Open gobullsclub opened 2 years ago
@gobullsclub could you share the code of the contract?
code means = source code, not the compiled binary
//SPDX-License-Identifier: MIT
pragma solidity ^0.8.7;
import "contracts/token/ERC721/extensions/ERC721Enumerable.sol"; import "contracts/access/Ownable.sol";
contract GoBulls is ERC721Enumerable, Ownable { using Strings for uint256;
string public baseURI;
string public baseExtension = ".json";
uint256 public cost = 0.05 ether;
uint256 public presaleCost = 0.03 ether;
uint256 public maxSupply = 1024;
uint256 public maxMintAmount = 1023;
bool public paused = false;
mapping(address => bool) public whitelisted;
mapping(address => bool) public presaleWallets;
constructor(
string memory _name,
string memory _symbol,
string memory _initBaseURI
) ERC721(_name, _symbol) {
setBaseURI(_initBaseURI);
mint(msg.sender, 2);
}
// internal
function _baseURI() internal view virtual override returns (string memory) {
return baseURI;
}
// public
function mint(address _to, uint256 _mintAmount) public payable {
uint256 supply = totalSupply();
require(!paused);
require(_mintAmount > 0);
require(_mintAmount <= maxMintAmount);
require(supply + _mintAmount <= maxSupply);
if (msg.sender != owner()) {
if (whitelisted[msg.sender] != true) {
if (presaleWallets[msg.sender] != true) {
//general public
require(msg.value >= cost * _mintAmount);
} else {
//presale
require(msg.value >= presaleCost * _mintAmount);
}
}
}
for (uint256 i = 1; i <= _mintAmount; i++) {
_safeMint(_to, supply + i);
}
}
function walletOfOwner(address _owner)
public
view
returns (uint256[] memory)
{
uint256 ownerTokenCount = balanceOf(_owner);
uint256[] memory tokenIds = new uint256[](ownerTokenCount);
for (uint256 i; i < ownerTokenCount; i++) {
tokenIds[i] = tokenOfOwnerByIndex(_owner, i);
}
return tokenIds;
}
function tokenURI(uint256 tokenId)
public
view
virtual
override
returns (string memory)
{
require(
_exists(tokenId),
"ERC721Metadata: URI query for nonexistent token"
);
string memory currentBaseURI = _baseURI();
return
bytes(currentBaseURI).length > 0
? string(
abi.encodePacked(
currentBaseURI,
tokenId.toString(),
baseExtension
)
)
: "";
}
//only owner
function setCost(uint256 _newCost) public onlyOwner {
cost = _newCost;
}
function setPresaleCost(uint256 _newCost) public onlyOwner {
presaleCost = _newCost;
}
function setmaxMintAmount(uint256 _newmaxMintAmount) public onlyOwner {
maxMintAmount = _newmaxMintAmount;
}
function setBaseURI(string memory _newBaseURI) public onlyOwner {
baseURI = _newBaseURI;
}
function setBaseExtension(string memory _newBaseExtension)
public
onlyOwner
{
baseExtension = _newBaseExtension;
}
function pause(bool _state) public onlyOwner {
paused = _state;
}
function whitelistUser(address _user) public onlyOwner {
whitelisted[_user] = true;
}
function removeWhitelistUser(address _user) public onlyOwner {
whitelisted[_user] = false;
}
function addPresaleUser(address _user) public onlyOwner {
presaleWallets[_user] = true;
}
function add100PresaleUsers(address[100] memory _users) public onlyOwner {
for (uint256 i = 0; i < 2; i++) {
presaleWallets[_users[i]] = true;
}
}
function removePresaleUser(address _user) public onlyOwner {
presaleWallets[_user] = false;
}
function withdraw() public payable onlyOwner {
(bool success, ) = payable(msg.sender).call{
value: address(this).balance
}("");
require(success);
}
}
i think the problem is its a Multipart Files ?the NFT contract is not a single file..multipartfiles ,BTW What EVM should is choose?
code means = source code, not the compiled binary
Yes ,i used the Code .But Didnt Work,The Screenshot above is just trying. Please Advice .
@gobullsclub please use flattened contract Web3 command line tool could generate it - https://github.com/gochain/web3 (also it could upload and verify as well)
Are you saying i can't Verify a MutilPart NFT Contract? and the contract must be flattened using CLI ..can't use Remix IDE?Because i prefer to use Remix IDE ,easy work..just a plugin
You cannot verify multipart contract because we cannot build it without having all files of the contract, please download all files of your contract and upload/verify using web3 tool
Does Remix have a way of verifying? Or exporting the contract to verify?
Okay I'll update soon..After Trying Out The Remix Flattened Contract Method.
On Thu, Feb 24, 2022, 02:18 r-gochain @.***> wrote:
You cannot verify multipart contract because we cannot build it without having all files of the contract, please download all files of your contract and upload/verify using web3 tool
— Reply to this email directly, view it on GitHub https://github.com/zeromintapp/community/issues/15#issuecomment-1049073776, or unsubscribe https://github.com/notifications/unsubscribe-auth/AXLJ5TD7SLACKFQ64ABDGLLU4UQFZANCNFSM5MRLUO6Q . Triage notifications on the go with GitHub Mobile for iOS https://apps.apple.com/app/apple-store/id1477376905?ct=notification-email&mt=8&pt=524675 or Android https://play.google.com/store/apps/details?id=com.github.android&referrer=utm_campaign%3Dnotification-email%26utm_medium%3Demail%26utm_source%3Dgithub.
You are receiving this because you were mentioned.Message ID: @.***>
Not sure,I'll check and update here soon,thanks guys!
On Thu, Feb 24, 2022, 04:16 Travis Reeder @.***> wrote:
Does Remix have a way of verifying? Or exporting the contract to verify?
— Reply to this email directly, view it on GitHub https://github.com/zeromintapp/community/issues/15#issuecomment-1049175393, or unsubscribe https://github.com/notifications/unsubscribe-auth/AXLJ5TFUZJWAZKX4WQCDC33U4U6BRANCNFSM5MRLUO6Q . Triage notifications on the go with GitHub Mobile for iOS https://apps.apple.com/app/apple-store/id1477376905?ct=notification-email&mt=8&pt=524675 or Android https://play.google.com/store/apps/details?id=com.github.android&referrer=utm_campaign%3Dnotification-email%26utm_medium%3Demail%26utm_source%3Dgithub.
You are receiving this because you were mentioned.Message ID: @.***>
Collaborator
What EVM should is choose?
petersburg I believe
Okay thanks Travis for the input , will try very soon!
On Thu, Feb 24, 2022, 11:34 PM Travis Reeder @.***> wrote:
petersburg I believe
— Reply to this email directly, view it on GitHub https://github.com/zeromintapp/community/issues/15#issuecomment-1049981713, or unsubscribe https://github.com/notifications/unsubscribe-auth/AXLJ5TBJOENSXWWD37MXET3U4ZFZRANCNFSM5MRLUO6Q . Triage notifications on the go with GitHub Mobile for iOS https://apps.apple.com/app/apple-store/id1477376905?ct=notification-email&mt=8&pt=524675 or Android https://play.google.com/store/apps/details?id=com.github.android&referrer=utm_campaign%3Dnotification-email%26utm_medium%3Demail%26utm_source%3Dgithub.
You are receiving this because you were mentioned.Message ID: @.***>
@gobullsclub there is a list of EMVs and compile versions (you should use that as on remix)
make sure that compiler version and EVM version are the same
I've Tried With All the Information Given above : Same Error as Above
Contract Name = GoBulls or Gobulls_flat (Both Tried) Compiler : 0.87 EVM Version:Petersburg Optimization: Checked
Hello Guys ,@treeder @r-gochain Any Suggestion Or there is something wrong i did?
@gobullsclub could you put your contract into a gist and I'll try to build it. Btw I still insist you should build/deploy/verify with web3 command line tool, because we use the same approach on the server side (vanila solc from docker container)
oh I've just notices the source code, let me play with it
Okay, I'll try again with gist and I'm gonna learn the CLI method of deploying smart contract, I've already install the CLI on my Windows Machine!
@gobullsclub @r-gochain found an issue with more recent solidity, so I think once the fix is rolled out, you can try again and we'll see if it works.
Noted, thanks Travis!
On Tue, Mar 1, 2022, 7:59 PM Travis Reeder @.***> wrote:
@gobullsclub https://github.com/gobullsclub @r-gochain https://github.com/r-gochain found an issue with more recent solidity, so I think once the fix is rolled out, you can try again and we'll see if it works.
— Reply to this email directly, view it on GitHub https://github.com/zeromintapp/community/issues/15#issuecomment-1055358612, or unsubscribe https://github.com/notifications/unsubscribe-auth/AXLJ5TGJKUW3G37CMBPJ373U5YBAVANCNFSM5MRLUO6Q . Triage notifications on the go with GitHub Mobile for iOS https://apps.apple.com/app/apple-store/id1477376905?ct=notification-email&mt=8&pt=524675 or Android https://play.google.com/store/apps/details?id=com.github.android&referrer=utm_campaign%3Dnotification-email%26utm_medium%3Demail%26utm_source%3Dgithub.
You are receiving this because you were mentioned.Message ID: @.***>
@gobullsclub please use the following approach: 1) In GoBulls_flat.sol remove unnecessary pragma solidity lines and add one in the beginning - pragma solidity ^0.8.7;
2) Build/Deploy/Verify using the following command:
WEB3_PRIVATE_KEY=0x4e6
web3 contract build GoBulls_flat.sol && web3 --network testnet contract deploy --verify GoBulls_flat.sol GoBulls.bin
Hello Guys @treeder @r-gochain ,how do i verify a NFT Contract On GoChain?What should i use for the Contract Code ? AbiCode ,Bytecode or The Transact Data from Remix.Ethereum.Org ?.I Fill up all the Details ,but got an error. What should i choose for the EVM option?Default?I'm using the current/latest settings on Remix.Ethereum.Org
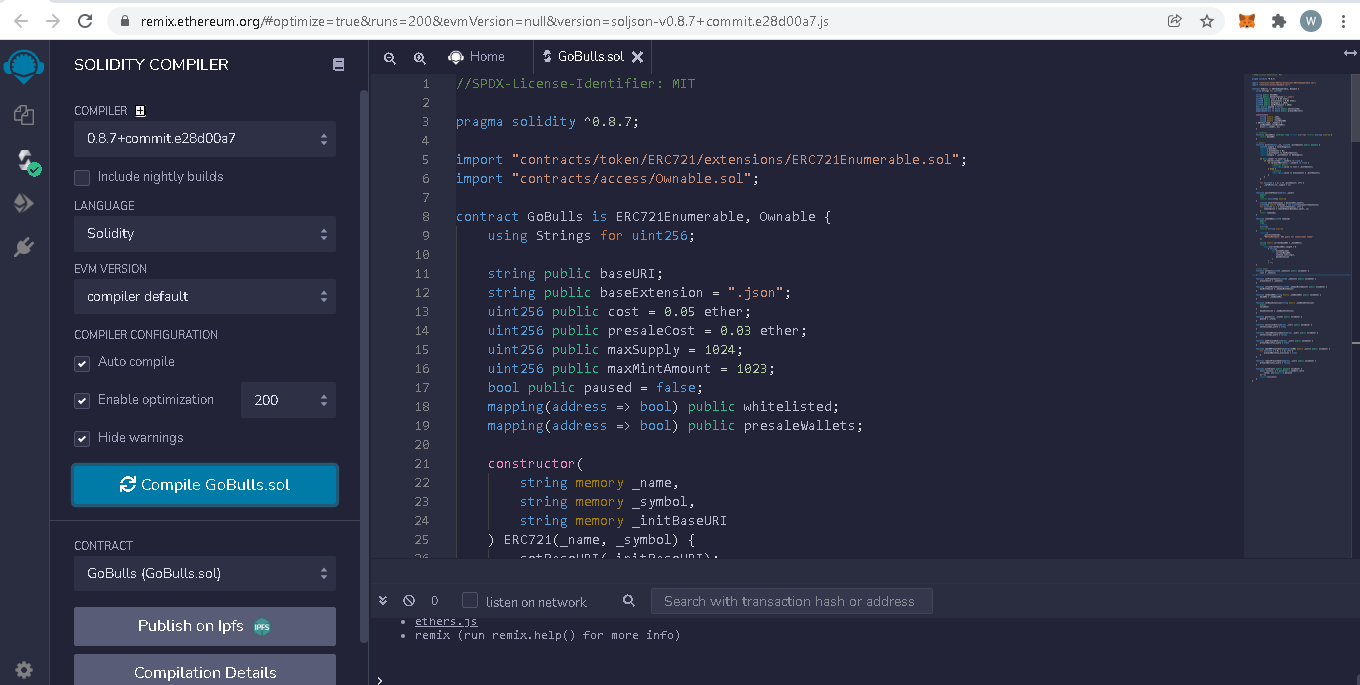