Open taipower opened 1 year ago
Can anyone help guide me?
Texture need image pixels not file bytes?
@wasabia I think that convert image to Unit8Array. I updated my code in convert image to Unit8Array // load image, create texture and generate mipmaps loadImage('assets/images/thumbnail2.jpg').then((bytes) { gl.texImage2D(GL_TEXTURE_2D, 0, GL_RGB, 1080, 1080, 0, GL_RGB, GL_UNSIGNED_BYTE, Uint8Array.from(bytes.toList())); gl.generateMipmap(GL_TEXTURE_2D); });
Future
// Decode the image
final ui.Codec codec = await ui.instantiateImageCodec(bytes);
final ui.Image image = (await codec.getNextFrame()).image;
// Flip the image vertically
final Uint8List pixelData = await _flipImageVertically(image);
return pixelData;
}
Future
final ByteData? byteData = await image.toByteData();
final Uint8List pixels = Uint8List.fromList(byteData?.buffer.asUint8List() ?? []);
for (int y = 0; y < height ~/ 2; y++) {
final int topOffset = y * width * 4;
final int bottomOffset = (height - y - 1) * width * 4;
for (int x = 0; x < width; x++) {
final int topIndex = topOffset + x * 4;
final int bottomIndex = bottomOffset + x * 4;
final int r = pixels[topIndex];
final int g = pixels[topIndex + 1];
final int b = pixels[topIndex + 2];
final int a = pixels[topIndex + 3];
pixels[topIndex] = pixels[bottomIndex];
pixels[topIndex + 1] = pixels[bottomIndex + 1];
pixels[topIndex + 2] = pixels[bottomIndex + 2];
pixels[topIndex + 3] = pixels[bottomIndex + 3];
pixels[bottomIndex] = r;
pixels[bottomIndex + 1] = g;
pixels[bottomIndex + 2] = b;
pixels[bottomIndex + 3] = a;
}
}
return pixels;
}
But I found problem. Image color is grey only and disproportionate.
My image
Hi @wasabia I can solve the problem now. Thank you for your response.
@taipower how did you solve it? Can you please share your code?
I write code for generate texture on rectangle. My code `import 'dart:async'; import 'dart:ffi'; import 'dart:io'; import 'dart:math'; import 'dart:typed_data';
import 'dart:ui' as ui; import 'package:flutter/foundation.dart'; import 'package:flutter/material.dart'; import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart'; import 'package:flutter_gl/flutter_gl.dart'; import 'package:flutter_gl/openGL/opengl/opengl_es_bindings/opengl_es_bindings.dart';
typedef NativeUint8Array = Pointer;
class ExampleTexture extends StatefulWidget { _MyAppState createState() => _MyAppState(); }
class _MyAppState extends State {
late FlutterGlPlugin flutterGlPlugin;
int? fboId; num dpr = 1.0; late double width; late double height;
ui.Size? screenSize;
dynamic glProgram; dynamic _vao; dynamic _vbo; dynamic _ebo; dynamic _texture;
dynamic sourceTexture;
dynamic defaultFramebuffer; dynamic defaultFramebufferTexture;
int n = 0;
int t = DateTime.now().millisecondsSinceEpoch;
@override void initState() { super.initState();
}
// Platform messages are asynchronous, so we initialize in an async method. Future initPlatformState() async {
width = screenSize!.width;
height = width;
}
setup() async { // web no need use fbo if (!kIsWeb) { await flutterGlPlugin.prepareContext();
}
initSize(BuildContext context) { if (screenSize != null) { return; }
}
@override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: const Text('Example app'), ), body: Builder( builder: (BuildContext context) { initSize(context); return SingleChildScrollView(child: _build(context)); }, ), floatingActionButton: FloatingActionButton( onPressed: () { clickRender(); }, child: Text("Render"), ), ), ); }
Widget _build(BuildContext context) { return Column( children: [ Container( width: width, height: width, color: Colors.black, child: Builder(builder: (BuildContext context) { if (kIsWeb) { return flutterGlPlugin.isInitialized ? HtmlElementView( viewType: flutterGlPlugin.textureId!.toString()) : Container(); } else { return flutterGlPlugin.isInitialized ? Texture(textureId: flutterGlPlugin.textureId!) : Container(); } })), ], ); }
animate() { render();
}
setupDefaultFBO() { final _gl = flutterGlPlugin.gl; int glWidth = (width dpr).toInt(); int glHeight = (height dpr).toInt();
}
clickRender() { print(" click render ... "); render(); }
render() { final _gl = flutterGlPlugin.gl;
}
prepare() { final _gl = flutterGlPlugin.gl;
precision mediump float; // add a precision qualifier
define attribute in
define varying out
layout (location = 0) in vec3 a_Position; layout (location = 1) in vec3 a_Color; layout (location = 2) in vec2 a_TexCoord;
out vec3 ourColor; out vec2 TexCoord;
void main() { gl_Position = vec4(a_Position, 1.0); ourColor = a_Color; TexCoord = vec2(a_TexCoord.x, a_TexCoord.y); } """;
precision mediump float;
out highp vec4 pc_fragColor;
define gl_FragColor pc_fragColor
in highp vec3 ourColor; in highp vec2 TexCoord;
uniform highp sampler2D ourTexture;
void main() { gl_FragColor = texture(ourTexture, TexCoord) * vec4(ourColor, 1.0); } """;
}
initVertexBuffers(gl) { // Vertices var dim = 3;
}
initShaders(gl, vs_source, fs_source) { // Compile shaders var vertexShader = makeShader(gl, vs_source, gl.VERTEX_SHADER); var fragmentShader = makeShader(gl, fs_source, gl.FRAGMENT_SHADER);
}
makeShader(gl, src, type) { var shader = gl.createShader(type); gl.shaderSource(shader, src); gl.compileShader(shader); var _res = gl.getShaderParameter(shader, gl.COMPILE_STATUS); if (_res == 0 || _res == false) { print("Error compiling shader: ${gl.getShaderInfoLog(shader)}"); return; } return shader; }
Future loadImage(String imgPath) async{
ByteData byteData = await rootBundle.load(imgPath);
Uint8List bytes = byteData.buffer.asUint8List();
}
}`
My image
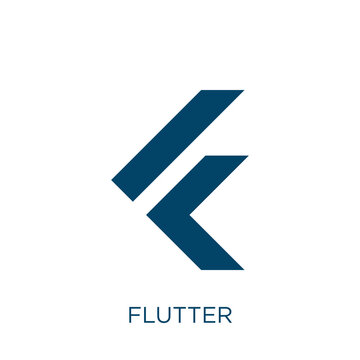
When I run