Closed Eichenherz closed 1 year ago
In your renderer, how do you define projWidth
? Typically znear shouldn't affect the projection results, but if you define projWidth
/projHeight
as the view-space bounds of the camera slice at znear (so the size of the front clipped plane of the viewing frustum), then yeah - you'd need to divide.
I prefer the formulation where projection width/height define the size of the image plane at Z=1; this definition doesn't depend on znear, which means you can vary znear to adjust for clipping without changing the projection math.
inline DirectX::XMMATRIX PerspInvDepthInfFovLH( float fovYRads, float aspectRatioWH, float zNear )
{
float sinFov;
float cosFov;
DirectX::XMScalarSinCos( &sinFov, &cosFov, fovYRads * 0.5f );
float h = cosFov / sinFov;
float w = h / aspectRatioWH;
DirectX::XMMATRIX proj;
proj.r[ 0 ] = DirectX::XMVectorSet( w, 0, 0, 0 );
proj.r[ 1 ] = DirectX::XMVectorSet( 0, h, 0, 0 );
proj.r[ 2 ] = DirectX::XMVectorSet( 0, 0, 0, zNear );
proj.r[ 3 ] = DirectX::XMVectorSet( 0, 0, 1, 0 );
return proj;
}
projWidth would be w, projHeight would be h From the nvidia paper I figured it out. They explicitly multiply the extreme points on the sphere by proj and it would result in 1/znear as being a scaling coef.
I'm not sure I know how to toggle between Z=1 formulation and what I currently have.
if( visible && LATE_PASS ){
vec3 viewSpaceCenter = ( globs.view * vec4( center.x, center.y, center.z, 1.0f ) ).xyz;
if( viewSpaceCenter.z > radius + cullInfo.zNear ){
vec4 aabb = ProjectedSphereToAABB( viewSpaceCenter, radius,
cullInfo.projWidth / cullInfo.zNear,
cullInfo.projHeight / cullInfo.zNear );
......
and ProjectedSphereToAABB is identical to what niagara does.
Hello Zeux ! I've been dealing with a bug in the "fast/compact" sphere projection
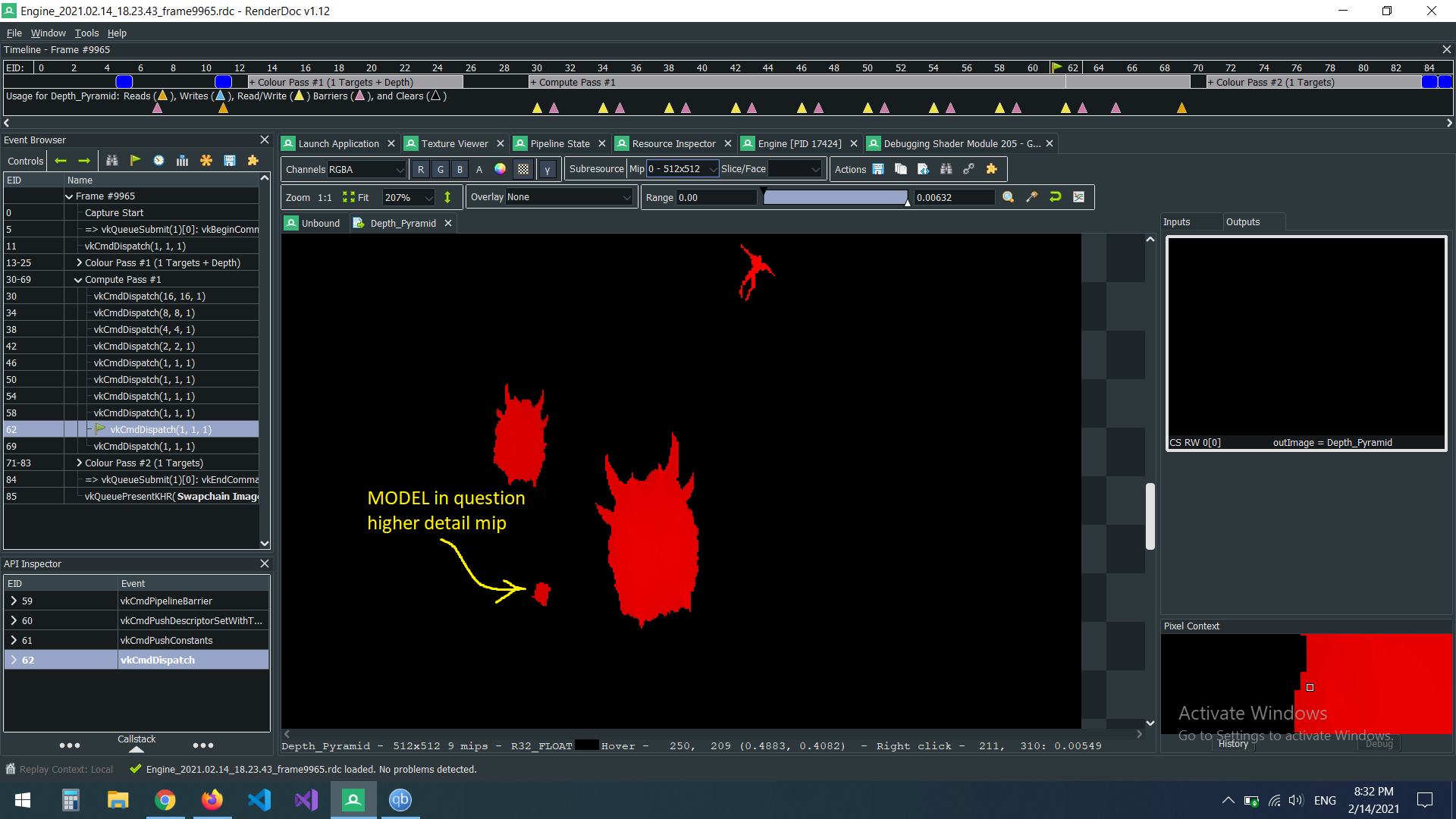
Basically , I was getting occlusion culling on "the object in question" but only in a "Bermuda Tiangle" region, and it shouldn't have happen because it was visible. The issue is produced by the quick and dirty projection, namely it lacks the 1/zNear as a factor:
Took me a while to figure out :) Cheers !