS_GSM_LOCATION
float lat = 0; float lon = 0; float accuracy = 0; int year = 0; int month = 0; int day = 0; int hour = 0; int min = 0; int sec = 0; for (int8_t i = 15; i; i--) { DBG("Requesting current GSM location"); if (modem.getGsmLocation(&lat, &lon, &accuracy, &year, &month, &day, &hour, &min, &sec)) { DBG("Latitude:", String(lat, 8), "\tLongitude:", String(lon, 8)); DBG("Accuracy:", accuracy); DBG("Year:", year, "\tMonth:", month, "\tDay:", day); DBG("Hour:", hour, "\tMinute:", min, "\tSecond:", sec); break; } else { DBG("Couldn't get GSM location, retrying in 15s."); delay(15000L); } } DBG("Retrieving GSM location again as a string"); String location = modem.getGsmLocation(); DBG("GSM Based Location String:", location);
endif
if TINY_GSM_TEST_GPS && defined TINY_GSM_MODEM_HAS_GPS
DBG("Enabling GPS/GNSS/GLONASS and waiting 15s for warm-up"); modem.enableGPS(); delay(15000L); float lat2 = 0; float lon2 = 0; float speed2 = 0; float alt2 = 0; int vsat2 = 0; int usat2 = 0; float accuracy2 = 0; int year2 = 0; int month2 = 0; int day2 = 0; int hour2 = 0; int min2 = 0; int sec2 = 0; for (int8_t i = 15; i; i--) { DBG("Requesting current GPS/GNSS/GLONASS location"); if (modem.getGPS(&lat2, &lon2, &speed2, &alt2, &vsat2, &usat2, &accuracy2, &year2, &month2, &day2, &hour2, &min2, &sec2)) { DBG("Latitude:", String(lat2, 8), "\tLongitude:", String(lon2, 8)); DBG("Speed:", speed2, "\tAltitude:", alt2); DBG("Visible Satellites:", vsat2, "\tUsed Satellites:", usat2); DBG("Accuracy:", accuracy2); DBG("Year:", year2, "\tMonth:", month2, "\tDay:", day2); DBG("Hour:", hour2, "\tMinute:", min2, "\tSecond:", sec2); break; } else { DBG("Couldn't get GPS/GNSS/GLONASS location, retrying in 15s."); delay(15000L); } } DBG("Retrieving GPS/GNSS/GLONASS location again as a string"); String gps_raw = modem.getGPSraw(); DBG("GPS/GNSS Based Location String:", gps_raw); DBG("Disabling GPS"); modem.disableGPS();
endif
if TINY_GSM_TEST_NTP && defined TINY_GSM_MODEM_HAS_NTP
DBG("Asking modem to sync with NTP"); modem.NTPServerSync("132.163.96.5", 20);
endif
if TINY_GSM_TEST_TIME && defined TINY_GSM_MODEM_HAS_TIME
int year3 = 0; int month3 = 0; int day3 = 0; int hour3 = 0; int min3 = 0; int sec3 = 0; float timezone = 0; for (int8_t i = 5; i; i--) { DBG("Requesting current network time"); if (modem.getNetworkTime(&year3, &month3, &day3, &hour3, &min3, &sec3, &timezone)) { DBG("Year:", year3, "\tMonth:", month3, "\tDay:", day3); DBG("Hour:", hour3, "\tMinute:", min3, "\tSecond:", sec3); DBG("Timezone:", timezone); break; } else { DBG("Couldn't get network time, retrying in 15s."); delay(15000L); } } DBG("Retrieving time again as a string"); String time = modem.getGSMDateTime(DATE_FULL); DBG("Current Network Time:", time);
endif
if TINY_GSM_TEST_BATTERY && defined TINY_GSM_MODEM_HAS_BATTERY
uint8_t chargeState = -99; int8_t percent = -99; uint16_t milliVolts = -9999; modem.getBattStats(chargeState, percent, milliVolts); DBG("Battery charge state:", chargeState); DBG("Battery charge 'percent':", percent); DBG("Battery voltage:", milliVolts / 1000.0F);
endif
if TINY_GSM_TEST_TEMPERATURE && defined TINY_GSM_MODEM_HAS_TEMPERATURE
float temp = modem.getTemperature(); DBG("Chip temperature:", temp);
endif
if TINY_GSM_POWERDOWN
if TINY_GSM_TEST_GPRS
modem.gprsDisconnect(); delay(5000L); if (!modem.isGprsConnected()) { DBG("GPRS disconnected"); } else { DBG("GPRS disconnect: Failed."); }
endif
if TINY_GSM_TEST_WIFI
modem.networkDisconnect(); DBG("WiFi disconnected");
endif
// Try to power-off (modem may decide to restart automatically) // To turn off modem completely, please use Reset/Enable pins modem.poweroff(); DBG("Poweroff.");
endif
DBG("End of tests.");
// Do nothing forevermore while (true) { modem.maintain(); } }`
It couldn't communicate with the extension board because the results look like this: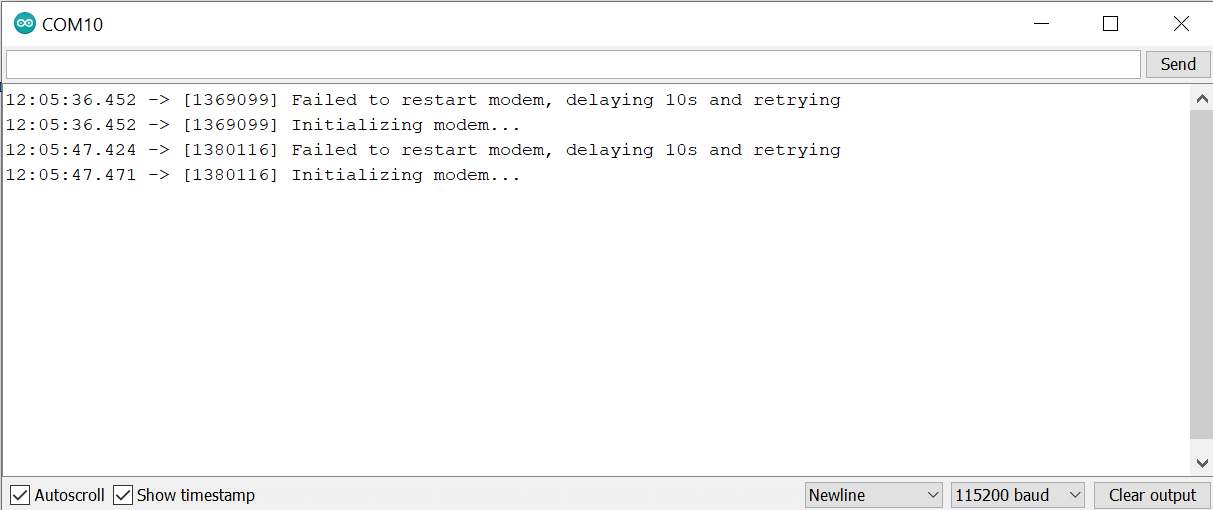
Any advice or help would be appreciate! Thanks everyone!