Closed LTAbhirup closed 1 year ago
Related: #1148. Looks like it may be related to https://github.com/wojtekmaj/react-pdf/issues/1148#issuecomment-1314180559?
@wojtekmaj for now, solved this issue by importing pdfjs from this library and using the workerSrc
pdfjs.GlobalWorkerOptions.workerSrc = //unpkg.com/pdfjs-dist@${pdfjs.version}/build/pdf.worker.min.js
;
Thanks
@wojtekmaj for now, solved this issue by importing pdfjs from this library and using the workerSrc pdfjs.GlobalWorkerOptions.workerSrc =
//unpkg.com/pdfjs-dist@${pdfjs.version}/build/pdf.worker.min.js
;Thanks
import pdfWorker from 'pdfjs-dist/build/pdf.worker.js?url';
import {Document, Page} from 'react-pdf/dist/esm/entry.vite';
import {pdfjs} from "react-pdf";
pdfjs.GlobalWorkerOptions.workerSrc = pdfWorker;
it works with vite3 + ts 4.6 + react 18.2 + react-pdf 6.2.2 compared with your solution, this method does not require external CDN.
Just a small note: if you're manually providing workerSrc, there's no point to keep using Vite-specific entry point which sole purpose is to provide workerSrc. :)
@wojtekmaj for now, solved this issue by importing pdfjs from this library and using the workerSrc pdfjs.GlobalWorkerOptions.workerSrc =
//unpkg.com/pdfjs-dist@${pdfjs.version}/build/pdf.worker.min.js
; Thanksimport pdfWorker from 'pdfjs-dist/build/pdf.worker.js?url'; import {Document, Page} from 'react-pdf/dist/esm/entry.vite'; import {pdfjs} from "react-pdf"; pdfjs.GlobalWorkerOptions.workerSrc = pdfWorker;
it works with vite3 + ts 4.6 + react 18.2 + react-pdf 6.2.2 compared with your solution, this method does not require external CDN.
Do you have a new solution for this, @flymyd?
When using the latest versions, I encounter an error stating that it can't find react-pdf/dist/esm/entry.vite
. Switching to plain react-pdf
resolves the issue. However, a new error surfaces: Failed to resolve import "pdfjs-dist/build/pdf.worker.js?url".
There is no pdf.worker.js
in the directory, only pdf.worker.mjs,
which does not support my import.
@wojtekmaj for now, solved this issue by importing pdfjs from this library and using the workerSrc pdfjs.GlobalWorkerOptions.workerSrc =
//unpkg.com/pdfjs-dist@${pdfjs.version}/build/pdf.worker.min.js
; Thanksimport pdfWorker from 'pdfjs-dist/build/pdf.worker.js?url'; import {Document, Page} from 'react-pdf/dist/esm/entry.vite'; import {pdfjs} from "react-pdf"; pdfjs.GlobalWorkerOptions.workerSrc = pdfWorker;
it works with vite3 + ts 4.6 + react 18.2 + react-pdf 6.2.2 compared with your solution, this method does not require external CDN.
Do you have a new solution for this, @flymyd? When using the latest versions, I encounter an error stating that it can't find
react-pdf/dist/esm/entry.vite
. Switching to plainreact-pdf
resolves the issue. However, a new error surfaces:Failed to resolve import "pdfjs-dist/build/pdf.worker.js?url".
There is nopdf.worker.js
in the directory, onlypdf.worker.mjs,
which does not support my import.
What versions of Vite and react-pdf are used in your project?
@wojtekmaj for now, solved this issue by importing pdfjs from this library and using the workerSrc pdfjs.GlobalWorkerOptions.workerSrc = ; Thanks
//unpkg.com/pdfjs-dist@${pdfjs.version}/build/pdf.worker.min.js
import pdfWorker from 'pdfjs-dist/build/pdf.worker.js?url'; import {Document, Page} from 'react-pdf/dist/esm/entry.vite'; import {pdfjs} from "react-pdf"; pdfjs.GlobalWorkerOptions.workerSrc = pdfWorker;
it works with vite3 + ts 4.6 + react 18.2 + react-pdf 6.2.2 compared with your solution, this method does not require external CDN.
Do you have a new solution for this, @flymyd? When using the latest versions, I encounter an error stating that it can't find . Switching to plain resolves the issue. However, a new error surfaces: There is no in the directory, only which does not support my import.
react-pdf/dist/esm/entry.vite``react-pdf``Failed to resolve import "pdfjs-dist/build/pdf.worker.js?url".``pdf.worker.js``pdf.worker.mjs,
This is a sample with vite@5.0.3 and react-pdf@7.5.1
import 'react-pdf/dist/esm/Page/AnnotationLayer.css';
import 'react-pdf/dist/esm/Page/TextLayer.css';
import {FC, useState} from "react";
import {pdfjs, Document, Page} from 'react-pdf';
pdfjs.GlobalWorkerOptions.workerSrc = new URL(
'pdfjs-dist/build/pdf.worker.min.js',
import.meta.url,
).toString();
const App: FC = () => {
const [numPages, setNumPages] = useState(null);
return (
<Document file="https://test.com/sample.pdf" onLoadSuccess={(e: any) => setNumPages(e.numPages)}>
{Array.from(new Array(numPages), (_el, index) => <Page key={`page_${index + 1}`} pageNumber={index + 1} width={980}/>)}
</Document>
)
}
export default App;
Should be 'pdfjs-dist/build/pdf.worker.min.mjs'
instead of 'pdfjs-dist/build/pdf.worker.min.js'
in version 9.0
using pdfjs.GlobalWorkerOptions.workerSrc = new URL( 'pdfjs-dist/build/pdf.worker.min.mjs', import.meta.url ).toString();
locally works but on the production getting an error Setting up fake worker failed: "Failed to fetch dynamically imported module: https://www.test.com/assets/pdf.worker.min-DGf6LXVo.mjs".
any solution to tackle this?
here is my vite.comfig.ts
export default defineConfig({ plugins: [react()], resolve: { alias: { '@': path.resolve(__dirname, 'src'), }, }, build: { rollupOptions: { output: { entryFileNames:
[name].[hash].mjs, chunkFileNames:
[name].[hash].mjs, }, }, }, define: { 'process.env': {} }, optimizeDeps: { esbuildOptions: { plugins: [fixReactVirtualized], }, }, });
when checking the network, the file is there but seems like is commented out!
using pdfjs.GlobalWorkerOptions.workerSrc = new URL( 'pdfjs-dist/build/pdf.worker.min.mjs', import.meta.url ).toString();
locally works but on the production getting an error Setting up fake worker failed: "Failed to fetch dynamically imported module: https://www.test.com/assets/pdf.worker.min-DGf6LXVo.mjs".
any solution to tackle this?
here is my vite.comfig.ts
export default defineConfig({ plugins: [react()], resolve: { alias: { '@': path.resolve(__dirname, 'src'), }, }, build: { rollupOptions: { output: { entryFileNames:
[name].[hash].mjs, chunkFileNames:
[name].[hash].mjs, }, }, }, define: { 'process.env': {} }, optimizeDeps: { esbuildOptions: { plugins: [fixReactVirtualized], }, }, });
when checking the network, the file is there but seems like is commented out!
Facing the same issue.
Before you start - checklist
Description
I am getting this error when using vite entry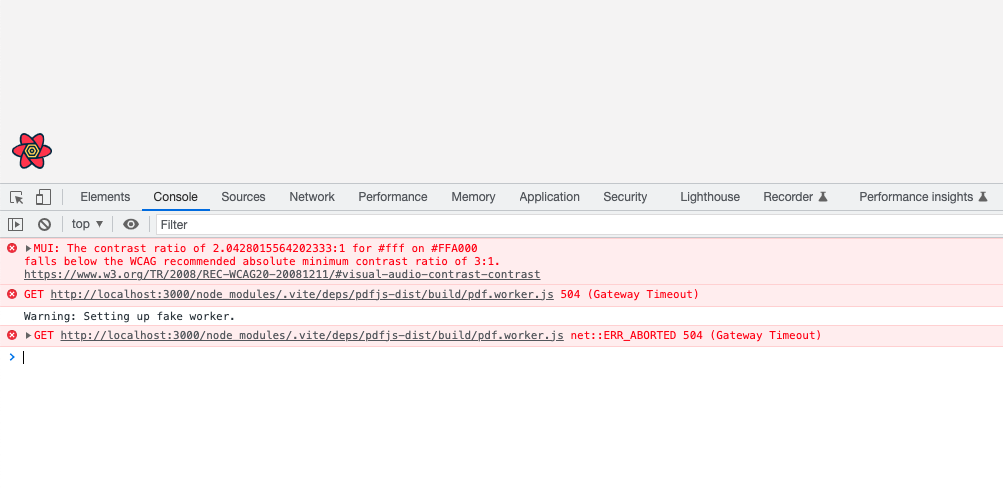
Steps to reproduce
Code:
Expected behavior
Should render the pdf
Actual behavior
Webpage crashing
Additional information
No response
Environment