Tiktok Downloader & Stalk User
Table of Contents
Description
This project uses the Unofficial API from Tiktok.
- Can be used to download videos, images / slides and music from Tiktok
- Can be used to view someone's profile from Tiktok
- No login or password are required
- It is recommended to use your own cookies on Tiktok Stalker
How to get Tiktok Cookie
You can use cookies in the form of String or JSON
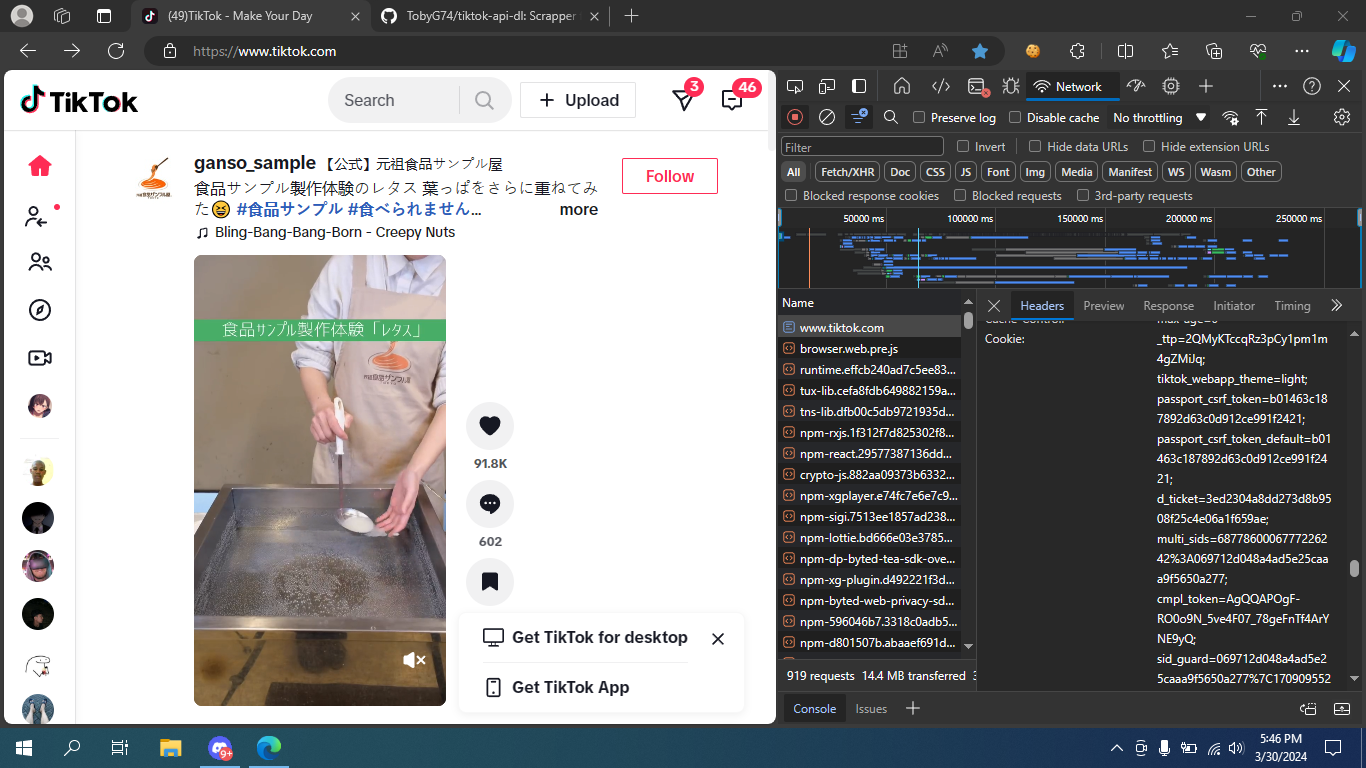
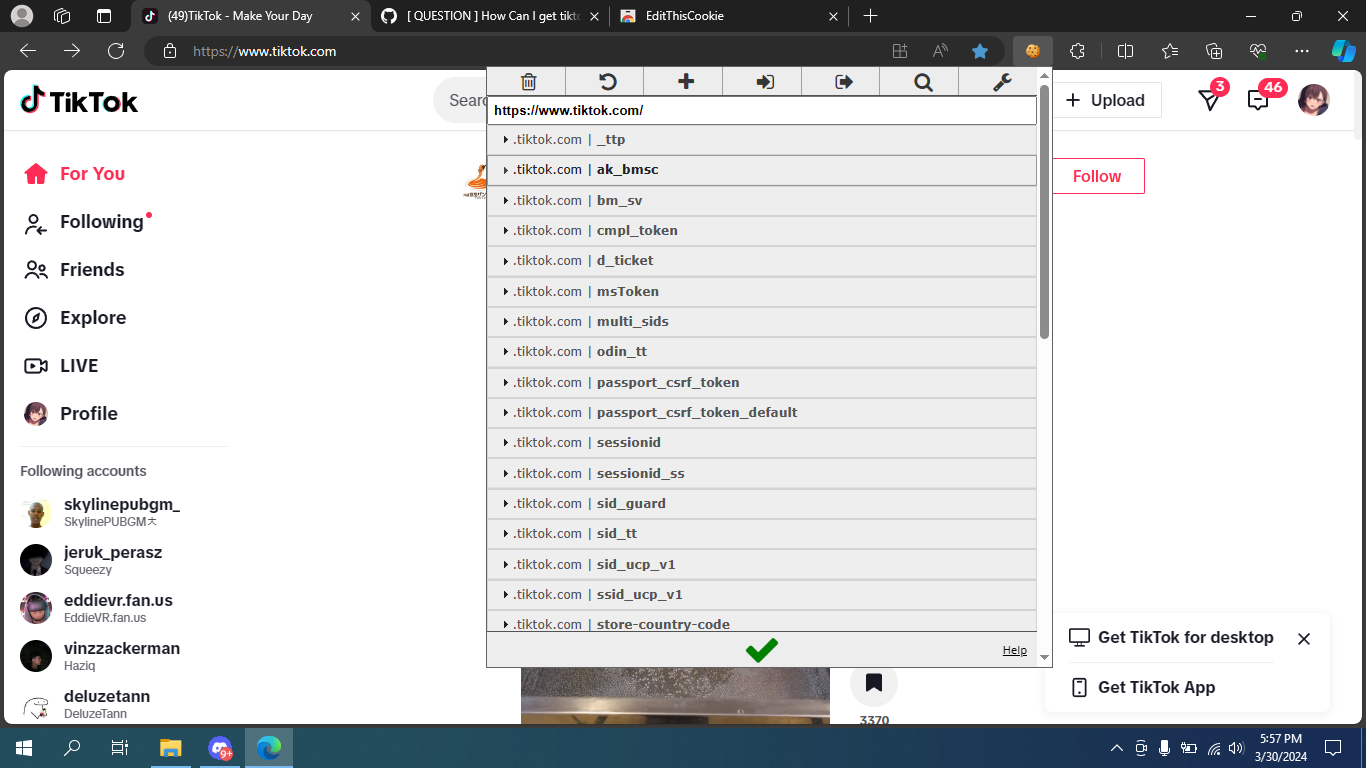
Build this project
- Clone the repository
- Install the dependencies
- Run the build script
git clone https://github.com/TobyG74/tiktok-api-dl.git
cd tiktok-api-dl
npm install
npm run build
Install
- This module requires Node.js v10+ to run.
From NPM
npm install @tobyg74/tiktok-api-dl
From YARN
yarn add @tobyg74/tiktok-api-dl
From Github
npm install github:TobyG74/tiktok-api-dl
Examples
Tiktok Downloader
- V1 uses the API from TiktokAPI
- V2 uses the API from SSSTik
- V3 uses the API from MusicalDown
Options
version
: The version of the downloader you want to use
v1
: TiktokAPI
v2
: SSSTik
v3
: MusicalDown
proxy
: Proxy for request
const Tiktok = require("@tobyg74/tiktok-api-dl")
const tiktok_url = "https://vt.tiktok.com/ZS84BnrU9"
Tiktok.Downloader(tiktok_url, {
version: "v1", // version: "v1" | "v2" | "v3"
proxy: "YOUR_PROXY" // Support Proxy Http, Https, Socks5
}).then((result) => {
console.log(result)
})
Tiktok Search
Note : Cookies are required for searching users or live
Options
type
: The type of search you want to use
user
: Search User
live
: Search Live
page
: The page you want to search
cookie
: Your Tiktok Cookie
proxy
: Proxy for request
Search User | Live
const Tiktok = require("@tobyg74/tiktok-api-dl")
const username = "tobz2k19"
Tiktok.Search(username, {
type: "user" || "live",
page: 1,
cookie: process.env.COOKIE || "Your Cookie",
proxy: "YOUR_PROXY" // Support Proxy Http, Https, Socks5
}).then((result) => {
console.log(result)
})
Tiktok Stalker
Options
cookie
: Only needed if you want to get all user posts
postLimit
: Limit the number of posts to display
proxy
: Proxy for request
const Tiktok = require("@tobyg74/tiktok-api-dl")
const username = "tobz2k19"
Tiktok.StalkUser(username, {
cookie: process.env.COOKIE || "Your Cookie"
postLimit: 10, // Limit the number of posts to display
proxy: "YOUR_PROXY" // Support Proxy Http, Https, Socks5
}).then((result) => {
console.log(result)
})
Response
Tiktok Downloader V1
```ts
{
status: "success" | "error"
message?: string
result?: {
type: "video" | "image"
id: string
createTime: number
description: string
isADS: boolean
hashtag: string[]
author: {
uid: string
username: string
nickname: string
signature: string
region: string
avatarLarger: string
avatarThumb: string
avatarMedium: string
url: string
}
statistics: {
playCount: number
downloadCount: number
shareCount: number
commentCount: number
diggCount: number
collectCount: number
forwardCount: number
whatsappShareCount: number
loseCount: number
loseCommentCount: number
whatsappShareCount: number
repostCount: number
}
video?: {
ratio: string
duration: number
playAddr: string
downloadAddr: string
cover: string
originCover: string
dynamicCover: string
}
images?: string[]
music: {
id: number
title: string
author: string
album: string
playUrl: string[]
coverLarge: string[]
coverMedium: string[]
coverThumb: string[]
duration: number
isCommerceMusic: boolean
isOriginalSound: boolean
isAuthorArtist: boolean
}
}
}
```
Tiktok Downloader V2
```ts
{
status: "success" | "error"
message?: string
result?: {
type: "video" | "image"
description: string
author: {
nickname: string
avatr: string
}
statistics: {
likeCount: string
commentCount: string
shareCount: string
}
video?: string
images?: string[]
music: string
}
}
```
Tiktok Downloader V3
```ts
{
status: "success" | "error"
message?: string
result?: {
type: "video" | "image"
desc?: string
author: {
avatar?: string
nickname: string
}
music?: string
images?: string[]
video1?: string
video2?: string
videoHD?: string
videoWatermark?: string
}
}
```
Tiktok Search "User"
```ts
{
status: "success" | "error"
message?: string
result?: [{
uid: string
username: string
nickname: string
signature: string
followerCount: number
avatarThumb: string[]
isVerified: boolean
secUid: string
url: string
}]
}
```
Tiktok Search "Live"
```ts
{
status: "success" | "error"
message?: string
result?: [{
id: string
title: string
cover: string[]
squareCover: string[]
rectangleCover: string[]
liveTypeThirdParty: boolean
hashtag: string
startTime: number
stats: {
totalUser: number
viewerCount: number
likeCount: number
}
owner: {
id: string
nickname: string
username: string
signature: string
avatarThumb: string[]
avatarMedium: string[]
avatarLarge: string[]
modifyTime: number
stats: {
followingCount: number
followerCount: number
}
isVerified: boolean
}
}]
}
```
Tiktok Stalker
```ts
{
status: "success" | "error"
message?: string
result?: {
users: {
username: string
nickname: string
avatar: string
signature: string
verified: boolean
region: string
}
stats: {
followerCount: number
followingCount: number
heartCount: number
videoCount: number
likeCount: number
}
posts: Posts[]
}
}
```
Contributing
- This repository is open source. We really appreciate it if you want to participate in developing this repository...
- Please read our CONTRIBUTING.md and CODE_OF_CONDUCT.md before contributing.
License
- This project is licensed under the Apache License - see the LICENSE file for details.