Open TMD-Burger opened 6 years ago
Great work :) Thanks for sharing
I would be Interested If this could be Installed on a raspberry pie with loxberry Image. I have a e golf at home and would like to see some Information like battery status on my loxone smart Home.
Best regards from Austria Reinhard
Nice work, but shouldn't you create a repo for this? Or create a fork, update this project and submit a PR?
Hi, is this still working, after name-change to We Connect? I cannot get the token-url to work, or to be precise, I am getting unsupported_grant_type exception.
@ottopaulsen, see issue https://github.com/wez3/volkswagen-carnet-client/issues/16
Hello!
As a hobbyist, I'm always happy when someone somewhere in the world has already done something in a certain direction and that's why I wanted to give something to the community. Here are my "research" with the "app interface", which in my opinion is much faster. I'm sure one or the other may need something. This is the python3-code, which has read-only access for my purposes (so far): (Since we only have a vw car with carnet, I have decided (for speed reasons) to simply omit the vehicle search function and program the VIN firmly.)
Looking for the endpoints of the vw-server, I went the way on "android-studio" and found some variables. maybe valuable for someone: (What is missing here is the last expression of url)
Since my origin plan was to make a "homekit to carnet bridge", which i rejected because of insufficient fexibility on the part of homekit. To become the ability of access those information as fast as possible, here is a php script, which practically does the same: (The return-values are in german, but its easy to change, requirements are to install composer, then requests. Google knows how to do that.)
(Here are a screenshot of my "fast" info-website", to get the informations as fast as possible: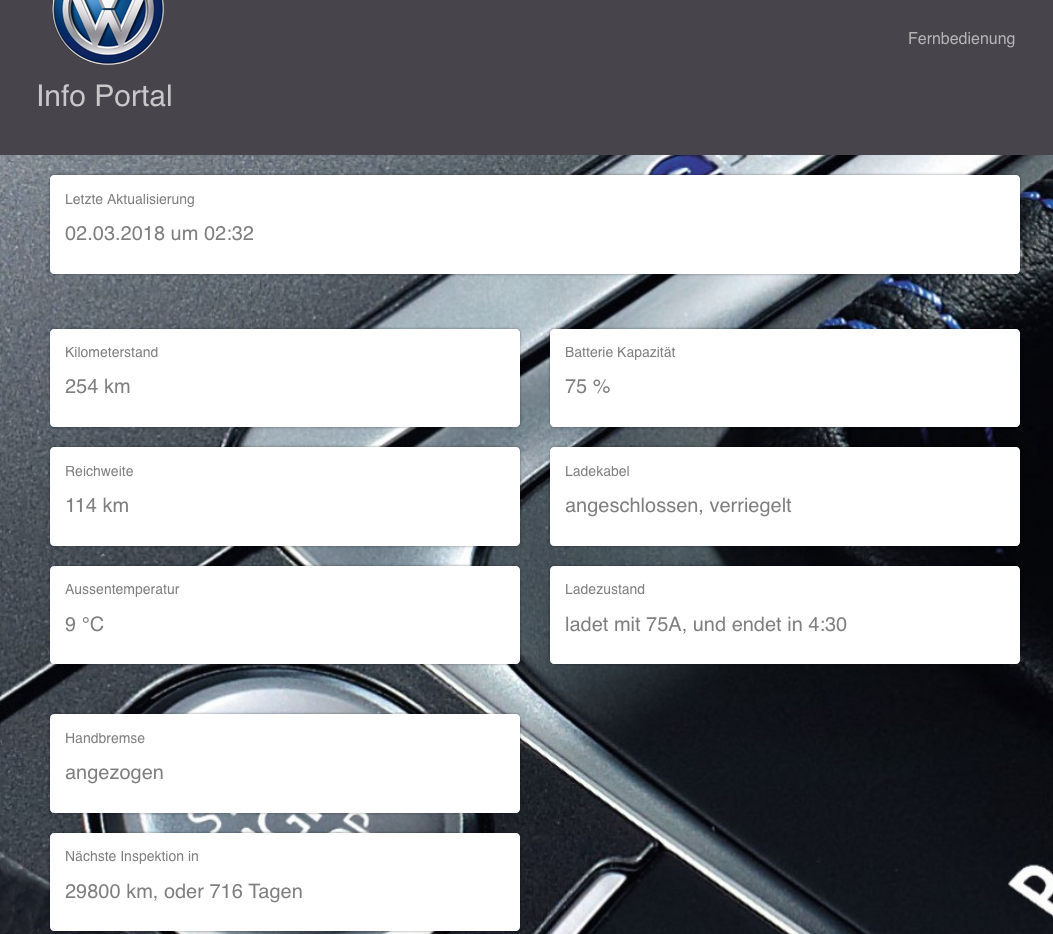
Have fun with it! Stefan ;-)