Documentation ·
Report a Bug ·
Examples .
Request Feature ·
Send a Pull Request
FTXUI
Functional Terminal (X) User interface
A simple cross-platform C++ library for terminal based user interfaces!
Feature
- Functional style. Inspired by
1
and React
- Simple and elegant syntax (in my opinion)
- Keyboard & mouse navigation.
- Support for UTF8 and fullwidth chars (→ 测试)
- Support for animations. Demo 1, Demo 2
- Support for drawing. Demo
- No dependencies
- Cross platform: Linux/MacOS (main target), WebAssembly, Windows (Thanks to contributors!).
- Learn by examples, and tutorials
- Multiple packages: CMake FetchContent (preferred), vcpkg, pkgbuild, conan.
- Good practices: documentation, tests, fuzzers, performance tests, automated CI, automated packaging, etc...
Documentation
Example
vbox({
hbox({
text("one") | border,
text("two") | border | flex,
text("three") | border | flex,
}),
gauge(0.25) | color(Color::Red),
gauge(0.50) | color(Color::White),
gauge(0.75) | color(Color::Blue),
});

Short gallery
DOM
This module defines a hierarchical set of Element. An Element manages layout and can be responsive to the terminal dimensions.
They are declared in <ftxui/dom/elements.hpp>
Layout
Element can be arranged together:
- horizontally with `hbox`
- vertically with `vbox`
- inside a grid with `gridbox`
- wrap along one direction using the `flexbox`.
Element can become flexible using the the `flex` decorator.
[Example](https://arthursonzogni.github.io/FTXUI/examples_2dom_2vbox_hbox_8cpp-example.html) using `hbox`, `vbox` and `filler`.
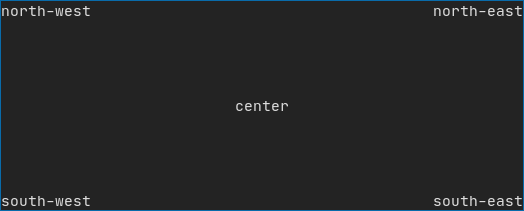
[Example](https://arthursonzogni.github.io/FTXUI/examples_2dom_2gridbox_8cpp-example.html) using gridbox:
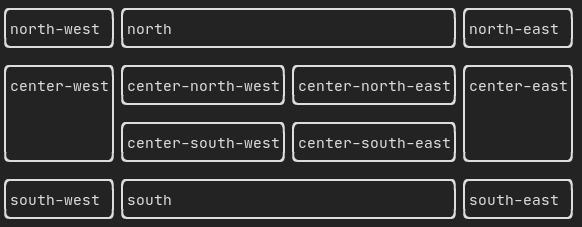
[Example](https://github.com/ArthurSonzogni/FTXUI/blob/master/examples/dom/hflow.cpp) using flexbox:
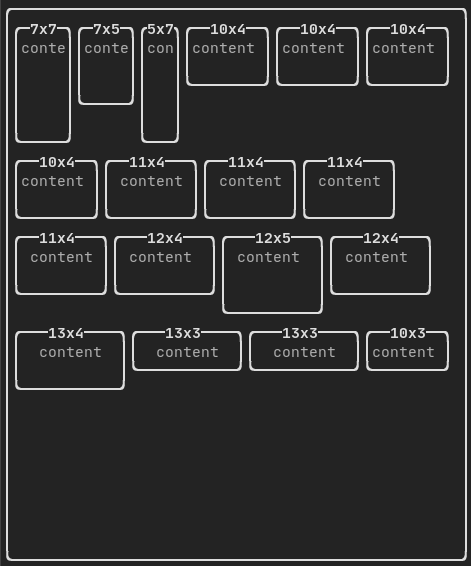
[See](https://arthursonzogni.github.io/FTXUI/examples_2dom_2hflow_8cpp-example.html) also this [demo](https://arthursonzogni.github.io/FTXUI/examples/?file=component/flexbox).
Style
An element can be decorated using the functions:
- `bold`
- `dim`
- `inverted`
- `underlined`
- `underlinedDouble`
- `blink`
- `strikethrough`
- `color`
- `bgcolor`
- `hyperlink`
[Example](https://arthursonzogni.github.io/FTXUI/examples_2dom_2style_gallery_8cpp-example.html)

FTXUI supports the pipe operator. It means: `decorator1(decorator2(element))` and `element | decorator1 | decorator2` can be used.
Colors
FTXUI support every color palette:
Color [gallery](https://arthursonzogni.github.io/FTXUI/examples_2dom_2color_gallery_8cpp-example.html):
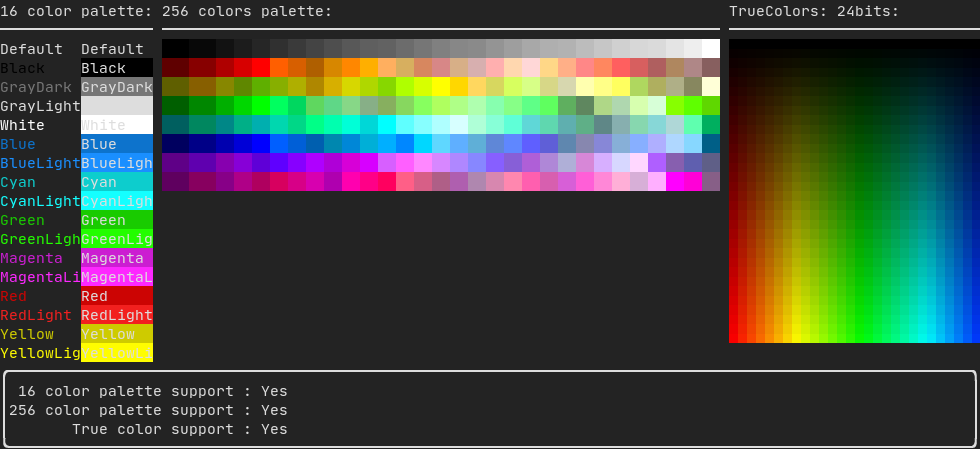
Border and separator
Use decorator border and element separator() to subdivide your UI:
```cpp
auto document = vbox({
text("top"),
separator(),
text("bottom"),
}) | border;
```
[Demo](https://arthursonzogni.github.io/FTXUI/examples_2dom_2separator_8cpp-example.html):

Text and paragraph
A simple piece of text is represented using `text("content")`.
To support text wrapping following spaces the following functions are provided:
```cpp
Element paragraph(std::string text);
Element paragraphAlignLeft(std::string text);
Element paragraphAlignRight(std::string text);
Element paragraphAlignCenter(std::string text);
Element paragraphAlignJustify(std::string text);
```
[Paragraph example](https://arthursonzogni.github.io/FTXUI/examples_2dom_2paragraph_8cpp-example.html)
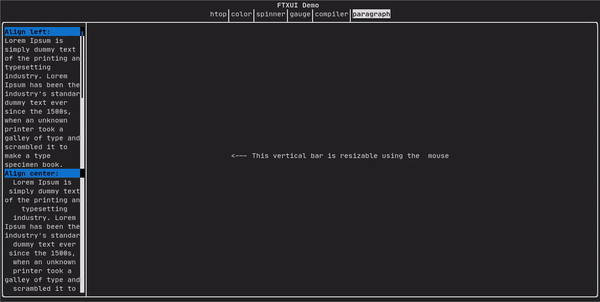
Table
A class to easily style a table of data.
[Example](https://arthursonzogni.github.io/FTXUI/examples_2dom_2table_8cpp-example.html):
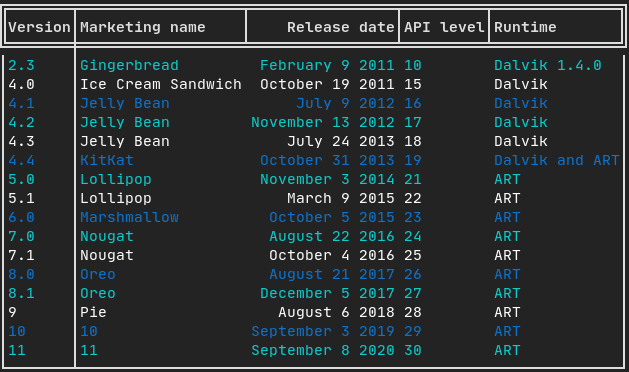
Canvas
Drawing can be made on a Canvas, using braille, block, or simple characters:
Simple [example](https://github.com/ArthurSonzogni/FTXUI/blob/master/examples/dom/canvas.cpp):
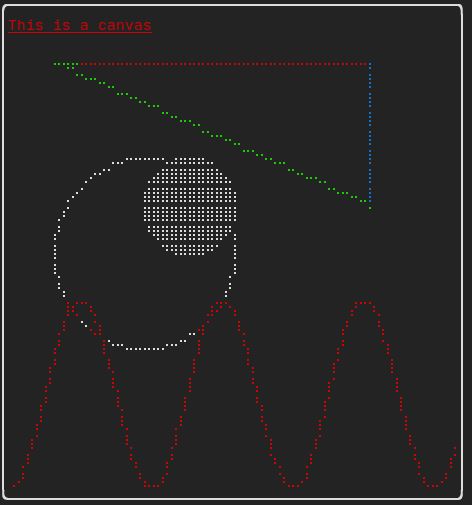
Complex [examples](https://github.com/ArthurSonzogni/FTXUI/blob/master/examples/component/canvas_animated.cpp):
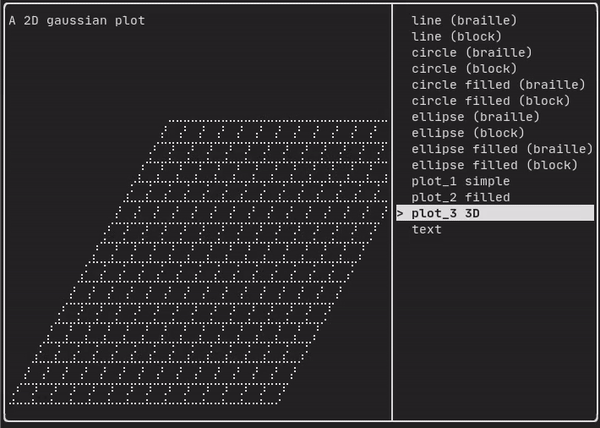
Component
ftxui/component produces dynamic UI, reactive to the user's input. It defines a set of ftxui::Component. A component reacts to Events (keyboard, mouse, resize, ...) and Renders as an Element (see previous section).
Prebuilt components are declared in <ftxui/component/component.hpp>
Gallery
[Gallery](https://arthursonzogni.github.io/FTXUI/examples_2component_2gallery_8cpp-example.html) of multiple components. ([demo](https://arthursonzogni.github.io/FTXUI/examples/?file=component/gallery))
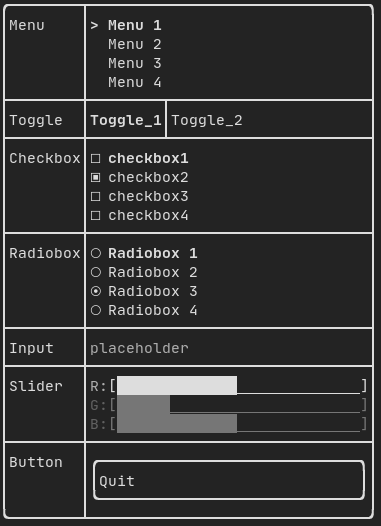
Radiobox
[Example](https://arthursonzogni.github.io/FTXUI/examples_2component_2radiobox_8cpp-example.html):
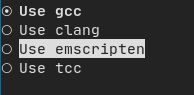
Checkbox
[Example](https://arthursonzogni.github.io/FTXUI/examples_2component_2checkbox_8cpp-example.html):
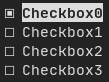
Input
[Example](https://arthursonzogni.github.io/FTXUI/examples_2component_2input_8cpp-example.html):
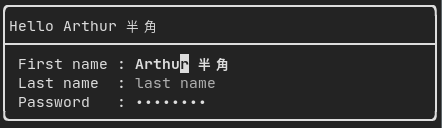
Toggle
[Example](https://arthursonzogni.github.io/FTXUI/examples_2component_2toggle_8cpp-example.html):

Slider
[Example](https://arthursonzogni.github.io/FTXUI/examples_2component_2slider_8cpp-example.html):
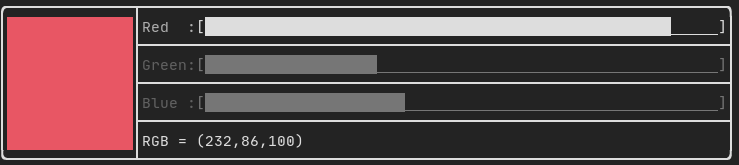
Menu
[Example](https://arthursonzogni.github.io/FTXUI/examples_2component_2menu_8cpp-example.html):

ResizableSplit
[Example](https://arthursonzogni.github.io/FTXUI/examples_2component_2resizable_split_8cpp-example.html):
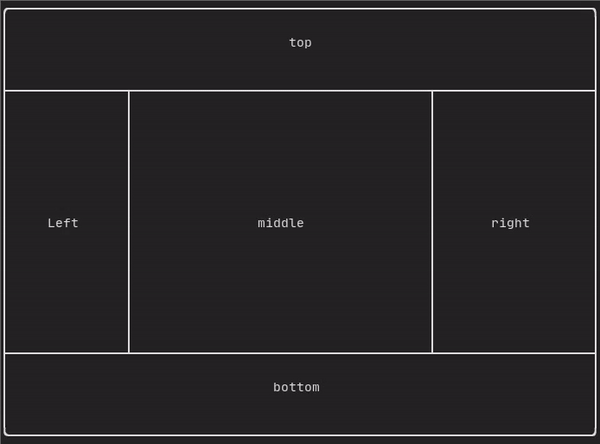
Dropdown
[Example](https://arthursonzogni.github.io/FTXUI/examples_2component_2dropdown_8cpp-example.html):
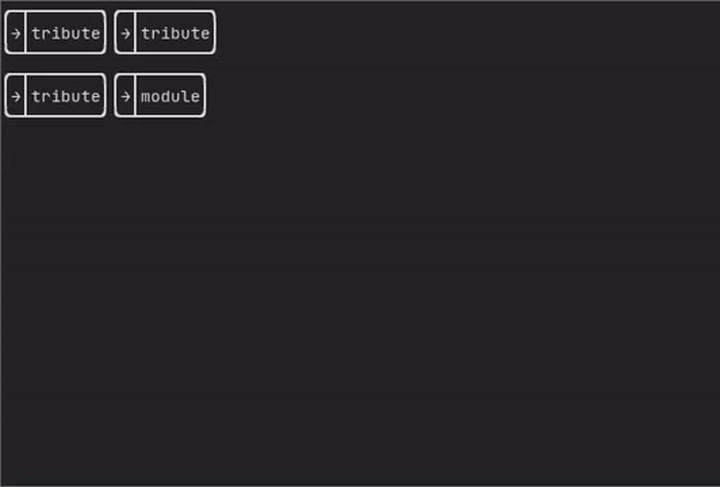
Tab
[Vertical](https://arthursonzogni.github.io/FTXUI/examples_2component_2tab_vertical_8cpp-example.html):
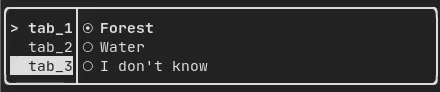
[Horizontal](https://arthursonzogni.github.io/FTXUI/examples_2component_2tab_horizontal_8cpp-example.html):
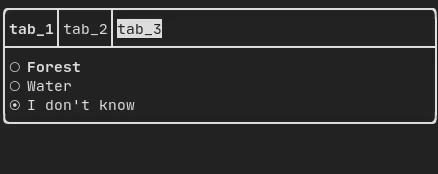
Libraries for FTXUI
Project using FTXUI
Feel free to add your projects here:
Several games using the FTXUI have been made during the Game Jam:
Utilization
It is highly recommended to use CMake FetchContent to depend on FTXUI so you may specify which commit you would like to depend on.
include(FetchContent)
FetchContent_Declare(ftxui
GIT_REPOSITORY https://github.com/ArthurSonzogni/ftxui
GIT_TAG v5.0.0
)
FetchContent_GetProperties(ftxui)
if(NOT ftxui_POPULATED)
FetchContent_Populate(ftxui)
add_subdirectory(${ftxui_SOURCE_DIR} ${ftxui_BINARY_DIR} EXCLUDE_FROM_ALL)
endif()
If you don't, FTXUI may be used from the following packages:
If you choose to build and link FTXUI yourself, ftxui-component
must be first in the linking order relative to the other FTXUI libraries, i.e.
g++ . . . -lftxui-component -lftxui-dom -lftxui-screen . . .
Contributors