Open qq516249940 opened 2 years ago
go version: 1.18
package main import ( "crypto/tls" "fmt" "log" "net/http" "github.com/cavaliercoder/go-zabbix" ) func main() { // Default approach - without session caching session, err := zabbix.NewSession("http://zabbix/api_jsonrpc.php", "Admin", "zabbix") if err != nil { panic(err) } version, err := session.GetVersion() if err != nil { panic(err) } fmt.Printf("Connected to Zabbix API v%s", version) // Use session builder with caching. // You can use own cache by implementing SessionAbstractCache interface // Optionally an http.Client can be passed to the builder, allowing to skip TLS verification, // pass proxy settings, etc. client := &http.Client{ Transport: &http.Transport{ TLSClientConfig: &tls.Config{ InsecureSkipVerify: true } } } cache := zabbix.NewSessionFileCache().SetFilePath("./zabbix_session") session, err := zabbix.CreateClient("http://zabbix/api_jsonrpc.php"). WithCache(cache). WithHTTPClient(client). WithCredentials("Admin", "zabbix"). Connect() if err != nil { log.Fatalf("%v\n", err) } version, err := session.GetVersion() if err != nil { log.Fatalf("%v\n", err) } fmt.Printf("Connected to Zabbix API v%s", version) }
./main.go:35:29: syntax error: unexpected newline, expecting comma or } ./main.go:36:5: syntax error: unexpected newline, expecting comma or } ./main.go:37:4: syntax error: unexpected newline, expecting comma or }
go version: 1.18
command-line-arguments
./main.go:35:29: syntax error: unexpected newline, expecting comma or } ./main.go:36:5: syntax error: unexpected newline, expecting comma or } ./main.go:37:4: syntax error: unexpected newline, expecting comma or }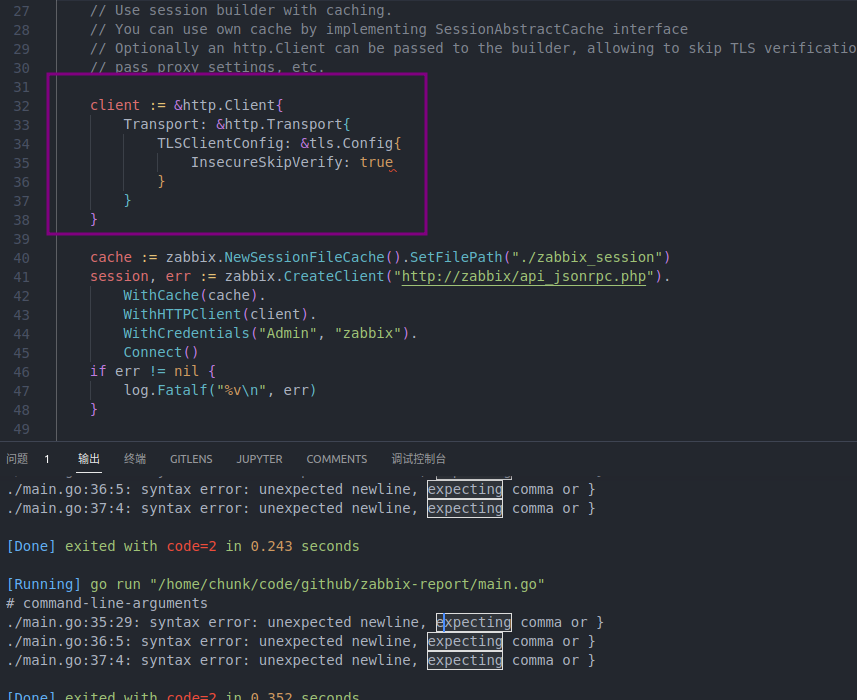