So i started to investigate how i can possibly using it but i have to conclude i'm stucked.
This is my usage :
I'm creating a list of vertices and indices for creating a Shape for a PhysicsBody
Code
```
Vector3[] verticesData = new Vector3[]
{
// TOP FACE
new Vector3( 64, 64, 64 ),
new Vector3( -64, 64, 64),
new Vector3( -64, -64, 64 ),
new Vector3( 64, -64, 64 ),
// BOTTOM FACE
new Vector3( -64, 64, -64 ),
new Vector3( 64, 64, -64 ),
new Vector3( 64, -64, -64 ),
new Vector3( -64, -64, -64 ),
};
int[] trianglesData = new int[]
{
0,1,2,
0,2,3,
4,5,6,
4,6,7,
5,0,3,
5,3,6,
6,3,2,
6,2,7,
7,2,1,
7,1,4,
4,1,0,
4,0,5,
};
```
When a create a triangle, i store these vertices in a List<TriangleVertices> of my Entity. (TriangleVertices is a custom class with List<Vector3>)
So basically, when i create a Triangle Shape, i have 3 vertices, so 1 TriangleVertices object is stored at the index 0 of my List.
And then i add a second Triangle, which is at the index 1 of my List... etc...
Example of adding 1 triangle manually
```
// Init for creating my final PhysicsShape
List allVerts = new();
List allIndices = new();
// Init for adding 1 triangle vertices
List verts = new();
// First vertex of my triangle
int extraVertexIndex = trianglesData[(0 * 3) + 0];
Vector3 extraPos = verticesData[extraVertexIndex];
// Add my vertex into my List which be contained in my List
verts.Add( extraPos );
// Add my Vertex into my List for creating my final PhysicsShape
allVerts.Add( new SimpleVertex()
{
position = extraPos,
normal = Vector3.Up,
tangent = Vector3.Forward,
texcoord = Vector2.Zero,
} );
// Add my indices int my List for creating my final PhysicsShape
allIndices.Add( 0 * 3 + 0 );
// Second vertex of my triangle
extraVertexIndex = trianglesData[(0 * 3) + 1];
extraPos = verticesData[extraVertexIndex];
verts.Add( extraPos );
allVerts.Add( new SimpleVertex()
{
position = extraPos,
normal = Vector3.Up,
tangent = Vector3.Forward,
texcoord = Vector2.Zero,
} );
allIndices.Add( 0 * 3 + 1 );
// Third vertex of my triangle
extraVertexIndex = trianglesData[(0 * 3) + 2];
extraPos = verticesData[extraVertexIndex];
verts.Add( extraPos );
allVerts.Add( new SimpleVertex()
{
position = extraPos,
normal = Vector3.Up,
tangent = Vector3.Forward,
texcoord = Vector2.Zero,
} );
allIndices.Add( 0 * 3 + 2 );
// Add my first triangle vertices in an custom List<> for my Entity
TriangleVertices.Add( verts );
verts.Clear();
// [...] Repeat the operation for others triangles
```
And finally, i add all Vertices and Indices to my PhysicsBody with: PhysicsBody.AddMeshShape(allVerts, allIndices).
So i have 1 Entity with 1 PhysicsBody with 1 PhysicsShape that stored X triangles
So I use TraceResult.Triangle to retrieve the current Triangle hit. So it will return 0,1,2... etc. and with this index, i can retrieve my list of Vertices stored in my list by List<TriangleVertices>.ElementAt(tr.Triangle);
But something occurs when my Triangle use vertices several times: the shape index starts to be reordered independently of my vertices list order. See my picture below
So List<TriangleVertices>.ElementAt(tr.Triangle) becomes wrong and i don't understand how these triangles are re-ordered:
If someone knows how these indexes are set, i will be able to reorder my List and so be able to retrieve my Shape vertices by TraceResult.Triangle
What should it be?
It could be cool to get an "official" example of Sandbox.TraceResult.Triangle usage
I tried my example cause a PhysicsShape currently didn't store any interesting information. (There is already an issue with adding more information to PhysicsShape: https://github.com/sboxgame/issues/issues/2405)
So i started to implement this logic by thinking that adding vertices will follow the list order but it doesn't (well the engine seems to reorder indexes)
What it is?
Hello i'm searching for a use case of using https://asset.party/api/Sandbox.TraceResult.Triangle. There are currently no public code with an usage example.
So i started to investigate how i can possibly using it but i have to conclude i'm stucked.
This is my usage :
I'm creating a list of vertices and indices for creating a Shape for a PhysicsBody
Code
``` Vector3[] verticesData = new Vector3[] { // TOP FACE new Vector3( 64, 64, 64 ), new Vector3( -64, 64, 64), new Vector3( -64, -64, 64 ), new Vector3( 64, -64, 64 ), // BOTTOM FACE new Vector3( -64, 64, -64 ), new Vector3( 64, 64, -64 ), new Vector3( 64, -64, -64 ), new Vector3( -64, -64, -64 ), }; int[] trianglesData = new int[] { 0,1,2, 0,2,3, 4,5,6, 4,6,7, 5,0,3, 5,3,6, 6,3,2, 6,2,7, 7,2,1, 7,1,4, 4,1,0, 4,0,5, }; ```When a create a triangle, i store these vertices in a
List<TriangleVertices>
of my Entity. (TriangleVertices
is a custom class withList<Vector3>
) So basically, when i create a Triangle Shape, i have 3 vertices, so 1 TriangleVertices object is stored at the index 0 of my List. And then i add a second Triangle, which is at the index 1 of my List... etc...Example of adding 1 triangle manually
``` // Init for creating my final PhysicsShape ListAnd finally, i add all Vertices and Indices to my PhysicsBody with:
PhysicsBody.AddMeshShape(allVerts, allIndices)
. So i have 1 Entity with 1 PhysicsBody with 1 PhysicsShape that stored X trianglesSo I use
TraceResult.Triangle
to retrieve the current Triangle hit. So it will return 0,1,2... etc. and with this index, i can retrieve my list of Vertices stored in my list byList<TriangleVertices>.ElementAt(tr.Triangle);
But something occurs when my Triangle use vertices several times: the shape index starts to be reordered independently of my vertices list order. See my picture below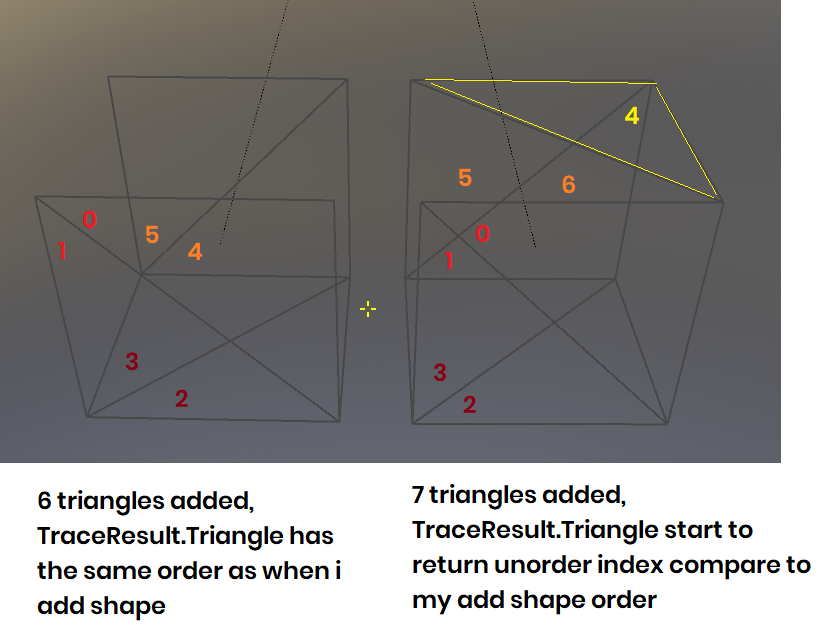
So and so be able to retrieve my Shape vertices by
List<TriangleVertices>.ElementAt(tr.Triangle)
becomes wrong and i don't understand how these triangles are re-ordered: If someone knows how these indexes are set, i will be able to reorder my ListTraceResult.Triangle
What should it be?
It could be cool to get an "official" example of
Sandbox.TraceResult.Triangle
usageI tried my example cause a
PhysicsShape
currently didn't store any interesting information. (There is already an issue with adding more information toPhysicsShape
: https://github.com/sboxgame/issues/issues/2405) So i started to implement this logic by thinking that adding vertices will follow the list order but it doesn't (well the engine seems to reorder indexes)Regards,