Open sulthanalihsan opened 1 year ago
Hi, @sulthanalihsan, where you able to fix this? I'm currently having a similar issue. The debugger throws the following error: HttpLinkServerException
and I haven't had any luck catching it. I know for sure that the API server is not running so I expect to get this error. The problem is not being able to handle it. My code is below
void someFunc() async {
try {
final result = await client.mutate(
MutationOptions(
document: gql(someMutation),
variables: <String, String>{
"var": "value",
},
// This gets printed but result is not catched.
onError: (OperationException? error) => debugPrint(error),
)
);
} catch(err) {
// We never get here.
debugPrint(err);
}
}
Isn't this a very old issue that was supposed to had been fixed in v3.0.0? @HofmannZ
@sulthanalihsan @axelrogg I was able to overcome this issue by unchecking "Uncaught Exceptions" from Breakpoints in vscode debugger. Suggested here
Give it a try.
Might be an issue with the vscode debugger, as we are technically "catching" the exception.
When open the issue please add the reproducer otherwise will be closed at some point when I will scan again the issue
import 'package:flutter/material.dart';
import 'package:graphql_flutter/graphql_flutter.dart';
void main() {
//final HttpLink graphqlLink = HttpLink("https://graphql-pokemon2.vercel.app");
final HttpLink graphqlLink = HttpLink("https://fake.link/graphql");
final AuthLink authLink = AuthLink(getToken: () => null);
final Link link = authLink.concat(graphqlLink);
ValueNotifier<GraphQLClient> graphqlClient = ValueNotifier(
GraphQLClient(
link: link,
cache: GraphQLCache(store: InMemoryStore()),
),
);
runApp(
GraphQLProvider(
client: graphqlClient,
child: const MyApp(),
),
);
}
class MyApp extends StatefulWidget {
const MyApp({super.key});
@override
State<MyApp> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
String data = "[not loaded...]";
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: ListView(children: [
TextButton(
onPressed: clickHandle,
child: const Text("Load pokemons"),
),
Center(child: Text(data)),
]),
),
);
}
void clickHandle() {
var gqlClient = GraphQLProvider.of(context).value;
try {
gqlClient
.query(
QueryOptions(
document: gql("""
query {
pokemons(first: 5) {
name
}
}
"""),
onError: (error) => print(error),
),
)
.catchError((e) => print(e))
.then((value) => setState(
() => data = value.data!["pokemons"].toString(),
));
} catch (e) {
print(e);
}
}
}
Exception dont catched with catch
block or catchError
in Future.
Hi, thankyou for making this excellent package
i have a question about my error, previously i have successfully request to the server, but I didn't get a response and get the error as below, It can be see that there is response in that error
the error
I also tried something like this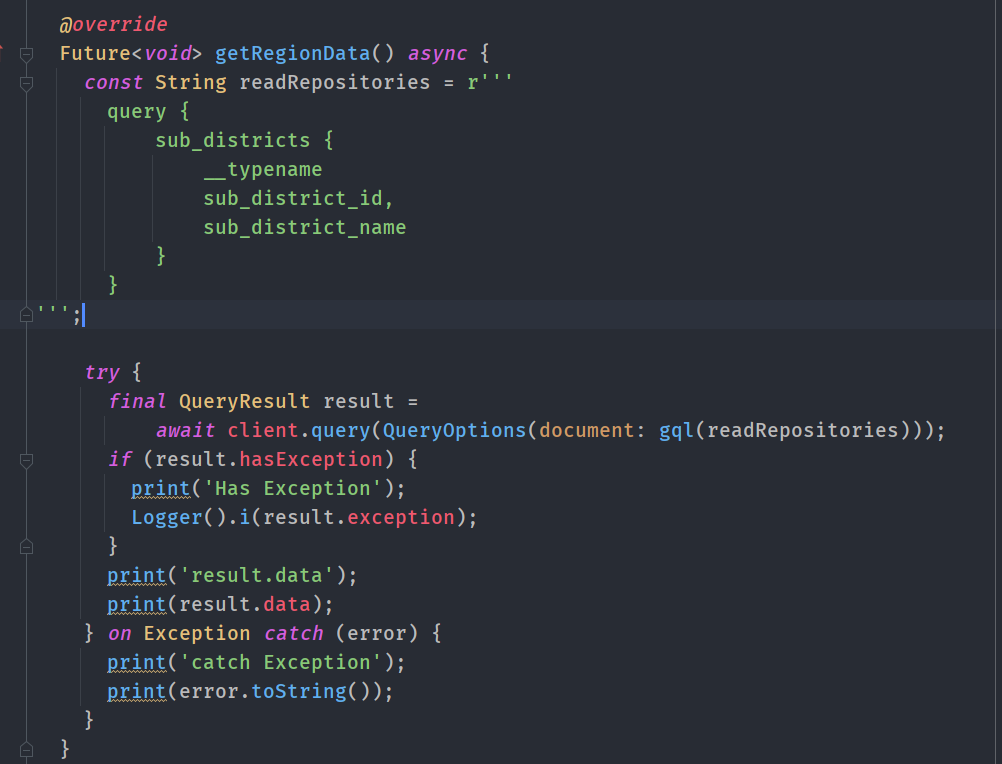
what is wrong with my code? thanks