Open danielolsen opened 2 years ago
Proposed implementation plan, part 1: refactor build_hydro and add a feature flag (toggle). Goal of the feature is to better represent the monthly energy totals from EIA 923. "Better represent" will be assessed on the basis of R^2 and MAPE, comparing against prior metrics generated by Daniel O. and reported in the issue. The code provided in the issue can be used to directly assess these metrics. Actual values for 2020 will be retrieved with the same API key as we use to grab the BA-level hydro generation. Actual values will also be used to create scaling factors which will be applied to our profiles.
Plan part 2: Identify pumped hydro vs. conventional hydro and clip profile of conventional hydro with minimum value of 0. Assess against R^2 and MAPE to see if this is an improvement. If so, implement this additional improvement.
:rocket:
Describe the workflow you want to enable
Currently, when building hydro profiles for the HIFLD grid model using
prereise.gather.griddata.hifld.data_process.profiles.build_hydro
, we use the balancing-authority-aggregated generation shape (after filtering and imputing) to populate the time-series profile for each hydro plant. There is additional information we could incorporate into these profiles however: information from EIA Form 923 which provides monthly net generation on a plant-level resolution.Context
Looking at the existing time-series outputs of
build_hydro
and comparing to values from EIA 923, we see pretty significant differences, mostly for the smaller generators+month combinations but some significant differences exist for the larger ones as well.For 2020 (the most recent year of full final data), the r-squared is 0.895, which seems pretty good! Raw MAPE is about 1200% for the 1,389 matching plants (seems pretty bad), although when we weight this by the generation for each (plant + month) combination, the weighted MAPE is about 40%: much better, but still not great. Part of this could be that there is a large chunk of data missing for CISO in this year, so CISO plants use an averaged profiles of all the other BAs. The following plots all show the same information, with different axis scales:
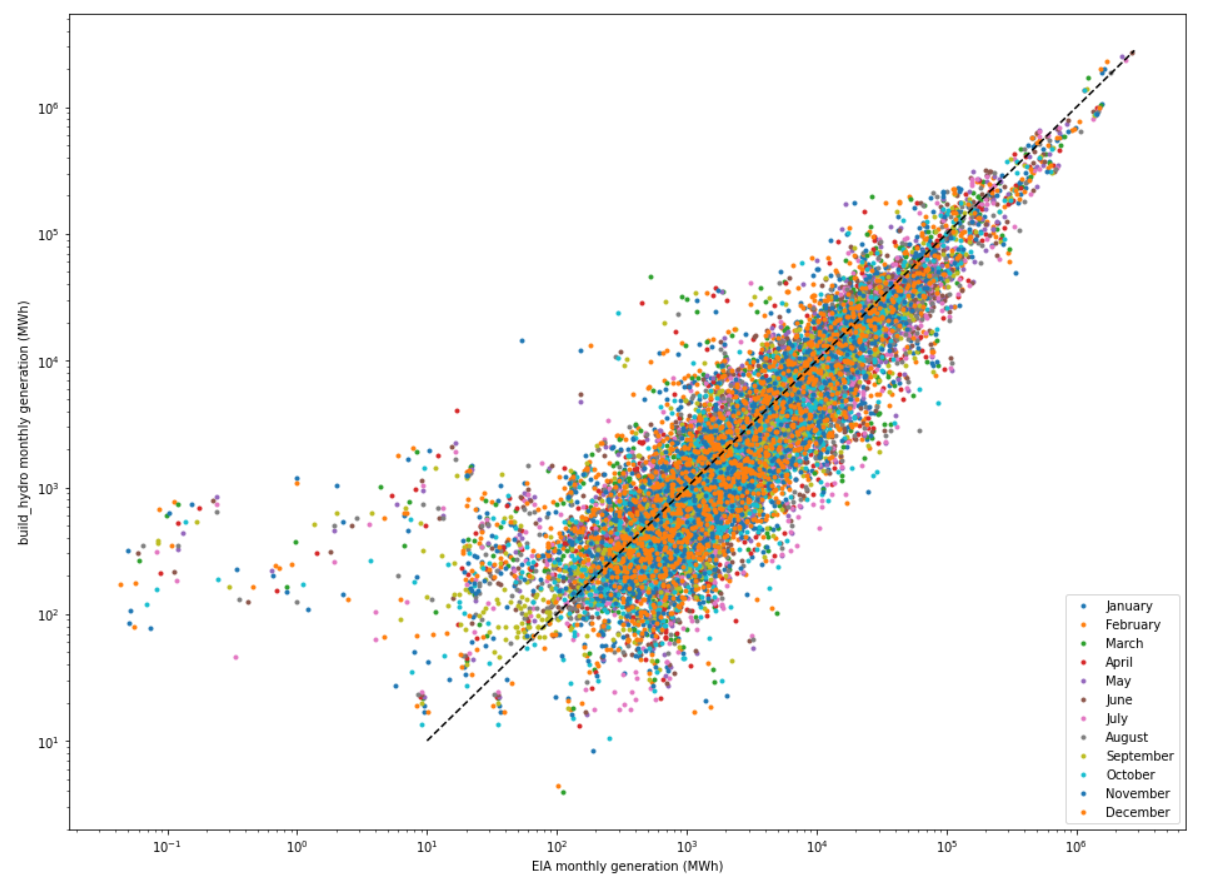
For 2021 (final data not yet available), there are only 135 matching plants (only those reporting monthly). The raw MAPE is worse than 2020, nearly 1600%, but the weighted MAPE is better, about 34%, and the r-squared is 0.897.
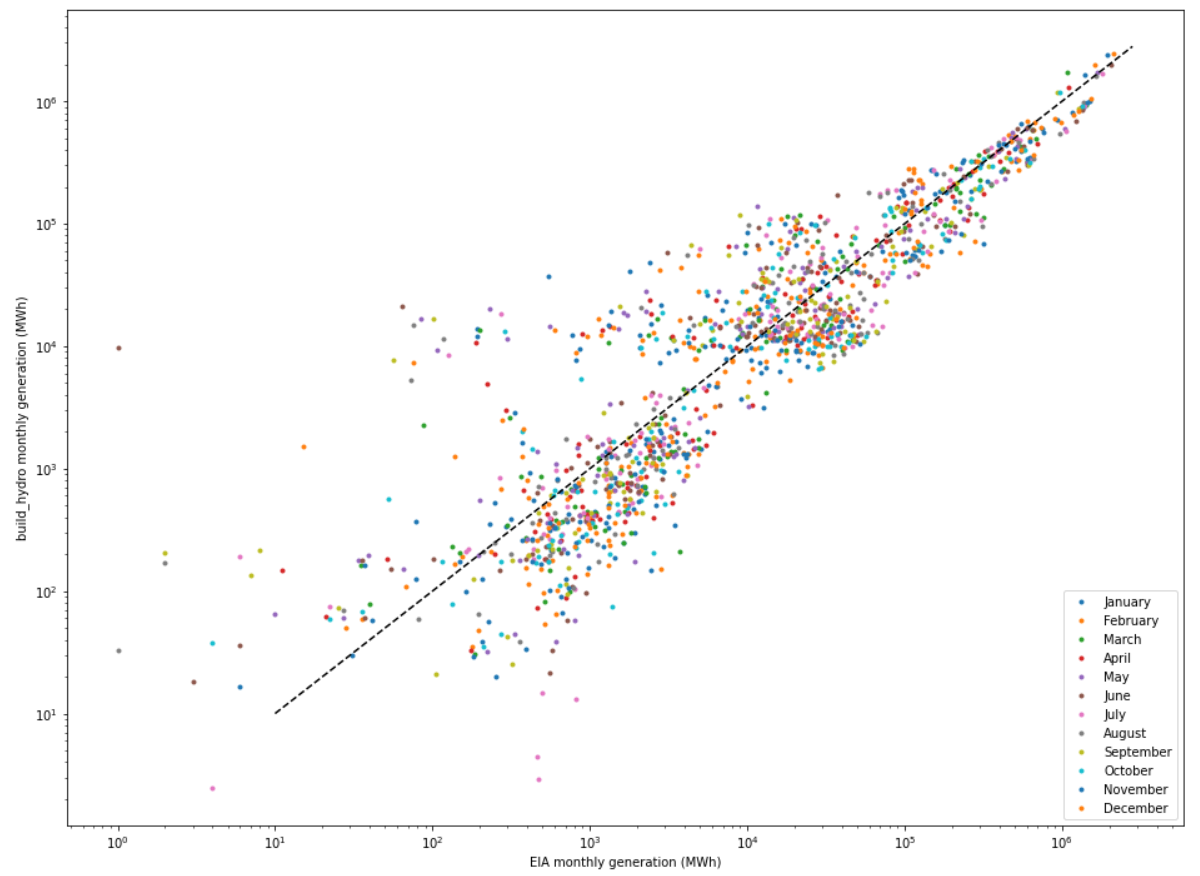
Code to reproduce (with the
hifld
branch of PreREISE checked out):Describe your proposed implementation, if applicable
build_hydro
is refactored to be able to (potentially toggled by a feature flag) scale the hourly BA-level shapes to better represent the monthly energy totals from EIA 923. I believe this information can be retrieved from the EIA's API using the same API key as we use to grab the BA-level hydro generation, or alternatively could be retrieved from an alternate source (see #246). Using a naive scaling factor based on (EIA 923 monthly energy / build_hydro monthly energy) could be problematic since it could result in values which are greater than 1 during some hours. If we use this approach, we'll need to be sure to clip these values to be no larger than 1.EIA Form 923 also seems to sometimes contain distinct entries for conventional hydro (
"Reported Prime Mover" == "HY"
) vs. pumped storage hydro ("Reported Prime Mover" == "PS"
) within the same Plant Code entry, and reports the 'fuel consumed' for pumping. We may be able to use this information to identify which plants are pumped storage (and whose profiles can therefore go negative) vs. which plants are conventional hydro only (and should have their profiles clipped to a minimum of 0).